Getting Started with Angular
Last Updated :
20 Mar, 2024
Angular is a front-end framework for building dynamic web applications. It allows the MVC (Model-View-Controller) architecture and utilizes TypeScript for building applications. So, Angular is an open-source JavaScript framework written in TypeScript. It is maintained by Google, and its primary purpose is to develop single-page applications. It enables users to create large applications in a maintainable manner.
Prerequisites:
What is Angular?
Angular is a popular open-source Typescript framework created by Google for developing web applications. Front-end developers use frameworks like Angular or React to present and manipulate data efficiently and dynamically. Angular has been actively updated and become more and more efficient with time, especially since the time the core functionality was moved to different modules.
Features of Angular
- It uses components and directives. Components are the directives with a template.
- It is written in Microsoft’s TypeScript language, which is a superset of ECMAScript 6 (ES6).
- Angular is supported by all the popular mobile browsers.
- Properties enclosed in “()” and “[]” are used to bind data between the view and the model.
- It provides support for TypeScript and JavaScript.
- Angular uses @Route Config{(…)} for routing configuration.
- It has a better structure compared to AngularJS, easier to create and maintain for large applications but behind AngularJS in the case of small applications.
- It comes with the Angular CLI tool.
Installing the Angular CLI:
CLI is a command line interface. So, the Angular CLI can be installed either locally or globally. But it is often recommended to install it globally, thus installing Angular CLI globally using npm.
Open a terminal window and enter the following command line:
npm install -g @angular/cli
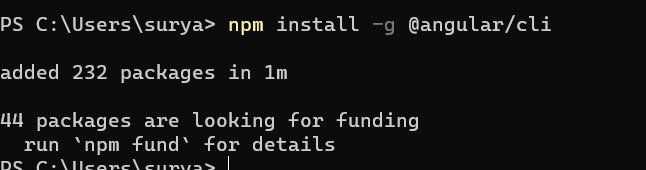
Understanding Angular Basic:
1. Components:
The component is the basic building block of Angular. It has a selector, template, style, and other properties, and it specifies the metadata required to process the component.
Syntax to create components in Angular:
ng generate component my-component
export class Mycomponent{
title = 'Hello,Geeksforgeek!';
2. Moduls:
Modules are a way to group related components and directives, along with the services, pipes, and other codes that they rely on, into a single cohesive unit. Modules provide a way to keep the code organized and make it easier to reuse components and directives across different parts of the application. Modules are defined using the Angular NgModule decorator, which takes an object that specifies the components, directives, and other code that the module contains.
Syntax to create modules in Angular:
ng generate module my-module
3. Services:
The Services is a function or an object that avails or limit to the application in AngularJS, ie., it is used to create variables/data that can be shared and can be used outside the component in which it is defined. Service facilitates built-in service or can make our own service.
Syntax to create services in Angular:
ng generate service my-service
4. Templates and Data Binding:
Templates and data binding play important role in creating dynamic and interactive user interfaces. Templates are HTML files enhanced with Angular-specific syntax, while data binding enables synchronization of data between the component’s model and the view.
Syntax:
<input [(ngModel)]="username">
5. Rounting and Navigation:
Routing enables navigation between different parts of the angular application.learn how to set up rounting in your Angular project using Angular router.
// app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Create a New Angular Project and workspace:
A project is the set of files that comprise an app , a library, or end-to-end tests.
Once Angular CLI is installed, you can create a new Angular project by running the following command:
ng new my-angular-app
The Angular CLI installs the necessary Angular npm packages and other dependecies.
It also creates the following workspacce and start your project files:
- A new workspace, with a root folder named my-angular-ap
- An initial skeleton app project also called my-angular-app (in the src subfolder)
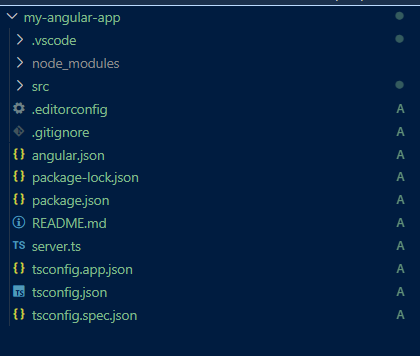
Navigate to the Project preferences:
After the project is create, navigate to the project directory using the ‘cd’ command.
cd my-angular-app
Run the Angular Application:
Once inside your project directory, you can run the Angular application using the following command:
ng serve
This command will compile the application and start a development server.By defauts,the application will be accessible at ‘http://localhost:4200/’ in your web browser.
