Sort a linked list of 0s, 1s and 2s
Last Updated :
20 Mar, 2023
Given a linked list of 0s, 1s and 2s, The task is to sort and print it.
Examples:
Input: 1 -> 1 -> 2 -> 0 -> 2 -> 0 -> 1 -> NULL
Output: 0 -> 0 -> 1 -> 1 -> 1 -> 2 -> 2 -> NULL
Input: 1 -> 1 -> 2 -> 1 -> 0 -> NULL
Output: 0 -> 1 -> 1 -> 1 -> 2 -> NULL
Source: Microsoft Interview | Set 1
Sort Linked List of 0s, 1s and 2s using frequency counting:
Follow the below steps to implement the idea:
- Traverse the list and count the number of 0s, 1s, and 2s. Let the counts be n1, n2, and n3 respectively.
- Traverse the list again, fill the first n1 nodes with 0, then n2 nodes with 1, and finally n3 nodes with 2.
Below image is a dry run of the above approach:
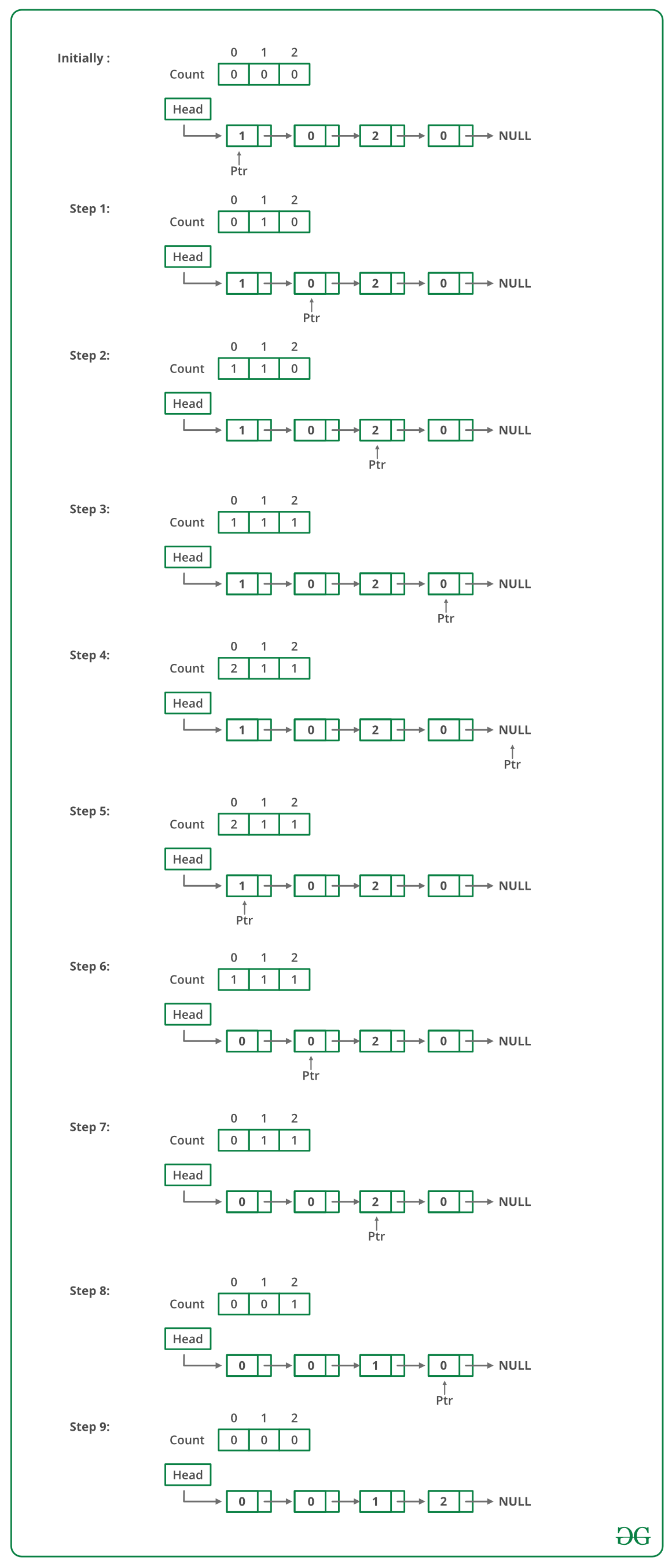
Below is the implementation of the above approach.
