Types of Operators in Programming
Last Updated :
23 Apr, 2024
Types of operators in programming are symbols or keywords that represent computations or actions performed on operands. Operands can be variables, constants, or values, and the combination of operators and operands form expressions. Operators play a crucial role in performing various tasks, such as arithmetic calculations, logical comparisons, bitwise operations, etc.
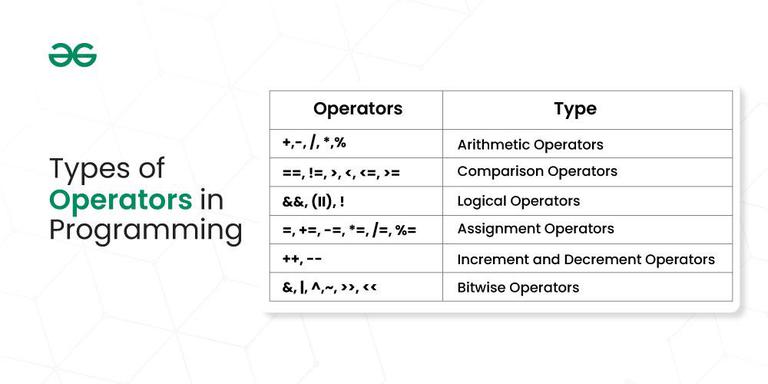
Types of Operators in Programming
Types of Operators in Programming:
Here are some common types of operators:
- Arithmetic Operators: Perform basic arithmetic operations on numeric values.
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Modulus (
%
)
- Assignment Operators
- Basic Assignment (
=
) - Add and Assign (
+=
) - Subtract and Assign (
-=
) - Multiply and Assign (
*=
) - Divide and Assign (
/=
) - Modulus and Assign (
%=
)
- Unary Operators
- Increment (
++
) - Decrement (
--
) - Unary Plus (
+
) - Unary Minus (
-
) - Logical NOT (
!
)
- Comparison Operators
- Equal to (
==
) - Not Equal to (
!=
) - Less Than (
<
) - Greater Than (
>
) - Less Than or Equal To (
<=
) - Greater Than or Equal To (
>=
)
- Logical Operators
- Logical AND (
&&
) - Logical OR (
||
) - Logical NOT (
!
)
- Bitwise Operators
- Bitwise AND (
&
) - Bitwise OR (
|
) - Bitwise XOR (
^
) - Bitwise NOT (
~
) - Left Shift (
<<
) - Right Shift (
>>
)
- Conditional Operator
These operators provide the building blocks for creating complex expressions and performing diverse operations in programming languages. Understanding their usage is crucial for writing efficient and expressive code.
Arithmetic Operators in Programming:
Arithmetic operators in programming are fundamental components of programming languages, enabling the manipulation of numeric values for various computational tasks. Here’s an elaboration on the key arithmetic operators:
Operator | Description | Examples |
---|
+ (Addition) | Combines two numeric values, yielding their sum. | result = 5 + 3; (result will be 8) |
– (Subtraction) | Subtracts the right operand from the left operand. | difference = 10 - 4; (difference will be 6) |
* (Multiplication) | Multiplies two numeric values, producing their product. | product = 3 * 7; (product will be 21) |
/ (Division) | Divides the left operand by the right operand, producing a quotient. | quotient = 15 / 3; (quotient will be 5) |
% (Modulo) | Returns the remainder after the division of the left operand by the right operand. | remainder = 10 % 3; (remainder will be 1) |
These operators are foundational for mathematical calculations, financial computations, and various algorithmic implementations. They are commonly used in everyday programming scenarios, providing the tools necessary for handling numerical data and solving mathematical problems within a program. Understanding how to use arithmetic operators is essential for performing precise and efficient calculations in programming.
