What are middlewares in React Redux ?
Last Updated :
15 Mar, 2024
In the world of creating websites and apps, React and Redux are powerful tools used to build apps that can grow and be easily updated. React helps build user interfaces, while Redux helps keep track of the app’s data in a way that makes it easy to understand. Redux uses something called “middlewares” to control how actions, that change the data, are handled.
In this article, we’ll learn what middleware is and how it makes it easier to manage data in React Redux applications.
What are Middlewares in React Redux?
Middleware in Redux is like a gatekeeper that steps in between when an action is triggered and before it gets processed by the reducer. It gives developers the chance to do extra things with the action before it reaches the reducer, like running extra checks, doing stuff in the background, or even triggering more actions based on the initial one.
Redux Middleware allows you to intercept every action sent to the reducer so you can make changes to the action or cancel the action.
Middleware helps you with logging, error reporting, making asynchronous requests, and a whole lot more.
Common Use Cases of Middlewares:
- Logging: Middleware is frequently leveraged to record actions and their subsequent state alterations. Developers can establish middleware functions to document details such as action type, payload, and modified state, facilitating debugging and comprehending app dynamics.
- Asynchronous Operations: Despite Redux’s inherently synchronous nature, middleware empowers the management of asynchronous activities, including API requests. Middleware frameworks like Redux Thunk or Redux Saga simplify the distribution of asynchronous actions, enabling developers to retrieve data from servers or trigger side effects before distributing future actions.
- Authentication and Authorization: Middleware can monitor actions associated with authentication and authorization processes. For instance, middleware can verify user authentication prior to specific actions.
- Caching: Middleware allows for the implementation of caching, where frequently accessed data is stored for faster retrieval. The middleware intercepts data retrieval actions, checks if the data is already cached, and avoids making network requests for cached data. This technique enhances performance by reducing the need for repetitive data retrieval.
Approach to implement Middleware in React Redux:
- In reducers.js, we define the initial state and the reducer function for our Redux store. This reducer manages the state of a simple counter.
- In middleware/logger.js, we define a middleware function that logs the dispatched action and the new state.
- store: Represents the Redux store.
- next: A function that represents the next middleware in the chain. It allows the middleware to pass the action along to the next middleware or the reducer.
- action: The action object being dispatched.
- Inside the middleware function, we log the dispatched action and the current state of the Redux store. Then, we called next(action) to pass the action along to the next middleware.
- In store/index.js, we create the Redux store, apply the middleware, and export the configured store.
- In App.js, we create a simple React component that displays the counter value and provides buttons to increment and decrement the counter. We use React Redux hooks (useSelector and useDispatch) to interact with the Redux store.
- Now, whenever an action is dispatched, our custom middleware logs the action and the Redux store’s current state to the console. This aids in debugging and tracing action flows within the app.
- In index.js, we render the App component wrapped in a Provider from React Redux. This provides the Redux store to all components in the application.
Steps to Create a Middleware in React:
Step 1: To initialize the project type the below command in your terminal.
npx create-react-app middleware-react-redux
Step 2: Naviagte to the root directory of your application.
cd middleware-react-redux
Step 3: Install the required packages in your application using the following command.
npm install redux react-redux @reduxjs/toolkit
To create a middleware, we first need to import the applyMiddleware function from Redux like this:
import { applyMiddleware } from "redux";
Example: Let’s say we want to create a middleware that logs every action that is dispatched along with the current state of the Redux store.
Javascript
// store/reducers.js
const initialState = {
count: 0
};
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
...state,
count: state.count + 1
};
case 'DECREMENT':
return {
...state,
count: state.count - 1
};
default:
return state;
}
};
export default rootReducer;
JavaScript
// store/middleware/logger.js
const logger = store => next => action => {
console.log('Dispatching action:', action);
const result = next(action);
console.log('New state:', store.getState());
return result;
};
export default logger;
JavaScript
// store/index.js
import { createStore, applyMiddleware } from 'redux';
import rootReducer from './reducers';
import logger from './middleware/logger';
const store = createStore(
rootReducer,
applyMiddleware(logger)
);
export default store;
JavaScript
// App.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
function App() {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
const increment = () => {
dispatch({ type: 'INCREMENT' });
};
const decrement = () => {
dispatch({ type: 'DECREMENT' });
};
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default App;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Type the following command to run:
npm start
Output:
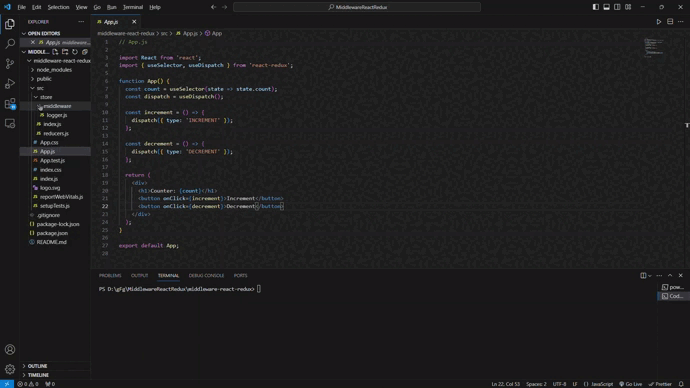
Middlewares in React Redux
Conclusion:
In React Redux apps, middlewares act as gatekeepers for actions. They can control what happens to actions, changing or adding to how they work. With middlewares, you can log actions, handle asynchronous tasks, and add authentication and authorization rules. By using middlewares effectively, you can make your React Redux apps stronger, easier to scale, and simpler to maintain.
Share your thoughts in the comments
Please Login to comment...