Swagger and API Lifecycle Management
Last Updated :
26 Mar, 2024
In today’s world of software development and integration, Application Programming Interfaces (APIs) play a crucial role in enabling communication and data exchange between different systems. However, creating an API involves multiple steps, and effective management throughout its lifecycle is vital to ensure usability, reliability, and scalability.
Swagger and API Lifecycle Management
Below, we will see some concepts related to Swagger and API Lifecycle Management.
What is API Lifecycle Management?
API lifecycle management consists of good planning, designing, developing, testing, deploying, and retiring of APIs. So making it easy we have a Swagger tool called “SwaggerHub” which helps with every step of the API journey. This is user-friendly and flexible.
Advantages
API lifecycle management involves planning, designing, developing, testing, deploying, and retiring APIs. SwaggerHub, a user-friendly and flexible Swagger tool, aids in every step of the API journey, making the process more accessible.
- Increased Productivity: Standardized processes in API design and development reduce confusion, leading to improved collaboration and sustainable productivity gains.
- Greater Visibility: Lifecycle management provides a clear roadmap for API projects, aiding in effective monitoring of API health, performance, and usage.
- Organizational Alignment: Establishing a common vocabulary and framework for API-related tasks promotes better communication and alignment within the organization, improving overall efficiency.
Swagger and API Lifecycle Management in Action:
Swagger, now known as the OpenAPI Specification (OAS), plays a vital role in ensuring consistency, security, versioning, performance monitoring, and collaboration throughout an API’s lifecycle. Let’s explore how Swagger and API lifecycle management intersect at each stage:
Five Stages of API Lifecycle
- Planning and Designing the API: Before creating an API, planning and designing involve mapping resources, operations, and analyzing requirements. SwaggerHub provides a robust Editor experience for designing API solutions, efficiently mapping out API functionalities.
- Developing the API: The development phase implements the API based on the plan and design. SwaggerHub’s tools, like Swagger Codegen, generate the basic code needed to start building the API, making development smoother.
- Testing the API: Testing is crucial to ensure the API behaves as expected. SwaggerHub facilitates easy testing, ensuring thorough testing and integration with tools like SoapUI and LoadUI.
- Deploying the API: Deploying the API to a secure environment makes it accessible to users. SwaggerHub aids in securely deploying APIs to platforms like AWS API Gateway, IBM API Connect, or Microsoft Azure.
- Retiring the API: Deprecating outdated API versions is a natural part of the lifecycle. SwaggerHub helps organizations mark APIs as deprecated and notifies users through features like webhooks.
Swagger and API Lifecycle Management Example
Below, are the code example of Swagger and API Lifecycle Management.
Create a Virtual Environment
First, create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
Code Explanation
Below, are the step-by-step code explanation of Swagger and API Lifecycle Management example.
Step 1: Flask Setup and API Configuration
below, code initializes a Flask application (app
) and sets up a RESTful API using the Flask-RESTx extension (api
). It also imports necessary modules and classes for handling file uploads (FileStorage
), image processing (PIL.Image
), and defining API resources.
Python3
from flask import Flask, request, send_file
from flask_restx import Api, Resource, reqparse
from werkzeug.datastructures import FileStorage
from PIL import Image
import os
app = Flask(__name__)
api = Api(app, version='1.0', title='Image Upload API',
description='API for uploading and displaying images')
Step 2: API Resource for Image Upload
below, code defines an API resource (ImageUpload
) for handling image uploads. It specifies a POST method that expects a file upload (image
parameter) and saves the uploaded image to a specified directory (image_store
). Additionally, it resizes the uploaded image to a maximum size of 300×300 pixels and saves the resized image to the same directory. The API endpoint for image uploads is /upload
.