C++
#include <iostream>
using namespace std;
int main()
{
int a = 10, b = 5;
cout << a << " + " << b << " = " << a + b << endl;
cout << a << " - " << b << " = " << a - b << endl;
cout << a << " * " << b << " = " << a * b << endl;
cout << a << " / " << b << " = " << a / b << endl;
cout << a << " % " << b << " = " << a % b << endl;
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
int a = 10, b = 5;
System.out.println(a + " + " + b + " = " + (a + b));
System.out.println(a + " - " + b + " = " + (a - b));
System.out.println(a + " * " + b + " = " + (a * b));
System.out.println(a + " / " + b + " = " + (a / b));
System.out.println(a + " % " + b + " = " + (a % b));
}
}
Python3
a = 10
b = 5
print(f"{a} + {b} = {a + b}")
print(f"{a} - {b} = {a - b}")
print(f"{a} * {b} = {a * b}")
print(f"{a} / {b} = {a / b}")
print(f"{a} % {b} = {a % b}")
Javascript
// Main function
function main() {
// Declare and initialize variables
let a = 10, b = 5;
// Output addition operation
console.log(a + " + " + b + " = " + (a + b));
// Output subtraction operation
console.log(a + " - " + b + " = " + (a - b));
// Output multiplication operation
console.log(a + " * " + b + " = " + (a * b));
// Output division operation
console.log(a + " / " + b + " = " + (a / b));
// Output modulus operation
console.log(a + " % " + b + " = " + (a % b));
}
// Call the main function to execute the program
main();
Output10 + 5 = 15
10 - 5 = 5
10 * 5 = 50
10 / 5 = 2
10 % 5 = 0
Comparison Operators in Programming:
Comparison operators in programming are used to compare two values or expressions and return a Boolean result indicating the relationship between them. These operators play a crucial role in decision-making and conditional statements. Here are the common comparison operators:
Operator | Description | Examples |
---|
== (Equal to) | Checks if the values on both sides are equal. | 5 == 5; (evaluates to true) |
!= (Not equal to) | Checks if the values on both sides are not equal. | 10 != 5; (evaluates to true) |
< (Less than) | Tests if the value on the left is less than the value on the right. | 3 < 7; (evaluates to true) |
> (Greater than) | Tests if the value on the left is greater than the value on the right. | 10 > 8; (evaluates to true) |
<= (Less than or equal to) | Checks if the value on the left is less than or equal to the value on the right. | 5 <= 5; (evaluates to true) |
>= (Greater than or equal to) | Checks if the value on the left is greater than or equal to the value on the right. | 8 >= 8; (evaluates to true) |
These operators are extensively used in conditional statements, loops, and decision-making constructs to control the flow of a program based on the relationship between variables or values. Understanding comparison operators is crucial for creating logical and effective algorithms in programming.
C++
#include <iostream>
using namespace std;
int main()
{
int a = 5, b = 5, c = 10;
if (a == b)
cout << a << " is equal to " << b << endl;
if (a != c)
cout << a << " is not equal to " << c << endl;
if (a < c)
cout << a << " is less than " << c << endl;
if (a <= b)
cout << a << " is less than or equal to " << b << endl;
if (c > a)
cout << c << " is greater than " << a << endl;
if (a >= b)
cout << a << " is greater than or equal to " << b << endl;
return 0;
}
Java
/* package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
int a = 5, b = 5, c = 10;
if (a == b)
System.out.println(a + " is equal to " + b);
if (a != c)
System.out.println(a + " is not equal to " + c);
if (a < c)
System.out.println(a + " is less than " + c);
if (a <= b)
System.out.println(a + " is less than or equal to " + b);
if (c > a)
System.out.println(c + " is greater than " + a);
if (a >= b)
System.out.println(a + " is greater than or equal to " + b);
}
}
Python3
a = 5
b = 5
c = 10
if a == b:
print(f"{a} is equal to {b}")
if a != c:
print(f"{a} is not equal to {c}")
if a < c:
print(f"{a} is less than {c}")
if a <= b:
print(f"{a} is less than or equal to {b}")
if c > a:
print(f"{c} is greater than {a}")
if a >= b:
print(f"{a} is greater than or equal to {b}")
JavaScript
function main() {
let a = 5, b = 5, c = 10;
if (a === b)
console.log(a + " is equal to " + b);
if (a !== c)
console.log(a + " is not equal to " + c);
if (a < c)
console.log(a + " is less than " + c);
if (a <= b)
console.log(a + " is less than or equal to " + b);
if (c > a)
console.log(c + " is greater than " + a);
if (a >= b)
console.log(a + " is greater than or equal to " + b);
}
main();
Output5 is equal to 5
5 is not equal to 10
5 is less than 10
5 is less than or equal to 5
10 is greater than 5
5 is greater than or equal to 5
Logical Operators in Programming:
Logical operators in programming are used to perform logical operations on Boolean values. These operators are crucial for combining or manipulating conditions and controlling the flow of a program based on logical expressions. Here are the common logical operators:
Operator | Description | Examples |
---|
&& (Logical AND) | Returns true if both operands are true; otherwise, it returns false. | true && false; (evaluates to false) |
(||) Logical OR | Returns true if at least one of the operands is true; otherwise, it returns false | true || false; (evaluates to true)
|
! (Logical NOT) | Returns true if the operand is false and vice versa; it negates the Boolean value. | !true; (evaluates to false) |
These logical operators are frequently used in conditional statements (if, else if, else), loops, and decision-making constructs to create complex conditions based on multiple Boolean expressions. Understanding how to use logical operators is essential for designing effective and readable control flow in programming.
