Python has become popular in various tech fields and image processing is one of them. This is all because of a vast collection of libraries that can provide a wide range of tools and functionalities for manipulating, analyzing, and enhancing images. Whether someone is a developer working on image applications, a researcher, or a machine learning engineer there is a Python library for image processing that fulfills their requirement. In this article, we will learn about top Python libraries used for image processing.
What is Image Processing?
Image processing is the process of analysis, manipulation, and interpretation of images using computational power. Various algorithms and methods are used to transform, enhance, or extract information from images. Image processing is used in various fields which include medical imaging, robotics, self-driving cars, computer vision, and more. One of the common examples is the face lock used in computers and mobile phones. The key objectives of image processing include:
- Image Enhancement
- Image Restoration
- Image Compression
- Image Segmentation
- Object Recognition
- Image Registration
- Feature Extraction
- Geometric Transformation
- Color Image Processing
- Pattern Recognition
Top Python Libraries for Image Processing
Computer vision is a part of artificial intelligence (AI) that helps computers understand and use information from pictures, videos, and other visuals. It lets them do things or give suggestions based on what they see. To get this information, image processing is used. Image processing means changing or working on an image to pick out important things from it. Now let’s discuss the most used libraries for image processing in Python.
OpenCV
OpenCV is a huge open-source library for computer vision, machine learning, and image processing. Numerous programming languages, including Python, C++, Java, and others, are supported by OpenCV. It can recognize faces, objects, and even human handwriting by processing photos and movies. The number of weapons in your arsenal grows as it is merged with different libraries, including NumPy, a highly efficient library for numerical operations. This is because OpenCV can combine every operation that can be done with NumPy. We can install OpenCV library in Python by executing the below command in the terminal.
pip install opencv-python
Grey Scaling using OpenCV
You can download the image from here.
Python3
import cv2 as cv
import numpy as np
import matplotlib.pyplot as plt
input_image = cv.imread( 'rose.jpg' )
gray_image = cv.cvtColor(input_image, cv.COLOR_BGR2GRAY)
fig, ax = plt.subplots( 1 , 2 , figsize = ( 16 , 8 ))
fig.tight_layout()
ax[ 0 ].imshow(cv.cvtColor(input_image, cv.COLOR_BGR2RGB))
ax[ 0 ].set_title( "Original Image" )
ax[ 1 ].imshow(gray_image, cmap = 'gray' )
ax[ 1 ].set_title( "Grayscale Image" )
plt.show()
|
Output:
-min.png)
SciPy
A Python package called Scipy is helpful for resolving a variety of mathematical problems and procedures. It is built upon the NumPy library, which provides further flexibility in locating scientific mathematical formulas, including LU Decomposition, polynomial equations, matrix rank, and inverse. By utilizing its high-level functions, the code’s complexity will be greatly reduced, improving data analysis. While the SciPy library is not primarily focused on image processing, it provides several modules that are highly useful for handling and manipulating images. We can install SciPy in Python by executing below command in terminal.
python -m pip install scipy
Here are some key aspects of SciPy in terms of image processing:
- Image Filters and Operations
- Image Morphology
- Image Measurements
- Interpolation and Geometric Transformations
- Integration with NumPy and Matplotlib
Blurring the Image using SciPy and Matplotlib
We’re using SciPy’s ndimage.gaussian_filter function to apply Gaussian blur to the input image.
