Data Types in Programming
Last Updated :
26 Mar, 2024
In Programming, data type is an attribute associated with a piece of data that tells a computer system how to interpret its value. Understanding data types ensures that data is collected in the preferred format and that the value of each property is as expected.
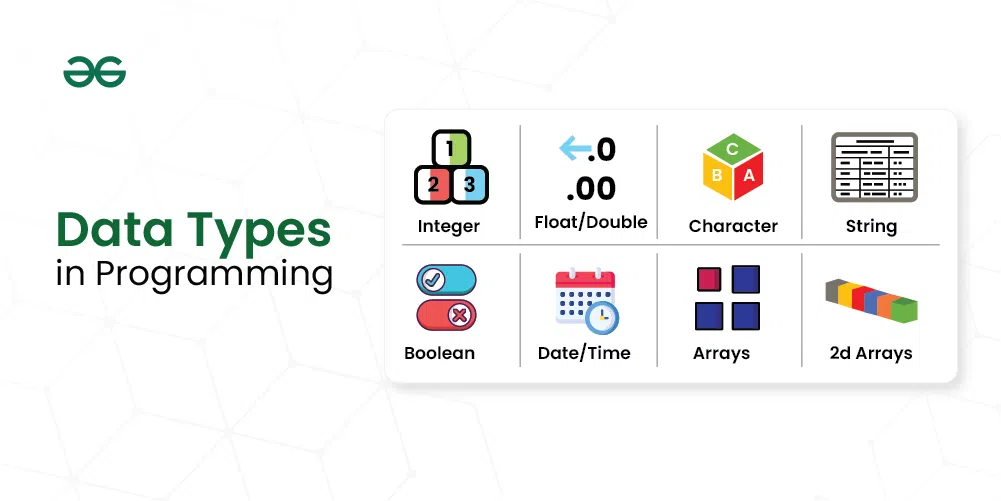
Data Types in Programming
What are Data Types in Programming?
An attribute that identifies a piece of data and instructs a computer system on how to interpret its value is called a data type.
The term “data type” in software programming describes the kind of value a variable possesses and the kinds of mathematical, relational, or logical operations that can be performed on it without leading to an error. Numerous programming languages, for instance, utilize the data types string, integer, and floating point to represent text, whole numbers, and values with decimal points, respectively. An interpreter or compiler can determine how a programmer plans to use a given set of data by looking up its data type.
The data comes in different forms. Examples include:
- your name – a string of characters
- your age – usually an integer
- the amount of money in your pocket- usually decimal type
- today’s date – written in date time format
Common Data Types in Programming:
.webp)
Data Types in Programming
1. Primitive Data Types:
Primitives are predefined data types that are independent of all other kinds and include basic values of particular attributes, like text or numeric values. They are the most fundamental type and are used as the foundation for more complex data types. Most computer languages probably employ some variation of these simple data types.
2. Composite Data Types:
Composite data types are made up of various primitive kinds that are typically supplied by the user. They are also referred to as user-defined or non-primitive data types. Composite types fall into four main categories: semi-structured (stores data as a set of relationships); multimedia (stores data as images, music, or videos); homogeneous (needs all values to be of the same data type); and tabular (stores data in tabular form).
3. User Defined Data Types:
A user-defined data type (UDT) is a data type that derived from an existing data type. You can use other built-in types already available and create your own customized data types.
Common Primitive Data Types in Programming:
Some common primitive datatypes are as follow:
Data Type
| Definition
| Examples
|
---|
Integer (int)
| represent numeric data type for numbers without fractions
|
300, 0 , -300
|
---|
Floating Point (float)
| represent numeric data type for numbers with fractions
|
34.67, 56.99, -78.09
|
---|
Character (char)
| represent single letter, digit, punctuation mark, symbol, or blank space
| a , 1, !