C++
#include <iostream>
using namespace std;
int main()
{
// Logical AND (&&)
int x = 5, y = 10;
if (x > 0 && y > 0) {
cout << "Both " << x << " and " << y
<< " are greater than 0." << endl;
}
// Logical OR (||)
x = 15;
y = -5;
if (x > 0 || y > 0) {
cout << "At least one of " << x << " or " << y
<< " is greater than 0." << endl;
}
// Logical NOT (!)
if (!(x == y)) {
cout << x << " is not equal to " << y << endl;
}
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
// Logical AND (&&)
int x = 5, y = 10;
if (x > 0 && y > 0) {
System.out.println("Both " + x + " and " + y
+ " are greater than 0.");
}
// Logical OR (||)
x = 15;
y = -5;
if (x > 0 || y > 0) {
System.out.println("At least one of " + x
+ " or " + y
+ " is greater than 0.");
}
// Logical NOT (!)
if (!(x == y)) {
System.out.println(x + " is not equal to " + y);
}
}
}
Python3
# Logical AND (and)
x, y = 5, 10
if x > 0 and y > 0:
print(f"Both {x} and {y} are greater than 0.")
# Logical OR (or)
x, y = 15, -5
if x > 0 or y > 0:
print(f"At least one of {x} or {y} is greater than 0.")
# Logical NOT (not)
if not (x == y):
print(f"{x} is not equal to {y}")
JavaScript
// Logical AND (&&)
let x = 5, y = 10;
if (x > 0 && y > 0) {
console.log(`Both ${x} and ${y} are greater than 0.`);
}
// Logical OR (||)
x = 15;
y = -5;
if (x > 0 || y > 0) {
console.log(`At least one of ${x} or ${y} is greater than 0.`);
}
// Logical NOT (!)
if (!(x === y)) {
console.log(`${x} is not equal to ${y}`);
}
OutputBoth 5 and 10 are greater than 0.
At least one of 15 or -5 is greater than 0.
15 is not equal to -5
Assignment Operators in Programming:
Assignment operators in programming are used to assign values to variables. They are essential for storing and updating data within a program. Here are common assignment operators:
Operator | Description | Examples |
---|
= (Assignment) | Assigns the value on the right to the variable on the left. | x = 10; assigns the value 10 to the variable x. |
+= (Addition Assignment) | Adds the value on the right to the current value of the variable on the left and assigns the result to the variable. | x += 5; is equivalent to x = x + 5; |
-= (Subtraction Assignment) | Subtracts the value on the right from the current value of the variable on the left and assigns the result to the variable. | y -= 3; is equivalent to y = y - 3; |
*= (Multiplication Assignment) | Multiplies the current value of the variable on the left by the value on the right and assigns the result to the variable. | z *= 2; is equivalent to z = z * 2; |
/= (Division Assignment) | Divides the current value of the variable on the left by the value on the right and assigns the result to the variable. | a /= 4; is equivalent to a = a / 4; |
%= (Modulo Assignment) | Calculates the modulo of the current value of the variable on the left and the value on the right, then assigns the result to the variable. | b %= 3; is equivalent to b = b % 3; |
Assignment operators are fundamental for updating variable values, especially in loops and mathematical computations, contributing to the dynamic nature of programming. Understanding how to use assignment operators is essential for effective variable manipulation in a program.
