Operators in programming are essential symbols that perform operations on variables and values, enabling tasks like arithmetic calculations, logical comparisons, and bitwise manipulations. In this article, we will learn about the basics of operators and their types.
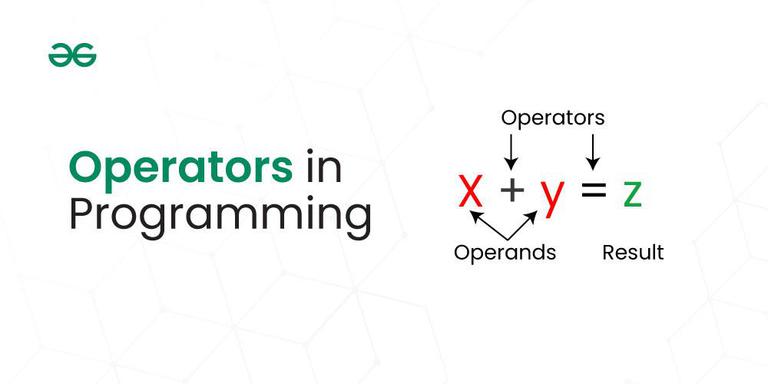
Operators in Programming
What are Operators in Programming?
Operators in programming are symbols or keywords that represent computations or actions performed on operands. Operands can be variables, constants, or values, and the combination of operators and operands form expressions. Operators play a crucial role in performing various tasks, such as arithmetic calculations, logical comparisons, bitwise operations, etc.
+ |
Addition |
Arithmetic Operators |
Adds two values |
result = num1 + num2; |
– |
Subtraction |
Subtracts the right operand from the left |
result = num1 – num2; |
* |
Multiplication |
Multiplies two values |
result = num1 * num2; |
/ |
Division |
Divides the left operand by the right |
result = num1 / num2; |
% |
Modulus |
Returns the remainder of division |
result = num1 % num2; |
++ |
Increment |
Unary Operators |
Increases the value of a variable by 1 |
num++; or ++num; |
— |
Decrement |
Decreases the value of a variable by 1 |
num–; or –num; |
= |
Assignment |
Assignment Operators |
Assigns a value to a variable |
x = 10; |
+= |
Add and Assign |
Adds the right operand to the left and assigns |
x += 5; (equivalent to x = x + 5;) |
-= |
Subtract and Assign |
Subtracts the right operand and assigns |
x -= 3; (equivalent to x = x – 3;) |
*= |
Multiply and Assign |
Multiplies the right operand and assigns |
x *= 2; (equivalent to x = x * 2;) |
/= |
Divide and Assign |
Divides the left operand and assigns |
x /= 4; (equivalent to x = x / 4;) |
%= |
Modulus and Assign |
Computes modulus and assigns |
x %= 3; (equivalent to x = x % 3;) |
== |
Equal to |
Relational or Comparison Operators |
Tests if two values are equal |
if (a == b) |
!= |
Not Equal to |
Tests if two values are not equal |
if (a != b) |
< |
Less Than |
Tests if the left value is less than the right |
if (a < b) |
> |
Greater Than |
Tests if the left value is greater than right |
if (a > b) |
<= |
Less Than or Equal To |
Tests if the left value is less than or equal |
if (a <= b) |
>= |
Greater Than or Equal To |
Tests if the left value is greater than or equal |
if (a >= b) |
&& |
Logical AND |
Logical Operators |
Returns true if both operands are true |
if (a && b) |
|| |
Logical OR |
|
|
! |
Logical NOT |
Reverses the logical state of its operand |
if (!condition) |
& |
Bitwise AND |
Bitwise Operators |
Performs bitwise AND on individual bits |
result = a & b; |
` |
Bitwise OR |
Performs bitwise OR on individual bits |
|
^ |
Bitwise XOR |
Performs bitwise XOR on individual bits |
result = a ^ b; |
~ |
Bitwise NOT |
Inverts the bits of its operand |
result = ~a; |
<< |
Left Shift |
Shifts bits to the left |
result = a << 2; |
>> |
Right Shift |
Shifts bits to the right |
result = a >> 1; |
?: |
Conditional (Ternary) |
Conditional Operator |
Evaluates a condition and returns one of two values |
result = (condition) ? value1 : value2; |
Types of Operators in Programming:
Here are some common types of operators:
- Arithmetic Operators: Perform basic arithmetic operations on numeric values. Examples: + (addition), – (subtraction), * (multiplication), / (division), % (modulo).
- Comparison Operators: Compare two values and return a Boolean result (true or false). Examples: == (equal to), != (not equal to), < (less than), > (greater than), <= (less than or equal to), >= (greater than or equal to).
- Logical Operators: Perform logical operations on Boolean values. Examples: && (logical AND), || (logical OR), ! (logical NOT).