|
---|
Boolean (bool)
| True or false values
| true- 1, false- 0
|
---|
Date
| Date in the YYYY-MM-DD format (ISO 8601 syntax)
|
2024-01-01
|
---|
Time
| Time in the hh:mm:ss format for the time of day, time since an event, or time interval between events
|
12:34:20
|
---|
Datetime
| Date and time together in the YYYY-MM-DD hh:mm:ss format
|
2024 -01-01 12:34:20
|
---|
Common Composite Data Types:
Some common composite data types are as follow:
Data Type
| Definition
| Example
|
---|
String (string)
| Sequence of characters, digits, or symbols—always treated as text
| hello , ram , i am a girl
|
---|
array
| List with a number of elements in a specific order—typically of the same type
| arr[4]= [0 , 1 , 2 , 3 ]
|
---|
pointers
| Blocks of memory that are dynamically allocated are managed and stored
| *ptr=9
|
---|
Common User-Defined Data Types:
Some common user defined data types are as follow:
Data Type
| Definition
| Example
|
---|
Enumerated Type (enum)
| Small set of predefined unique values (elements or enumerators) that can be text-based or numerical
| Sunday -0, Monday -1
|
---|
Structure
| allows to combining of data items of different kinds
| struct s{ …}
|
---|
Union
| contains a group of data objects that can have varied data types
| union u {…}
|
---|
Static vs. Dynamic Typing in Programming:
Characteristic | Static Typing | Dynamic Typing |
---|
Definition of Data Types | Requires explicit definition of data types | Data types are determined at runtime |
---|
Type Declaration | Programmer explicitly declares variable types | Type declaration is not required |
---|
Error Detection | Early error detection during compile time | Errors may surface at runtime |
---|
Code Readability | Explicit types can enhance code readability | Code may be more concise but less explicit |
---|
Flexibility | Less flexible as types are fixed at compile time | More flexible, allows variable types to change |
---|
Compilation Process | Requires a separate compilation step | No separate compilation step needed |
---|
Example Languages | C, Java, Swift | Python, JavaScript, Ruby |
---|
Type Casting in Programming:
- Converting a single data type value—such as an integer int, float, or double—into another data type is known as typecasting. You have the option of doing this conversion manually or automatically. The conversion is done in two ways, automatically by the compiler and manually by a programmer.
- Type casting is sometimes known as type conversion. For example, a programmer can type cast a long variable value into an int if they wish to store it in the program as a simple integer. Thus, type casting is a technique that allows users to utilize the cast operator to change values from one data type to another.
- Type casting is used when imagine you have an age value, let’s say 30, stored in a program. You want to display a message on a website or application that says “Your age is: 30 years.” To display it as part of the message (a string), you would need to convert the age (an integer) to a string.
- Simple explanation of type casting can be done by this example:
- Imagine you have two types of containers: one for numbers and one for words. Now, let’s say you have a number written on a piece of paper, like “42,” and you want to put it in the container meant for words. Type casting is like taking that number, converting it into words, and then putting it in the container for words. Similarly, in programming, you might have a number (like 42) stored as one type, and you want to use it as if it were another type (like a word or text). Type casting helps you make that conversion.
Types of Type Casting:
The process of type casting can be performed in two major types in a C program. These are:
- Implicit – done internally by compiler.
- Explicit – done by programmer manually.
