What are the 3 core concepts of React Redux ?
Last Updated :
18 Mar, 2024
Redux is a widely-used state management library that helps in managing the state in our projects. However, it comes with its own terminologies and jargon that can be confusing for beginners. Essentially, Redux comprises of three core concepts: actions, reducers, and store.
In this article, we will cover these concepts in detail and provide an example of how they can be used. By understanding these core concepts, you will be able to work with Redux more efficiently and effectively.
Working of Redux
- Redux operates by maintaining a centralized state tree, offering a method to manage the application state and address state changes.
- Actions are dispatched to the store, initiating reducers to define how the state should change.
- Reducers, being pure functions, take the previous state and an action as input and produce the new state as output.
- Components can subscribe to the store to access and update the state, ensuring a predictable uni-directional data flow throughout the application.
We will discuss about the following core concepts of Redux in this article.
Actions
Actions are nothing but a simple object of javascript, they contain the information that tells what kind of actions to perform and the payload which contains the data required to perform the action.
Syntax of Actions:
{
type: 'ACTION_TYPE',
payload: { /* data required for the action */ }
}
Functions that create these actions are known as Action Creators. These functions returns the action as an object.
function actionCreator(data) {
return {
type: 'ACTION_TYPE',
payload: data
}
}
Reducers
Reducers are pure functions of javascript that take current state and action and returns the new state. They create a new state based on the action type with the required modification and return that new state which then results in updation of the state.
Syntax of Reducers:
const reducerFunction = (state, action) => {
switch(action.type)
{
case 'ACTION_TYPE_1':
return {...state, ...action.payload};
case 'ACTION_TYPE_2':
return {...state, ...action.payload};
default:
return state;
}
}
Store
A store is a place where we store all the data, it is a single source, centralized store from where any component can update and get state.
- createStore(): To initialize store, usecreateStore() method which takes object of reducers.
- dispatch(action): To update the state, we need to dispatch an action which then triggers the reducer function to update the state.
- getState(): To get the state from the store, getState() method is used. It returns the current state of the store.
Syntax of Store:
// createStore()
const store = createStore(INITIAL_STATE);
// dispatch(action)
store.dispatch(actionCreator(data));
// getState()
const current_state = store.getState();
Steps to Create React Application And Installing Redux:
Step 1: Create a React application and Navigate to the project directory the following command:
npx create-react-app my-redux-project --template react
cd my-redux-project
Step 2: Install Redux and React-Redux packages
npm install redux react-redux
Project Structure:
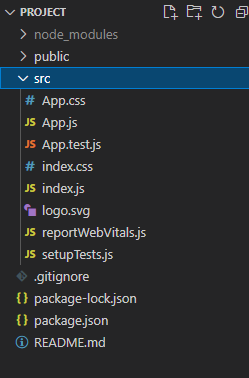
Project Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
"redux": "^5.0.1"
}
Example: Implementation to showcase concept of react redux.
Javascript
// store.js
import { createStore } from 'redux';
// Define the initial state
const initialState = {
todos: []
};
// Define the reducer function
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'ADD_TODO':
return {
...state,
todos: [...state.todos, action.payload]
};
case 'REMOVE_TODO':
return {
...state,
todos: state.todos.filter(todo => todo !== action.payload)
};
default:
return state;
}
};
// Create the Redux store
const store = createStore(reducer);
// Define the action creators
export const addTodo = (todo) => {
return {
type: 'ADD_TODO',
payload: todo
};
};
export const removeTodo = (todo) => {
return {
type: 'REMOVE_TODO',
payload: todo
};
};
console.log(store.getState().todos); // todos - []
store.dispatch(addTodo('Learn about actions'));
store.dispatch(addTodo('Learn about reducers'));
store.dispatch(addTodo('Learn about stores'));
console.log(store.getState().todos); // todos - [ 3 todos ]
store.dispatch(removeTodo('Learn about actions'));
store.dispatch(removeTodo('Learn about reducers'));
console.log(store.getState().todos); // todos - [ 1 todo ]
export default store;
Javascript
// index.js
import React from 'react';
import { createRoot } from 'react-dom/client';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
createRoot(document.getElementById('root')).render(
<Provider store={store}>
<App />
</Provider>
);
Output:

Output
Share your thoughts in the comments
Please Login to comment...