C++
#include <bits/stdc++.h>
using namespace std;
class Node
{
public :
int data;
Node* next;
};
void sortList(Node *head)
{
int count[3] = {0, 0, 0};
Node *ptr = head;
while (ptr != NULL)
{
count[ptr->data] += 1;
ptr = ptr->next;
}
int i = 0;
ptr = head;
while (ptr != NULL)
{
if (count[i] == 0)
++i;
else
{
ptr->data = i;
--count[i];
ptr = ptr->next;
}
}
}
void push (Node** head_ref, int new_data)
{
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList(Node *node)
{
while (node != NULL)
{
cout << node->data << " " ;
node = node->next;
}
cout << endl;
}
int main( void )
{
Node *head = NULL;
push(&head, 0);
push(&head, 1);
push(&head, 0);
push(&head, 2);
push(&head, 1);
push(&head, 1);
push(&head, 2);
push(&head, 1);
push(&head, 2);
cout << "Linked List before Sorting\n" ;
printList(head);
sortList(head);
cout << "Linked List after Sorting\n" ;
printList(head);
return 0;
}
|
C
#include<stdio.h>
#include<stdlib.h>
struct Node
{
int data;
struct Node* next;
};
void sortList( struct Node *head)
{
int count[3] = {0, 0, 0};
struct Node *ptr = head;
while (ptr != NULL)
{
count[ptr->data] += 1;
ptr = ptr->next;
}
int i = 0;
ptr = head;
while (ptr != NULL)
{
if (count[i] == 0)
++i;
else
{
ptr->data = i;
--count[i];
ptr = ptr->next;
}
}
}
void push ( struct Node** head_ref, int new_data)
{
struct Node* new_node =
( struct Node*) malloc ( sizeof ( struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList( struct Node *node)
{
while (node != NULL)
{
printf ( "%d " , node->data);
node = node->next;
}
printf ( "\n" );
}
int main( void )
{
struct Node *head = NULL;
push(&head, 0);
push(&head, 1);
push(&head, 0);
push(&head, 2);
push(&head, 1);
push(&head, 1);
push(&head, 2);
push(&head, 1);
push(&head, 2);
printf ( "Linked List before Sorting \n" );
printList(head);
sortList(head);
printf ( "Linked List after Sorting \n" );
printList(head);
return 0;
}
|
Java
class LinkedList
{
Node head;
class Node
{
int data;
Node next;
Node( int d) {data = d; next = null ; }
}
void sortList()
{
int count[] = { 0 , 0 , 0 };
Node ptr = head;
while (ptr != null )
{
count[ptr.data]++;
ptr = ptr.next;
}
int i = 0 ;
ptr = head;
while (ptr != null )
{
if (count[i] == 0 )
i++;
else
{
ptr.data= i;
--count[i];
ptr = ptr.next;
}
}
}
public void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null )
{
System.out.print(temp.data+ " " );
temp = temp.next;
}
System.out.println();
}
public static void main(String args[])
{
LinkedList llist = new LinkedList();
llist.push( 0 );
llist.push( 1 );
llist.push( 0 );
llist.push( 2 );
llist.push( 1 );
llist.push( 1 );
llist.push( 2 );
llist.push( 1 );
llist.push( 2 );
System.out.println( "Linked List before sorting" );
llist.printList();
llist.sortList();
System.out.println( "Linked List after sorting" );
llist.printList();
}
}
|
Python3
class LinkedList( object ):
def __init__( self ):
self .head = None
class Node( object ):
def __init__( self , d):
self .data = d
self . next = None
def sortList( self ):
count = [ 0 , 0 , 0 ]
ptr = self .head
while ptr ! = None :
count[ptr.data] + = 1
ptr = ptr. next
i = 0
ptr = self .head
while ptr ! = None :
if count[i] = = 0 :
i + = 1
else :
ptr.data = i
count[i] - = 1
ptr = ptr. next
def push( self , new_data):
new_node = self .Node(new_data)
new_node. next = self .head
self .head = new_node
def printList( self ):
temp = self .head
while temp ! = None :
print ( str (temp.data),end = " " )
temp = temp. next
print ()
llist = LinkedList()
llist.push( 0 )
llist.push( 1 )
llist.push( 0 )
llist.push( 2 )
llist.push( 1 )
llist.push( 1 )
llist.push( 2 )
llist.push( 1 )
llist.push( 2 )
print ( "Linked List before sorting" )
llist.printList()
llist.sortList()
print ( "Linked List after sorting" )
llist.printList()
|
C#
using System;
public class LinkedList
{
Node head;
class Node
{
public int data;
public Node next;
public Node( int d)
{
data = d; next = null ;
}
}
void sortList()
{
int []count = {0, 0, 0};
Node ptr = head;
while (ptr != null )
{
count[ptr.data]++;
ptr = ptr.next;
}
int i = 0;
ptr = head;
while (ptr != null )
{
if (count[i] == 0)
i++;
else
{
ptr.data= i;
--count[i];
ptr = ptr.next;
}
}
}
public void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null )
{
Console.Write(temp.data+ " " );
temp = temp.next;
}
Console.WriteLine();
}
public static void Main(String []args)
{
LinkedList llist = new LinkedList();
llist.push(0);
llist.push(1);
llist.push(0);
llist.push(2);
llist.push(1);
llist.push(1);
llist.push(2);
llist.push(1);
llist.push(2);
Console.WriteLine( "Linked List before sorting" );
llist.printList();
llist.sortList();
Console.WriteLine( "Linked List after sorting" );
llist.printList();
}
}
|
Javascript
<script>
var head;
class Node {
constructor(val) {
this .data = val;
this .next = null ;
}
}
function sortList() {
var count = [ 0, 0, 0 ];
var ptr = head;
while (ptr != null ) {
count[ptr.data]++;
ptr = ptr.next;
}
var i = 0;
ptr = head;
while (ptr != null ) {
if (count[i] == 0)
i++;
else {
ptr.data = i;
--count[i];
ptr = ptr.next;
}
}
}
function push(new_data) {
var new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
function printList() {
var temp = head;
while (temp != null ) {
document.write(temp.data + " " );
temp = temp.next;
}
document.write( "<br/>" );
}
push(0);
push(1);
push(0);
push(2);
push(1);
push(1);
push(2);
push(1);
push(2);
document.write( "Linked List before sorting<br/>" );
printList();
sortList();
document.write( "Linked List after sorting<br/>" );
printList();
</script>
|
Output
Linked List before Sorting
2 1 2 1 1 2 0 1 0
Linked List after Sorting
0 0 1 1 1 1 2 2 2
Time Complexity: O(n) where n is the number of nodes in the linked list.