- Assignment Operators: Assign values to variables. Examples: = (assign), += (add and assign), -=, *= (multiply and assign), /=, %=.
- Increment and Decrement Operators: Increase or decrease the value of a variable by 1. Examples: ++ (increment), — (decrement).
- Bitwise Operators: Perform operations on individual bits of binary representations of numbers. Examples: & (bitwise AND), | (bitwise OR), ^ (bitwise XOR), ~ (bitwise NOT), << (left shift), >> (right shift).
These operators provide the building blocks for creating complex expressions and performing diverse operations in programming languages. Understanding their usage is crucial for writing efficient and expressive code.
Arithmetic Operators in Programming:
Arithmetic operators in programming are fundamental components of programming languages, enabling the manipulation of numeric values for various computational tasks. Here’s an elaboration on the key arithmetic operators:
+ (Addition) |
Combines two numeric values, yielding their sum. |
result = 5 + 3; (result will be 8) |
– (Subtraction) |
Subtracts the right operand from the left operand. |
difference = 10 - 4; (difference will be 6) |
* (Multiplication) |
Multiplies two numeric values, producing their product. |
product = 3 * 7; (product will be 21) |
/ (Division) |
Divides the left operand by the right operand, producing a quotient. |
quotient = 15 / 3; (quotient will be 5) |
% (Modulo) |
Returns the remainder after the division of the left operand by the right operand. |
remainder = 10 % 3; (remainder will be 1) |
Comparison Operators in Programming:
Comparison operators in programming are used to compare two values or expressions and return a Boolean result indicating the relationship between them. These operators play a crucial role in decision-making and conditional statements. Here are the common comparison operators:
== (Equal to) |
Checks if the values on both sides are equal. |
5 == 5; (evaluates to true) |
!= (Not equal to) |
Checks if the values on both sides are not equal. |
10 != 5; (evaluates to true) |
< (Less than) |
Tests if the value on the left is less than the value on the right. |
3 < 7; (evaluates to true) |
> (Greater than) |
Tests if the value on the left is greater than the value on the right. |
10 > 8; (evaluates to true) |
<= (Less than or equal to) |
Checks if the value on the left is less than or equal to the value on the right. |
5 <= 5; (evaluates to true) |
>= (Greater than or equal to) |
Checks if the value on the left is greater than or equal to the value on the right. |
8 >= 8; (evaluates to true) |
These operators are extensively used in conditional statements, loops, and decision-making constructs to control the flow of a program based on the relationship between variables or values. Understanding comparison operators is crucial for creating logical and effective algorithms in programming.
Logical Operators in Programming:
Logical operators in programming are used to perform logical operations on Boolean values. These operators are crucial for combining or manipulating conditions and controlling the flow of a program based on logical expressions. Here are the common logical operators:
&& (Logical AND) |
Returns true if both operands are true; otherwise, it returns false. |
true && false; (evaluates to false) |
(||) Logical OR |
Returns true if at least one of the operands is true; otherwise, it returns false |
true || false; (evaluates to true)
|
! (Logical NOT) |
Returns true if the operand is false and vice versa; it negates the Boolean value. |
!true; (evaluates to false) |
These logical operators are frequently used in conditional statements (if, else if, else), loops, and decision-making constructs to create complex conditions based on multiple Boolean expressions. Understanding how to use logical operators is essential for designing effective and readable control flow in programming.
Assignment Operators in Programming:
Assignment operators in programming are used to assign values to variables. They are essential for storing and updating data within a program. Here are common assignment operators:
= (Assignment) |
Assigns the value on the right to the variable on the left. |
x = 10; assigns the value 10 to the variable x. |
+= (Addition Assignment) |
Adds the value on the right to the current value of the variable on the left and assigns the result to the variable. |
x += 5; is equivalent to x = x + 5; |
-= (Subtraction Assignment) |
Subtracts the value on the right from the current value of the variable on the left and assigns the result to the variable. |
y -= 3; is equivalent to y = y - 3; |
*= (Multiplication Assignment) |
Multiplies the current value of the variable on the left by the value on the right and assigns the result to the variable. |
z *= 2; is equivalent to z = z * 2; |
/= (Division Assignment) |
Divides the current value of the variable on the left by the value on the right and assigns the result to the variable. |
a /= 4; is equivalent to a = a / 4; |
%= (Modulo Assignment) |
Calculates the modulo of the current value of the variable on the left and the value on the right, then assigns the result to the variable. |
b %= 3; is equivalent to b = b % 3; |
Assignment operators are fundamental for updating variable values, especially in loops and mathematical computations, contributing to the dynamic nature of programming. Understanding how to use assignment operators is essential for effective variable manipulation in a program.