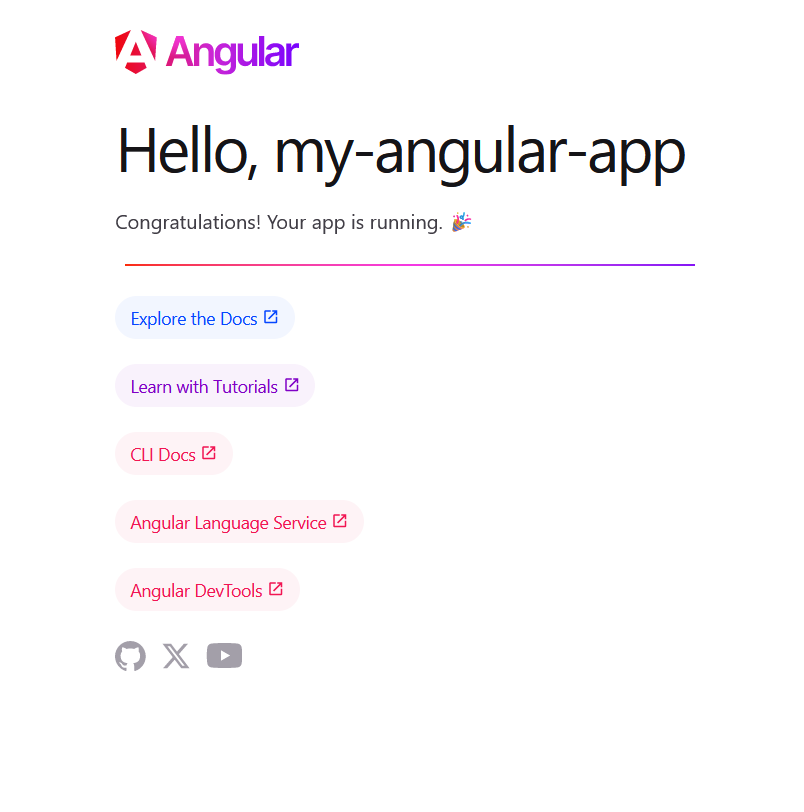
Example of a Basic Angular Application:
Creating a simple Angular component involves defining a TypeScript class with a @Component decorator. We are going to edit this component,thus open src folder-app-app.components.ts and change the title.
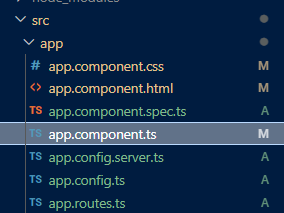
Modify the given files to see the results:
HTML
<!-- app.component.html -->
<main class="main">
<div class="content">
<div class="left-side">
<h1>Hello, {{ title }}</h1>
<p>Congratulations! Your app is running. 🎉</p>
</div>
<div class="divider" role="separator" aria-label="Divider"></div>
<div class="right-side">
</div>
</div>
</main>
<router-outlet />
CSS
/* app.component.css */
content_copyh1 {
color: #369;
font-family: Arial, Helvetica, sans-serif;
font-size: 250%;
}
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import App from '../client/src/App';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
title = 'GeeksForGeek first initiat project,welcome to Angular';
}
Output:
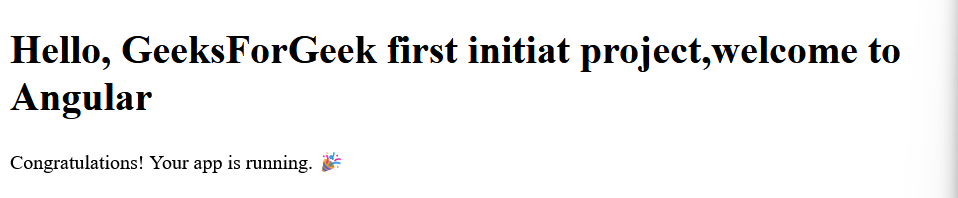
Share your thoughts in the comments
Please Login to comment...