C++
#include <iostream>
using namespace std;
int main()
{
// Assignment (=)
int x;
x = 10;
cout << "x after assignment: " << x << endl;
// Addition Assignment (+=)
x += 3;
cout << "x after x += 3: " << x << endl;
// Subtraction Assignment (-=)
x -= 2;
cout << "x after x -= 2: " << x << endl;
// Multiplication Assignment (*=)
x *= 4;
cout << "x after x *= 4: " << x << endl;
// Division Assignment (/=)
x /= 2;
cout << "x after x /= 2: " << x << endl;
// Modulo Assignment (%=)
x %= 3;
cout << "x after x %= 3: " << x << endl;
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
// Assignment (=)
int x;
x = 10;
System.out.println("x after assignment: " + x);
// Addition Assignment (+=)
x += 3;
System.out.println("x after x += 3: " + x);
// Subtraction Assignment (-=)
x -= 2;
System.out.println("x after x -= 2: " + x);
// Multiplication Assignment (*=)
x *= 4;
System.out.println("x after x *= 4: " + x);
// Division Assignment (/=)
x /= 2;
System.out.println("x after x /= 2: " + x);
// Modulo Assignment (%=)
x %= 3;
System.out.println("x after x %= 3: " + x);
}
}
Python3
# Assignment (=)
x = 10
print("x after assignment:", x)
# Addition Assignment (+=)
x += 3
print("x after x += 3:", x)
# Subtraction Assignment (-=)
x -= 2
print("x after x -= 2:", x)
# Multiplication Assignment (*=)
x *= 4
print("x after x *= 4:", x)
# Division Assignment (/=)
x /= 2
print("x after x /= 2:", x)
# Modulo Assignment (%=)
x %= 3
print("x after x %= 3:", x)
JavaScript
// Assignment (=)
let x;
x = 10;
console.log("x after assignment: " + x);
// Addition Assignment (+=)
x += 3;
console.log("x after x += 3: " + x);
// Subtraction Assignment (-=)
x -= 2;
console.log("x after x -= 2: " + x);
// Multiplication Assignment (*=)
x *= 4;
console.log("x after x *= 4: " + x);
// Division Assignment (/=)
x /= 2;
console.log("x after x /= 2: " + x);
// Modulo Assignment (%=)
x %= 3;
console.log("x after x %= 3: " + x);
Outputx after assignment: 10
x after x += 3: 13
x after x -= 2: 11
x after x *= 4: 44
x after x /= 2: 22
x after x %= 3: 1
Increment and Decrement Operators in Programming:
Increment and decrement operators in programming are used to increase or decrease the value of a variable by 1, respectively. They are shorthand notations for common operations and are particularly useful in loops. Here are the two types:
Operator | Description | Examples |
---|
++ (Increment) | Increases the value of a variable by 1. | x++; is equivalent to x = x + 1; or x += 1; |
— (Decrement) | Decreases the value of a variable by 1. | y--; is equivalent to y = y - 1; or y -= 1; |
These operators are frequently employed in loops, especially for iterating through arrays or performing repetitive tasks. Their concise syntax enhances code readability and expressiveness.
C++
#include <iostream>
using namespace std;
int main()
{
// Increment Operator (++)
int a = 5;
cout << "Value of a: " << a << endl;
a++;
cout << "Value of a after (a++): " << a << endl;
// Decrement Operator (--)
int b = 8;
cout << "Value of b: " << b << endl;
b--;
cout << "Value of b after (b--): " << b << endl;
// Increment Operator in Expression
int x = 10;
int y = x++;
cout << "Value of x: " << x << endl;
cout << "Value of y (post-increment): " << y << endl;
// Decrement Operator in Expression
int m = 15;
int n = --m;
cout << "Value of m: " << m << endl;
cout << "Value of n (pre-decrement): " << n << endl;
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static void main(String[] args)
{
// Increment Operator (++)
int a = 5;
System.out.println("Value of a: " + a);
a++;
System.out.println("Value of a after (a++): " + a);
// Decrement Operator (--)
int b = 8;
System.out.println("Value of b: " + b);
b--;
System.out.println("Value of b after (b--): " + b);
// Increment Operator in Expression
int x = 10;
int y = x++;
System.out.println("Value of x: " + x);
System.out.println("Value of y (post-increment): " + y);
// Decrement Operator in Expression
int m = 15;
int n = --m;
System.out.println("Value of m: " + m);
System.out.println("Value of n (pre-decrement): " + n);
}
}
Python3
# code in python
# Increment Operator (++)
a = 5
print("Value of a:", a)
a += 1
print("Value of a after (a++):", a)
# Decrement Operator (--)
b = 8
print("Value of b:", b)
b -= 1
print("Value of b after (b--):", b)
# Increment Operator in Expression
x = 10
y = x
x += 1
print("Value of x:", x)
print("Value of y (post-increment):", y)
# Decrement Operator in Expression
m = 15
n = m
m -= 1
print("Value of m:", m)
print("Value of n (pre-decrement):", n)
#this code is contributed by Sundaram