Syntax for Type Casting:
<datatype> variableName = (<datatype>) value;
Example of Type Casting:
1. Converting int into double
C++
#include <iostream>
using namespace std;
int main() {
int intValue = 54;
double doubleValue;
doubleValue = intValue;
cout << "int value: " << intValue << endl;
cout << "double value: " << doubleValue << endl;
return 0;
}
Java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int intValue = 54; // Declaring and initializing an integer variable
double doubleValue; // Declaring a double variable
doubleValue = intValue; // Implicit conversion from int to double
System.out.println("int value: " + intValue); // Printing the integer value
System.out.println("double value: " + doubleValue); // Printing the double value
}
}
Python
C#
using System;
class MainClass {
public static void Main (string[] args) {
int intValue = 54; // Declaring and initializing an integer variable
double doubleValue; // Declaring a double variable
doubleValue = intValue; // Implicit conversion from int to double
Console.WriteLine ("int value: " + intValue); // Printing the integer value
Console.WriteLine ("double value: " + doubleValue); // Printing the double value
}
}
JavaScript
// JavaScript program to demonstrate implicit type conversion
// Declaring and initializing an integer variable
let intValue = 54;
// Declaring a double variable
let doubleValue;
// Implicit conversion from int to double
doubleValue = intValue;
// Printing the integer value
console.log("int value: " + intValue);
// Printing the double value
console.log("double value: " + doubleValue);
Outputint value: 54
double value: 54
2. Automatic Conversion of double to int:
C++
#include <iostream>
using namespace std;
int main() {
double doubleValue = 54.7;
int intValue;
intValue = doubleValue;
cout << "double value: " << doubleValue << endl;
cout << "int value: " << intValue << endl;
return 0;
}
Java
public class Main {
public static void main(String[] args) {
// Declaring a double variable
double doubleValue = 54.7;
// Converting the double to an integer
int intValue = (int) doubleValue;
// Printing the double value
System.out.println("double value: " + doubleValue);
// Printing the integer value
System.out.println("int value: " + intValue);
}
}
C#
using System;
class MainClass {
public static void Main (string[] args) {
double doubleValue = 54.7; // Declaring and initializing a double variable
int intValue; // Declaring an integer variable
intValue = (int)doubleValue; // Explicitly casting the double value to an int
Console.WriteLine ("double value: " + doubleValue); // Printing the double value
Console.WriteLine ("int value: " + intValue); // Printing the integer value
}
}
JavaScript
// Declaring a double variable
const doubleValue = 54.7;
// Converting the double to an integer
const intValue = Math.floor(doubleValue);
// Printing the double value
console.log("double value: " + doubleValue);
// Printing the integer value
console.log("int value: " + intValue);
Python3
# The code declares a double variable, assigns a value to it,
# converts it to an integer, and prints both the double and integer values.
# Declaring a double variable
double_value = 54.7
# Converting the double to an integer
int_value = int(double_value)
# Printing the double value
print("double value:", double_value)
# Printing the integer value
print("int value:", int_value)
Outputdouble value: 54.7
int value: 54
Variables and Data Types in Programming:
The name of the memory area where data can be stored is called a variable. In a program, variables are used to hold data; they have three properties: name, value, and type. A variable’s value may fluctuate while a program is running.
Data type characterizes a variable’s attribute; actions also rely on the data type of the variables, as does the data that is stored in variables. The sorts of data that a variable can store are specified by its data types. Numerous built-in data types, including int, float, double, char, and bool, are supported by C programming. Every form of data has a range of values that it can store and a memory usage limit.
Example: Imagine a box labeled “age.” You can put a number like 25 in it initially, and later, you might change it to 30. A box labeled “number” is designed for holding numbers (like 42). Another box labeled “name” is designed for holding words or text (like “John”). So, in simple terms, a variable is like a labeled box where you can put things, and the data type is like a tag on the box that tells you what kind of things it can hold. Together, they help the computer understand and manage the information you’re working with in a program.
Type Safety in Programming:
Type safety in a programming language is an abstract construct that enables the language to avoid type errors.
There is an implicit level of type safety in all programming languages. Therefore, the compiler will use the type safety construct to validate types during program compilation, and it will raise an error if we attempt to assign the incorrect type to a variable. Type safety is verified during a program’s runtime in addition to during compilation. The type safety feature makes sure that no improper operations are carried out on the underlying object by the code.
Take the machine’s 32-bit quantity, for instance. It can be used to represent four ASCII characters, an int, or a floating point. In light of the situation, these interpretations might be accurate. For example, when using assembly, the programmer bears full responsibility for maintaining track of the data types. When a machine-level floating point addition is performed on a 32-bit number that actually represents an integer, the result is indeterminate, which means that the outcomes may vary from computer to computer.
In Conclusion, Programmers can create dependable and efficient code by utilizing data types. Data types can help organizations manage their data more effectively, from collection to integration, in addition to helping programmers write more effective code.
- Data types are the basis of programming languages.
- There are various kind of data types available according to the various kind of data available.
- Data types are of 3 types.
- Primitive Data type: int, float, char, bool
- Composite Data Types: string, array, pointers
- User Defined Data Type
Share your thoughts in the comments
Please Login to comment...