Public, Private, and Protected Scope in JavaScript
Last Updated :
20 Nov, 2023
In this article, we will see the fundamentals of public, private, and protected scopes in JavaScript. We’ll explore how to create these scopes, their practical applications, and the conventions used to simulate access control.
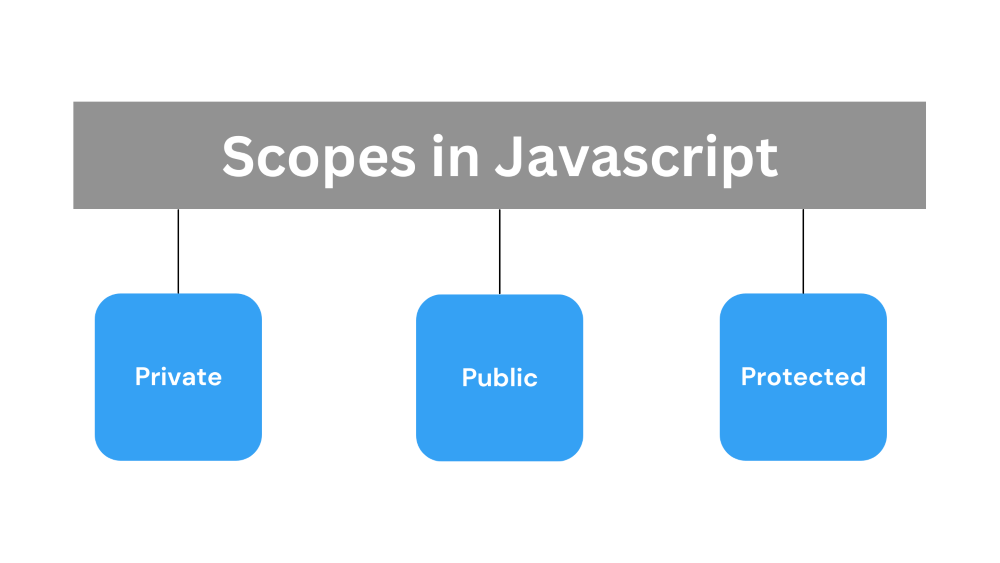
Scopes
Private Scope:
- Private scope in JavaScript is the private members of a class or object that can only be accessed from within the class or object itself.
- To create a private variable or function, you can create using the # prefix. or using closures.
- Private scope can be useful for encapsulating data and behavior within a class or object. This can help to make your code more modular and reusable. It can also help to prevent errors by preventing consumers of your code from accidentally accessing or modifying private members.
When to use Private Scope?
Use private scope for variables and functions that should only be accessible from within the class or object in which they are defined. For example, you might use private scope for the internal state of a class or object, or for implementation details of a method or function.
Example:
Javascript
let Employee = ( function () {
let empName = '' ;
return class {
constructor(name) {
empName = name;
}
getPrivateName() {
return empName;
}
}
})();
const employee = new Employee( 'Aryan' );
console.log(employee.getPrivateName());
console.log(employee.privateName);
|
Output:
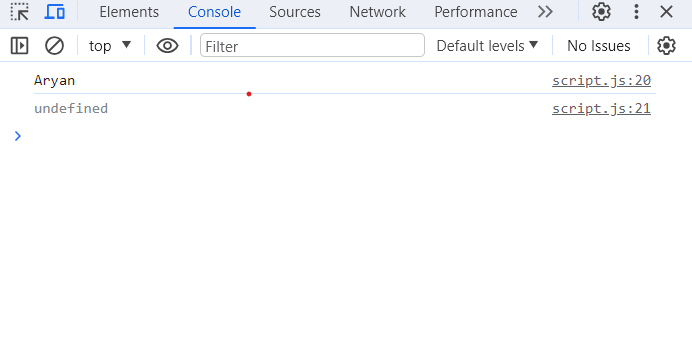
private
Public Scope:
- The Variables and methods declared directly on a class or outside any block are considered public in JavaScript.
- By default, everything declared in JavaScript is public. There is no concept of private/protected by default.
- Public scope can be useful for variables and functions that need to be used by multiple parts of your code. For example, you might use public scope for global variables or functions that are used by all pages of your website.
When to use Public Scope?
Use public scope for variables and functions that need to be accessible from anywhere in your code. For example, you might use public scope for global variables or functions that are used by multiple parts of your code.
Example:
Javascript
let empName = 'Aryan' ;
class Employee {
constructor() {
this .getName = function () {
return empName;
}
}
}
const employee = new Employee();
console.log(employee.getName());
|
Output:
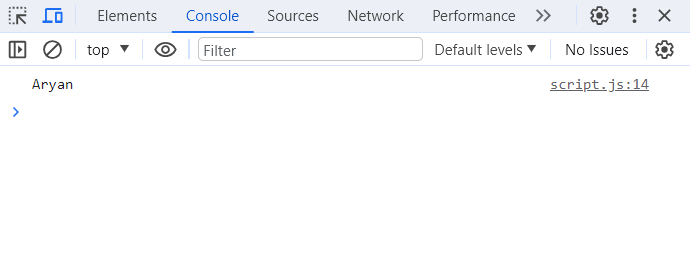
public
Protected Scope:
- Protected members are accessible only within the class itself and its subclasses. Protected methods can access properties by getting them from the WeakMap.
- Protected scope can be useful for variables and functions that need to be accessible from within the class in which they are defined, and from any classes that inherit from that class. For example, you might use protected scope for variables and functions that are shared by all subclasses of a particular class.
When to use Protected Scope?
Use protected scope for variables and functions that need to be accessible from within the class in which they are defined, and from any classes that inherit from that class. For example, you might use protected scope for variables and functions that are shared by all subclasses of a particular class.
Example:
Javascript
const protectedMap = new WeakMap();
class Person {
constructor(name) {
protectedMap.set( this , {
name
});
}
getName() {
return protectedMap.get( this ).name;
}
}
class Employee extends Person {
constructor(name, title) {
super (name);
protectedMap.get( this ).title = title;
}
getTitle() {
return protectedMap.get( this ).title;
}
}
const emp = new Employee( 'Aryan' , 'Manager' );
console.log(emp.getName());
console.log(emp.getTitle());
console.log(emp.name);
|
Output:
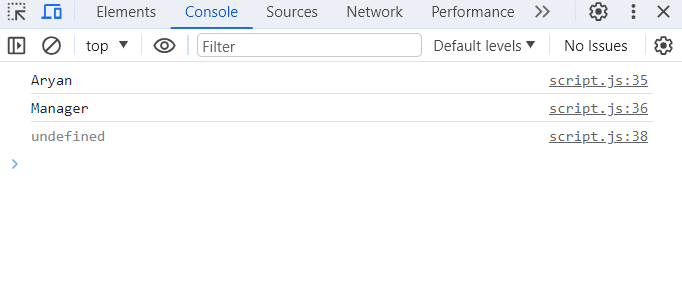
protected
Share your thoughts in the comments
Please Login to comment...