Python3
import matplotlib.pyplot as plt
from scipy import ndimage, misc
image_path = 'rose.jpg'
input_image = plt.imread(image_path)
blurred_image = ndimage.gaussian_filter(input_image, sigma = 10 )
fig, axes = plt.subplots( 1 , 2 , figsize = ( 12 , 6 ))
axes[ 0 ].imshow(input_image)
axes[ 0 ].set_title( 'Original Image' )
axes[ 0 ].axis( 'off' )
axes[ 1 ].imshow(blurred_image)
axes[ 1 ].set_title( 'Blurred Image' )
axes[ 1 ].axis( 'off' )
plt.show()
|
Output:
-min.png)
ImageIO
ImageIO is a Python library developed with the purpose of reading and writing images in various formats. It simplifies the process of working with images by providing a unified interface for different file formats. ImageIO supports a wide range of image and video formats, making it a handy tool for multimedia applications. To install ImageIO library in Python execute the below command in terminal.
pip install imageio
Here are some key features of ImageIO library in Python:
- Image Input and Output
- Working with Video Files
- Animated Image Support
- Integration with NumPy
- Flexible and Extensible
Reading Image using ImageIO
Python3
import imageio
import matplotlib.pyplot as plt
image_path = 'rose.jpg'
input_image = imageio.imread(image_path)
plt.imshow(input_image)
plt.axis( 'off' )
plt.show()
|
Output:
-min.png)
Scikit-Image
Scikit-Image is a Python module for image processing that utilizes NumPy arrays, a set of image processing methods. It provides a collection of algorithms for image processing, computer vision, and computer graphics. It is designed to be user-friendly, efficient, and suitable for a wide range of image processing tasks. We can install Scikit-Image library in Python by executing below command in the terminal.
pip install scikit-image
Here are some key features of Scikit-image library in Python:
- Rich Collection of Algorithms
- Easy Integration with NumPy
- Image Segmentation
- Feature Extraction
- Image Restoration
- Visualization Tools
Edge Detection using Scikit-image
Python3
import matplotlib.pyplot as plt
from skimage import io, color, filters
image_path = 'rose.jpg'
input_image = io.imread(image_path)
edges = filters.sobel(input_image)
fig, axes = plt.subplots( 1 , 2 , figsize = ( 12 , 6 ))
axes[ 0 ].imshow(input_image)
axes[ 0 ].set_title( 'Original Image' )
axes[ 0 ].axis( 'off' )
axes[ 1 ].imshow(edges, cmap = 'gray' )
axes[ 1 ].set_title( 'Edge Detection' )
axes[ 1 ].axis( 'off' )
plt.show()
|
Output:
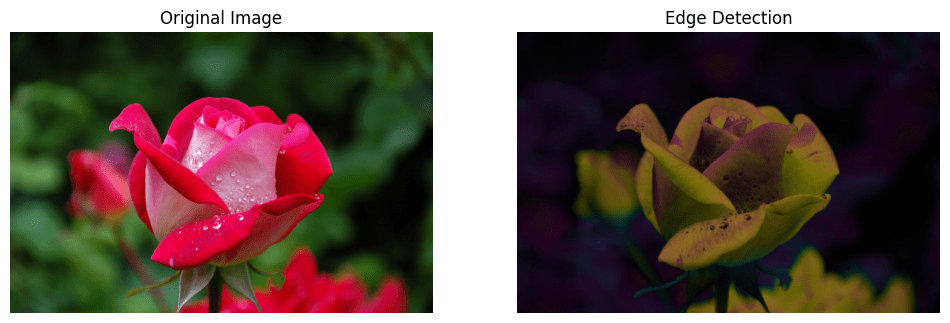
Python Image Library (PIL/Pillow)
Python Imaging Library (an extension of PIL) is the de facto image processing package for the Python language. It includes simple image processing capabilities to help with image creation, editing, and archiving. In 2011, support for the Python Imaging Library was stopped; however, a project called pillow forked the PIL project and added compatibility for Python 3.x. It was declared that Pillow will take the place of PIL going forward. Pillow is compatible with a wide range of image file types, such as TIFF, JPEG, PNG, and BMP. The library promotes developing new file decoders in order to add support for more recent formats. We can install PIL/Pillow library in Python by executing below command in the terminal.