Python3
upload_parser = api.parser()
upload_parser.add_argument('image', location='files',
type=FileStorage, required=True,
help='Image file')
image_store = "uploaded_images"
if not os.path.exists(image_store):
os.makedirs(image_store)
@api.route('/upload')
class ImageUpload(Resource):
@api.expect(upload_parser)
def post(self):
args = upload_parser.parse_args()
image_file = args['image']
image_path = os.path.join(image_store,
image_file.filename)
image_file.save(image_path)
# Resize image if needed
resized_path = os.path.join(image_store,
"resized_" + image_file.filename)
with Image.open(image_path) as img:
img.thumbnail((300, 300))
img.save(resized_path)
return {'message': 'Image uploaded successfully',
'image_path': resized_path}
Step 3: API Resource for Image Display
below, code defines another API resource (ImageDisplay
) for retrieving and displaying images. It specifies a GET method that takes the image name as a parameter in the URL and attempts to send the corresponding image file using Flask’s send_file
function. If the image file is not found, it returns a 404 error with a message.
Python3
@api.route('/image/<string:image_name>')
class ImageDisplay(Resource):
def get(self, image_name):
image_path = os.path.join(image_store, image_name)
if os.path.exists(image_path):
return send_file(image_path, mimetype='image/jpeg')
else:
return {'message': 'Image not found'}, 404
if __name__ == '__main__':
app.run(debug=True)
Complete Code
Python3
from flask import Flask, request, send_file
from flask_restx import Api, Resource, reqparse
from werkzeug.datastructures import FileStorage
from PIL import Image
import os
app = Flask(__name__)
api = Api(app, version='1.0', title='Image Upload API',
description='API for uploading and displaying images')
upload_parser = api.parser()
upload_parser.add_argument('image', location='files',
type=FileStorage, required=True,
help='Image file')
image_store = "uploaded_images"
if not os.path.exists(image_store):
os.makedirs(image_store)
@api.route('/upload')
class ImageUpload(Resource):
@api.expect(upload_parser)
def post(self):
args = upload_parser.parse_args()
image_file = args['image']
image_path = os.path.join(image_store,
image_file.filename)
image_file.save(image_path)
# Resize image if needed
resized_path = os.path.join(image_store,
"resized_" + image_file.filename)
with Image.open(image_path) as img:
img.thumbnail((300, 300))
img.save(resized_path)
return {'message': 'Image uploaded successfully',
'image_path': resized_path}
@api.route('/image/<string:image_name>')
class ImageDisplay(Resource):
def get(self, image_name):
image_path = os.path.join(image_store, image_name)
if os.path.exists(image_path):
return send_file(image_path, mimetype='image/jpeg')
else:
return {'message': 'Image not found'}, 404
if __name__ == '__main__':
app.run(debug=True)
Output
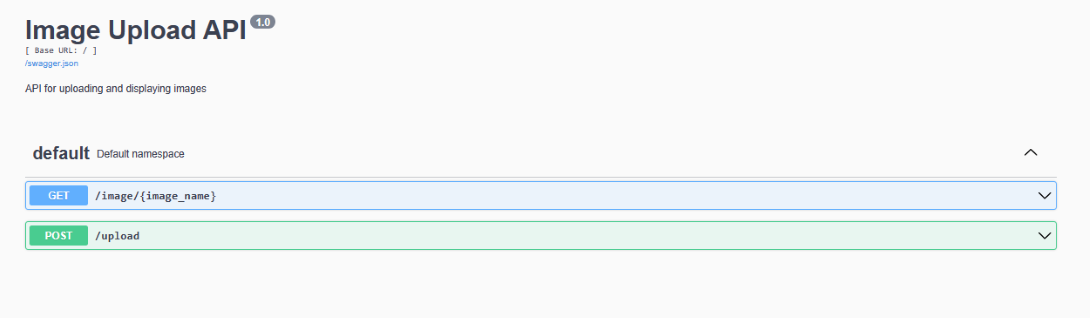
Video Demonstration
Share your thoughts in the comments
Please Login to comment...