Increment and Decrement Operators in Programming:
Increment and decrement operators in programming are used to increase or decrease the value of a variable by 1, respectively. They are shorthand notations for common operations and are particularly useful in loops. Here are the two types:
++ (Increment) |
Increases the value of a variable by 1. |
x++; is equivalent to x = x + 1; or x += 1; |
— (Decrement) |
Decreases the value of a variable by 1. |
y--; is equivalent to y = y - 1; or y -= 1; |
These operators are frequently employed in loops, especially for iterating through arrays or performing repetitive tasks. Their concise syntax enhances code readability and expressiveness.
Bitwise Operators in Programming:
Bitwise operators in programming perform operations at the bit level, manipulating individual bits of binary representations of numbers. These operators are often used in low-level programming, such as embedded systems and device drivers. Here are the common bitwise operators:
& (Bitwise AND) |
Performs a bitwise AND operation between corresponding bits of two operands. |
A & B sets each bit to 1 if both corresponding bits in A and B are 1. |
| (Bitwise OR) |
Performs a bitwise OR operation between corresponding bits of two operands. |
A | B sets each bit to 1 if at least one corresponding bit in A or B is 1. |
^ (Bitwise XOR) |
Performs a bitwise XOR (exclusive OR) operation between corresponding bits of two operands. |
A ^ B sets each bit to 1 if the corresponding bits in A and B are different. |
~ (Bitwise NOT) |
Inverts the bits of a single operand, turning 0s to 1s and vice versa. |
~A inverts all bits of A. |
<< (Left Shift) |
Shifts the bits of the left operand to the left by a specified number of positions. |
A << 2 shifts the bits of A two positions to the left. |
>> (Right Shift) |
Shifts the bits of the left operand to the right by a specified number of positions. |
A >> 3 shifts the bits of A three positions to the right. |
Bitwise operators are useful in scenarios where direct manipulation of binary representations or specific bit patterns is required, such as optimizing certain algorithms or working with hardware interfaces. Understanding bitwise operations is essential for low-level programming tasks.
Operator Precedence and Associativity in Programming:
Operator Precedence is a rule that determines the order in which operators are evaluated in an expression. It defines which operators take precedence over others when they are combined in the same expression. Operators with higher precedence are evaluated before operators with lower precedence. Parentheses can be used to override the default precedence and explicitly specify the order of evaluation.
Operator Associativity is a rule that determines the grouping of operators with the same precedence in an expression when they appear consecutively. It specifies the direction in which operators of equal precedence are evaluated. The two common associativities are:
- Left to Right (Left-Associative): Operators with left associativity are evaluated from left to right. For example, in the expression
a + b + c
, the addition operators have left associativity, so the expression is equivalent to (a + b) + c
.
- Right to Left (Right-Associative): Operators with right associativity are evaluated from right to left. For example, in the expression
a = b = c
, the assignment operator =
has right associativity, so the expression is equivalent to a = (b = c)
.
1 |
() |
Parentheses |
Left-to-Right |
x++, x– |
Postfix increment, decrement |
2 |
++x, –x |
Prefix increment, decrement |
Right-to-Left |
‘+’ , ‘-‘ |
Unary plus, minus |
! , ~ |
Logical NOT, Bitwise complement |
* |
Dereference Operator |
& |
Addressof Operator |
3 |
*, /, % |
Multiplication, division, modulus |
Left-to-Right |
4 |
+, – |
Addition, subtraction |
Left-to-Right |
5 |
<< , >> |
Bitwise shift left, Bitwise shift right |
Left-to-Right |
6 |
< , <= |
Relational less than, less than or equal to |
Left-to-Right |
> , >= |
Relational greater than, greater than or equal to |
7 |
== , != |
Relational is equal to, is not equal to |
Left-to-Right |
8 |
& |
Bitwise AND |
Left-to-Right |
9 |
^ |
Bitwise XOR |
Left-to-Right |
10 |
| |
Bitwise OR |
Left-to-Right |
11 |
&& |
Logical AND |
Left-to-Right |
12 |
|| |
Logical OR |
Left-to-Right |
13 |
?: |
Ternary conditional |
Right-to-Left |
14 |
= |
Assignment |
Right-to-Left |
+= , -= |
Addition, subtraction assignment |
*= , /= |
Multiplication, division assignment |
%= , &= |
Modulus, bitwise AND assignment |
^= , |= |
Bitwise exclusive, inclusive OR assignment |
<<=, >>= |
Bitwise shift left, right assignment |
15 |
, |
comma (expression separator) |
Left-to-Right |
Here are some frequently asked questions (FAQs) related to programming operators:
Q1: What are operators in programming?
A: Operators in programming are symbols that represent computations or actions to be performed on operands. They can manipulate data, perform calculations, and facilitate various operations in a program.
Q2: How are operators categorized?
A: Operators are categorized based on their functionality. Common categories include arithmetic operators (for mathematical operations), assignment operators (for assigning values), comparison operators (for comparing values), logical operators (for logical operations), and bitwise operators (for manipulating individual bits).