pip install pillow
Here are some key features of PIL/Pillow library in Python:
- Image Opening and Saving
- Image Manipulation
- Image Filtering and Enhancements
- Image Drawing
- Image Conversion and Formats
- Integration with NumPy
- Handling Animated Images
Cropping Image using Pillow
Python3
from PIL import Image
image = Image. open ( 'rose.jpg' )
box = ( 500 , 500 , 2500 , 2500 )
cropped_image = image.crop(box)
cropped_image.save( 'cropped_image.jpg' )
print (cropped_image.size)
plt.imshow(cropped_image)
plt.axis( 'on' )
plt.show()
|
Output:
-min.png)
Mahotas
Mahotas is a Python library used for computer vision, image processing, and manipulation. It is designed to be fast and efficient, making it suitable for real-time image processing applications. Mahotas builds on the strengths of NumPy and focuses on providing a wide range of algorithms to perform various tasks such as filtering, edge detection, morphology, and feature extraction. Mahotas is an array-based algorithm suite that has more than 100 functions for computer vision and image processing, and it is still expanding. To install Mahotas library in Python execute the below command in the terminal.
pip install mahotas
Here are some key features of the Mahotas library:
- Fast Image Processing
- Filtering and Convolution
- Morphological Operations
- Feature Extraction
- Image Segmentation
- Histograms and Thresholding
- Distance Transform
- Integration with NumPy
Converting the Image in Different Tone using Mahotas
Python3
import mahotas as mh
import matplotlib.pyplot as plt
image = mh.imread( 'rose.jpg' )
output_image = mh.colors.rgb2sepia(image)
plt.imshow(output_image)
plt.axis( 'on' )
plt.show()
|
Output:
-min.png)
Matplotlib
Matplotlib is a Python visualization package for two-dimensional array charts. Matplotlib is based on NumPy array and a multi-platform data visualization package intended to be used with the larger SciPy stack. In the year 2002, John Hunter introduced Matplotlib. The ability to visually access vast volumes of data in a format that is simple to understand is one of visualization’s biggest advantages. Many plot types, including line, bar, scatter, histogram, and more, are available in Matplotlib. We can install Matplotlib by executing below command.
pip install matplotlib
Here are some key features of Matplotlib in the context of image processing:
- Image Display
- Subplots and Multi-image Display
- Histograms
- Visualizing Filters
- Custom Annotations and Overlays
- Color Maps and Colorbars
- Saving Figures
SimpleCV
SimpleCV is an open-source framework used for computer vision tasks. It has simplified computer vision, as its name would imply. SimpleCV offers easy-to-use practices for completing common computer vision tasks, eliminating the complexity associated with OpenCV. Installing SimpleCV on Linux, Windows, and Mac is possible on all major operating systems, even those developed in Python. It can be obtained under a BSD license. Developers may work with both photos and videos using SimpleCV. We can install SimpleCV by using below command in the terminal.
pip install SimpleCV
Here are some key features of SimpleCV:
- Easy Image Processing
- Computer Vision Utilities
- Camera Support
- Integration with NumPy
- Feature Detection and Matching
- Interactive Shell
- Extensibility
SimpleITK
SimpleITK stands for simple Insight Segmentation and Registration Toolkit. It is a powerful open source library implemented in C++ and used for medical image analysis. It offers a wide range of functionalities to address various image processing challenges encountered in medical research and clinical practice. To install this library, execute below command in the terminal.
pip install SimpleITK
Here are some key features of SimpleCV:
- Simplified Interface
- Image Loading and Manipulation
- Image Registration
- Image Segmentation
- Image Filtering
- Image Visualization
- Cross-Platform Compatibility
Pgmagick
Pgmagick is a Python binding for GraphicsMagick that offers several image manipulation functions, including text drawing, gradient picture creation, sharpening, resizing, and rotating. To install this library execute below command in the teminal.
pip install pgmagick
Here are some key features of Pgmagick:
- Image Manipulation
- Image Filtering and Effects
- Drawing and Annotation
- Image Analysis
- Batch Processing
- High Performance
- Cross-Platform Compatibility
Share your thoughts in the comments
Please Login to comment...