Swagger UI
Last Updated :
06 Dec, 2023
Swagger UI is a powerful tool for documenting and testing RESTful APIs. It provides an interactive web-based interface that makes it easy for developers to understand and explore APIs. In this article, we will explore Swagger UI with the help of Python examples.
What is Swagger UI?
Swagger Ui is a part of the Swagger system, which provides a set of tools for designing, building, and documenting RESTful APIs. Swagger UI is specifically designed for API documentation. It generates a user-friendly, interactive documentation interface based on your API’s OpenAPI (formerly known as Swagger) specification.
Features of Swagger UI
- An interactive interface for API exploration and also for testing API’s.
- Clear and easy-to-read documentation.
- Built-in support for various API authentication methods.
- Automatic generation of API client SDKs.
- Integration with various programming languages and also with frameworks.
Use of Swagger UI
Swagger UI is a versatile tool that can be used for various use cases related to API development and documentation. Here are some common use cases for Swagger UI:
- API Documentation: Swagger UI is primarily used to create interactive and user-friendly documentation for your RESTful APIs. It allows developers, testers, and consumers to understand the endpoints, parameters, request and response structures, and authentication methods of an API
- API Testing: Swagger UI provides an interactive interface for testing APIs. Users can make real API requests and see the responses directly within the Swagger UI interface. This is useful for both API development and quality assurance.
- Integration with API Gateways: Swagger UI can be used in conjunction with API gateways and management tools. By providing a well-documented API through Swagger UI, API gateways can enforce policies, rate limits, and security measures while developers still have easy access to documentation.
Swagger UI Examples
Before we start, make sure we have Python installed on your system. We will also need the Flask and flask-restful
packages, which can be installed using pip:
pip install flask
pip install uvicorn
pip install flasgger
To run the code run below command
python main.py (python file name)
Example 1: Basic API Documentation
In this example, we will create a simple API and generate documentation using Swagger UI.
Python3
from flask import Flask
from flask_restful import Resource, Api
from flasgger import Swagger
app = Flask(__name__)
api = Api(app)
swagger = Swagger(app)
class HelloWorld(Resource):
def get( self ):
return { 'message' : 'Hello, World!' }
api.add_resource(HelloWorld, '/' )
if __name__ = = '__main__' :
app.run(debug = True )
|
Run the script and access Swagger UI at http://localhost:5000/apidocs
to explore the API documentation.
Output:
{'message': 'Hello, World!'}
Example 2: Documenting API Parameters
In this example, we’ll create an API with query parameters and document them using Swagger.
Python3
from flask import Flask, request
from flask_restful import Resource, Api
from flasgger import Swagger
app = Flask(__name__)
api = Api(app)
swagger = Swagger(app)
class GreetUser(Resource):
def get( self ):
name = request.args.get( 'name' )
return { 'message' : f 'Hello, {name}!' }
api.add_resource(GreetUser, '/greet' )
if __name__ = = '__main__' :
app.run(debug = True )
|
Run the script and access Swagger UI at http://localhost:5000/apidocs
to test the API with query parameters.
Output:
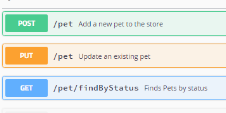
Output
Example 3: Documenting API Responses
In this example, we will create an API that returns a list of books and document the response format.
Python3
from flask import Flask
from flask_restful import Resource, Api
from flasgger import Swagger
app = Flask(__name__)
api = Api(app)
swagger = Swagger(app)
class Books(Resource):
def get( self ):
books = [
{ 'title' : 'Book 1' , 'author' : 'Author 1' },
{ 'title' : 'Book 2' , 'author' : 'Author 2' },
]
return books
api.add_resource(Books, '/books' )
if __name__ = = '__main__' :
app.run(debug = True )
|
Run the script and access Swagger UI at http://localhost:5000/apidocs
to explore the API documentation with response schema. and test API by taking any query and parameter your convenience.
Output
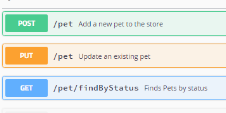
Output
Video Output
Conclusion
Swagger UI is a valuable tool for documenting and testing your RESTful APIs in Python. It provides a user-friendly interface for both developers and consumers to understand and interact with your APIs effectively. By following the examples above, you can easily integrate Swagger UI into your Python API projects and create comprehensive API documentation.
Share your thoughts in the comments
Please Login to comment...