Design First API Development with Swagger
Last Updated :
05 Feb, 2024
API development plays a crucial role in modern software architecture, enabling different services to communicate with each other seamlessly. One popular approach to API development is the “Design-First” methodology, where the API’s design is specified before the actual implementation. Swagger, now known as OpenAPI, is a powerful tool that facilitates Design-First API development by providing a standardized way to describe RESTful APIs.
What is Swagger?
Swagger, now part of the OpenAPI Initiative, is a specification for building APIs. It allows developers to define API endpoints, request/response formats, and various other details in a machine-readable format. With Swagger, you can design, document, and test your APIs before writing any code, ensuring a well-thought-out and user-friendly interface.
Design-First API Development with Swagger
Design-first API development involves creating a detailed API specification before implementing the actual code. This approach helps in identifying potential issues, defining clear communication contracts, and streamlining the development process. Swagger provides tools to follow this methodology effectively.
Let’s go through the steps of design-first API development using Swagger in Python
Step 1: Install Required Packages
To get started, you need to install the necessary Python packages. The primary tool we’ll be using is Swagger, which is a Python framework that automates the generation of Swagger documentation, and also the flask, flask_restx which we will use for creating the Swagger API.
pip install swagger
pip install flask
pip install flaks_restx
Step 2: Import Necessary Library
To get started, you need to import the necessary Python packages. The primary tool we’ll be using is swagger, which is a Python framework that automates the generation of Swagger documentation, and also the flask , flask_restx which we will use for creating the swagger API.
Python3
from flask import Flask, request
from flask_restx import Api, Resource, fields
|
Step 3: Create CRUD API using Swagger
In this example, below code defines a simple CRUD API using Flask and Flask-RESTx. It creates a Flask application with two API endpoints: /data for listing and creating data, and /data/<data_id> for getting, updating, and deleting data by ID. The API uses Swagger documentation to describe the data model, including fields like ‘id’, ‘name’, and ‘value’. The sample data is stored in-memory as a list of dictionaries. Each API endpoint is implemented as a class with methods corresponding to CRUD operations. The application runs on http://localhost:5000, and you can interact with the API using the Swagger UI at http://localhost:5000/swagger.
Python3
from flask import Flask, request
from flask_restx import Api, Resource, fields
app = Flask(__name__)
api = Api(app, version = '1.0' , title = 'CRUD API' , description = 'A simple CRUD API' )
data_model = api.model( 'Data' , {
'id' : fields.Integer(readOnly = True , description = 'The unique identifier' ),
'name' : fields.String(required = True , description = 'The name of the data' ),
'value' : fields.String(required = True , description = 'The value of the data' ),
})
sample_data = [
{ 'id' : 1 , 'name' : 'Data 1' , 'value' : 'Value 1' },
{ 'id' : 2 , 'name' : 'Data 2' , 'value' : 'Value 2' },
]
@api .route( '/data' )
class DataList(Resource):
@api .doc( 'list_data' )
def get( self ):
return sample_data
@api .doc( 'create_data' )
@api .expect(data_model)
def post( self ):
new_data = request.json
new_data[ 'id' ] = len (sample_data) + 1
sample_data.append(new_data)
return new_data, 201
@api .route( '/data/<int:data_id>' )
@api .response( 404 , 'Data not found' )
@api .param( 'data_id' , 'The data identifier' )
class Data(Resource):
@api .doc( 'get_data' )
def get( self , data_id):
data = next ((item for item in sample_data if item[ 'id' ] = = data_id), None )
if data is None :
api.abort( 404 , "Data not found" )
return data
@api .doc( 'update_data' )
@api .expect(data_model)
def put( self , data_id):
data = next ((item for item in sample_data if item[ 'id' ] = = data_id), None )
if data is None :
api.abort( 404 , "Data not found" )
updated_data = request.json
data.update(updated_data)
return data
@api .doc( 'delete_data' )
def delete( self , data_id):
global sample_data
sample_data = [item for item in sample_data if item[ 'id' ] ! = data_id]
return { 'message' : 'Data deleted successfully' }, 200
if __name__ = = '__main__' :
app.run(debug = True )
|
Step 4: Run Your API
Execute the following command in your terminal to run your API
http://127.0.0.1:5000/
Output:
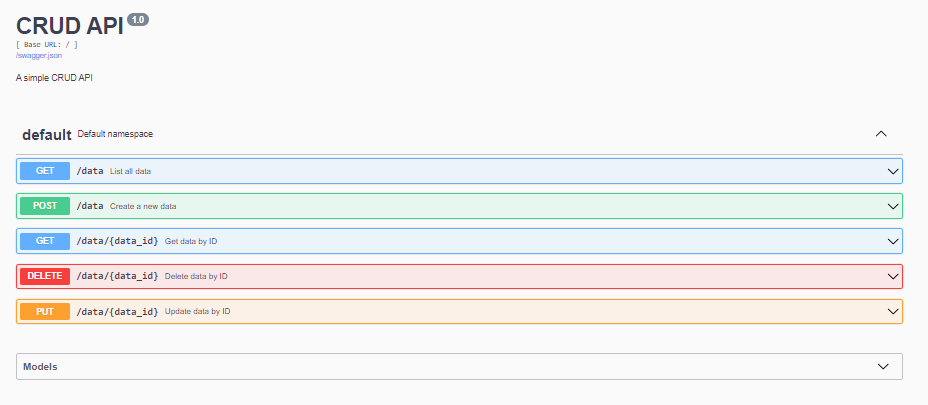
Video Demonstration
Conclusion
Design-first API development with Swagger in Python provides a structured and efficient way to design, document, and test APIs. By creating a clear specification upfront, you ensure better communication between teams and reduce the likelihood of issues during implementation. Swagger’s integration with Python through tools like connexion makes this process seamless and developer-friendly. Embrace design-first practices to build robust and well-documented APIs with Swagger in Python.
Share your thoughts in the comments
Please Login to comment...