JavaScript
// Increment Operator (++)
let a = 5;
console.log("Value of a: " + a);
a++;
console.log("Value of a after (a++): " + a);
// Decrement Operator (--)
let b = 8;
console.log("Value of b: " + b);
b--;
console.log("Value of b after (b--): " + b);
// Increment Operator in Expression
let x = 10;
let y = x++;
console.log("Value of x: " + x);
console.log("Value of y (post-increment): " + y);
// Decrement Operator in Expression
let m = 15;
let n = --m;
console.log("Value of m: " + m);
console.log("Value of n (pre-decrement): " + n);
OutputValue of a: 5
Value of a after (a++): 6
Value of b: 8
Value of b after (b--): 7
Value of x: 11
Value of y (post-increment): 10
Value of m: 14
Value of n (pre-decrement): 14
Bitwise Operators in Programming:
Bitwise operators in programming perform operations at the bit level, manipulating individual bits of binary representations of numbers. These operators are often used in low-level programming, such as embedded systems and device drivers. Here are the common bitwise operators:
Operator | Description | Examples |
---|
& (Bitwise AND) | Performs a bitwise AND operation between corresponding bits of two operands. | A & B sets each bit to 1 if both corresponding bits in A and B are 1. |
| (Bitwise OR) | Performs a bitwise OR operation between corresponding bits of two operands. | A | B sets each bit to 1 if at least one corresponding bit in A or B is 1. |
^ (Bitwise XOR) | Performs a bitwise XOR (exclusive OR) operation between corresponding bits of two operands. | A ^ B sets each bit to 1 if the corresponding bits in A and B are different. |
~ (Bitwise NOT) | Inverts the bits of a single operand, turning 0s to 1s and vice versa. | ~A inverts all bits of A. |
<< (Left Shift) | Shifts the bits of the left operand to the left by a specified number of positions. | A << 2 shifts the bits of A two positions to the left. |
>> (Right Shift) | Shifts the bits of the left operand to the right by a specified number of positions. | A >> 3 shifts the bits of A three positions to the right. |
Bitwise operators are useful in scenarios where direct manipulation of binary representations or specific bit patterns is required, such as optimizing certain algorithms or working with hardware interfaces. Understanding bitwise operations is essential for low-level programming tasks.
C++
#include <iostream>
using namespace std;
int main()
{
// Bitwise AND (&)
int A = 5, B = 3;
cout << "(" << A << " & " << B << ") = " << (A & B) << endl;
// Bitwise OR (|)
cout << "(" << A << " | " << B << ") = " << (A | B) << endl;
// Bitwise XOR (^)
cout << "(" << A << " ^ " << B << ") = " << (A ^ B) << endl;
// Bitwise NOT (~)
cout << "~" << A << " = " << (~A) << endl;
// Left Shift (<<)
cout << "(" << A << " << 2) = " << (A << 2) << endl;
// Right Shift (>>)
cout << "(" << A << " >> 2) = " << (A >> 2) << endl;
return 0;
}
Java
class GFG {
public static void main(String[] args)
{
// Bitwise AND (&)
int A = 5, B = 3;
System.out.println("(" + A + " & " + B
+ ") = " + (A & B));
// Bitwise OR (|)
System.out.println("(" + A + " | " + B
+ ") = " + (A | B));
// Bitwise XOR (^)
System.out.println("(" + A + " ^ " + B
+ ") = " + (A ^ B));
// Bitwise NOT (~)
System.out.println("~" + A + " = " + (~A));
// Left Shift (<<)
System.out.println("(" + A
+ " << 2) = " + (A << 2));
// Right Shift (>>)
System.out.println("(" + A
+ " >> 2) = " + (A >> 2));
}
}
Python3
# Bitwise AND (&)
A, B = 5, 3
print(f"({A} & {B}) = {A & B}")
# Bitwise OR (|)
print(f"({A} | {B}) = {A | B}")
# Bitwise XOR (^)
print(f"({A} ^ {B}) = {A ^ B}")
# Bitwise NOT (~)
print(f"~{A} = {~A}")
# Left Shift (<<)
print(f"({A} << 2) = {A << 2}")
# Right Shift (>>)
print(f"({A} >> 2) = {A >> 2}")
JavaScript
class GFG {
static main() {
// Bitwise AND (&)
let A = 5, B = 3;
console.log(`(${A} & ${B}) = ${A & B}`);
// Bitwise OR (|)
console.log(`(${A} | ${B}) = ${A | B}`);
// Bitwise XOR (^)
console.log(`(${A} ^ ${B}) = ${A ^ B}`);
// Bitwise NOT (~)
console.log(`~${A} = ${~A}`);
// Left Shift (<<)
console.log(`(${A} << 2) = ${A << 2}`);
// Right Shift (>>)
console.log(`(${A} >> 2) = ${A >> 2}`);
}
}
// Call main function
GFG.main();
Output(5 & 3) = 1
(5 | 3) = 7
(5 ^ 3) = 6
~5 = -6
(5 << 2) = 20
(5 >> 2) = 1
In conclusion, operators in programming are essential for tasks like math, comparison, and logical decision-making. They handle basic operations, value comparison, and variable manipulation. Understanding these is crucial for efficient coding in different languages.
Share your thoughts in the comments
Please Login to comment...