Q3: What is the difference between unary and binary operators?
A: Unary operators operate on a single operand, while binary operators operate on two operands. For example, the unary minus -x
negates the value of x
, while the binary plus a + b
adds the values of a
and b
.
Q4: Can operators be overloaded in programming languages?
A: Yes, some programming languages support operator overloading, allowing developers to define custom behaviors for operators when applied to user-defined types. This is commonly seen in languages like C++.
Q5: How do logical AND (&&
) and logical OR (||
) operators work?
A: The logical AND (&&
) operator returns true if both of its operands are true. The logical OR (||
) operator returns true if at least one of its operands is true. These operators are often used in conditional statements and expressions.
Q6: What is the purpose of the ternary operator (?:
)?
A: The ternary operator is a shorthand for an if-else
statement. It evaluates a condition and returns one of two values based on whether the condition is true or false. It is often used for concise conditional assignments.
Q7: How does the bitwise XOR operator (^
) work?
A: The bitwise XOR (^
) operator performs an exclusive OR operation on individual bits. It returns 1 for bits that are different and 0 for bits that are the same. This operator is commonly used in bit manipulation tasks.
Q8: What is the difference between =
and ==
?
A: The =
operator is an assignment operator used to assign a value to a variable. The ==
operator is a comparison operator used to check if two values are equal. It is important not to confuse the two, as =
is used for assignment, and ==
is used for comparison.
Q9: How do increment (++
) and decrement (--
) operators work?
A: The increment (++
) operator adds 1 to the value of a variable, while the decrement (--
) operator subtracts 1. These operators can be used as prefix (++x
) or postfix (x++
), affecting the order of the increment or decrement operation.
Q10: Are operators language-specific?
A: While many operators are common across programming languages, some languages may introduce unique operators or have variations in their behavior. However, the fundamental concepts of arithmetic, logical, and bitwise operations are prevalent across various languages.
Q11: What is the modulus operator (%
) used for?
A: The modulus operator (%
) returns the remainder when one number is divided by another. It is often used to check divisibility or to cycle through a sequence of values. For example, a % 2
can be used to determine if a
is an even or odd number.
Q12: Can the same operator have different meanings in different programming languages?
A: Yes, the same symbol may represent different operators or operations in different programming languages. For example, the +
operator is used for addition in most languages, but in some languages (like JavaScript), it is also used for string concatenation.
Q13: What is short-circuit evaluation in the context of logical operators?
A: Short-circuit evaluation is a behavior where the second operand of a logical AND (&&
) or logical OR (||
) operator is not evaluated if the outcome can be determined by the value of the first operand alone. This can lead to more efficient code execution.
Q14: How are bitwise left shift (<<
) and right shift (>>
) operators used?
A: The bitwise left shift (<<
) operator shifts the bits of a binary number to the left, effectively multiplying the number by 2. The bitwise right shift (>>
) operator shifts the bits to the right, effectively dividing the number by 2.
Q15: Can you provide an example of operator precedence in programming?
A: Operator precedence determines the order in which operators are evaluated. For example, in the expression a + b * c
, the multiplication (*
) has higher precedence than addition (+
), so b * c
is evaluated first.
Q16: How does the ternary operator differ from an if-else
statement?
A: The ternary operator (?:
) is a concise way to express a conditional statement with a single line of code. It returns one of two values based on a condition. An if-else
statement provides a more extensive code block for handling multiple statements based on a condition.
Q17: Are there any operators specifically designed for working with arrays or collections?
A: Some programming languages provide specific operators or methods for working with arrays or collections. For example, in Python, the in
operator is used to check if an element is present in a list.
Q18: How can bitwise operators be used for efficient memory management?
A: Bitwise operators are often used for efficient memory management by manipulating individual bits. For example, bitwise AND can be used to mask specific bits, and bitwise OR can be used to set particular bits.
Q19: Can operators be overloaded in all programming languages?
A: No, not all programming languages support operator overloading. While some languages like C++ allow developers to redefine the behavior of operators for user-defined types, others, like Java, do not permit operator overloading.
Q20: How do you handle operator precedence in complex expressions?
A: Parentheses are used to explicitly specify the order of operations in complex expressions, ensuring that certain operations are performed before others. For example, (a + b) * c
ensures that addition is performed before multiplication.
In conclusion, operators in programming are essential tools that enable the manipulation, comparison, and logical operations on variables and values. They form the building blocks of expressions and play a fundamental role in controlling program flow, making decisions, and performing calculations. From arithmetic and comparison operators for numerical tasks to logical operators for decision-making, and bitwise operators for low-level bit manipulation, each type serves specific purposes in diverse programming scenarios.
Share your thoughts in the comments
Please Login to comment...