Auxiliary Space: O(1)
Sort a linked list of 0s, 1s and 2s by changing links
Using a stable sort algorithm:
C++
#include <iostream>
struct Node {
int data;
Node *next;
};
void push(Node **head, int data) {
Node *newNode = new Node();
newNode->data = data;
newNode->next = nullptr;
if (*head == nullptr) {
*head = newNode;
return ;
}
Node *last = *head;
while (last->next != nullptr) {
last = last->next;
}
last->next = newNode;
}
void printList(Node *head) {
Node *current = head;
while (current != nullptr) {
std::cout << current->data << " " ;
current = current->next;
}
std::cout << std::endl;
}
void sortList(Node *head) {
int count[3] = {0, 0, 0};
Node *current = head;
while (current != nullptr) {
count[current->data]++;
current = current->next;
}
current = head;
int i = 0;
while (current != nullptr) {
if (count[i] == 0) {
i++;
} else {
current->data = i;
count[i]--;
current = current->next;
}
}
}
int main() {
Node *head = nullptr;
push(&head, 0);
push(&head, 1);
push(&head, 0);
push(&head, 2);
push(&head, 1);
push(&head, 1);
push(&head, 2);
push(&head, 1);
push(&head, 2);
std::cout << "Linked List before Sorting: " <<std::endl;
printList(head);
sortList(head);
std::cout << "Linked List after Sorting: " <<std::endl;
printList(head);
return 0;
}
|
Java
import java.util.*;
public class Main {
static class Node {
int data;
Node next;
Node( int data)
{
this .data = data;
this .next = null ;
}
}
static Node push(Node head, int data)
{
Node newNode = new Node(data);
if (head == null ) {
head = newNode;
return head;
}
Node last = head;
while (last.next != null ) {
last = last.next;
}
last.next = newNode;
return head;
}
static void printList(Node head)
{
Node current = head;
while (current != null ) {
System.out.print(current.data + " " );
current = current.next;
}
System.out.println();
}
static void sortList(Node head)
{
int [] count = { 0 , 0 , 0 };
Node current = head;
while (current != null ) {
count[current.data]++;
current = current.next;
}
current = head;
int i = 0 ;
while (current != null ) {
if (count[i] == 0 ) {
i++;
}
else {
current.data = i;
count[i]--;
current = current.next;
}
}
}
public static void main(String[] args)
{
Node head = null ;
head = push(head, 0 );
head = push(head, 1 );
head = push(head, 0 );
head = push(head, 2 );
head = push(head, 1 );
head = push(head, 1 );
head = push(head, 2 );
head = push(head, 1 );
head = push(head, 2 );
System.out.println( "Linked List before Sorting: " );
printList(head);
sortList(head);
System.out.println( "Linked List after Sorting: " );
printList(head);
}
}
|
Python3
class Node:
def __init__( self , data):
self .data = data
self . next = None
def push(head, data):
new_node = Node(data)
if head is None :
head = new_node
return head
last = head
while last. next is not None :
last = last. next
last. next = new_node
return head
def printList(head):
current = head
while current is not None :
print (current.data, end = ' ' )
current = current. next
print ()
def sortList(head):
count = [ 0 , 0 , 0 ]
current = head
while current is not None :
count[current.data] + = 1
current = current. next
current = head
i = 0
while current is not None :
if count[i] = = 0 :
i + = 1
else :
current.data = i
count[i] - = 1
current = current. next
if __name__ = = '__main__' :
head = None
head = push(head, 0 )
head = push(head, 1 )
head = push(head, 0 )
head = push(head, 2 )
head = push(head, 1 )
head = push(head, 1 )
head = push(head, 2 )
head = push(head, 1 )
head = push(head, 2 )
print ( "Linked List before Sorting:" )
printList(head)
sortList(head)
print ( "Linked List after Sorting:" )
printList(head)
|
C#
using System;
class Gfg{
public class Node {
public int data;
public Node next;
}
public static void Push( ref Node head, int data) {
Node newNode = new Node();
newNode.data = data;
newNode.next = null ;
if (head == null ) {
head = newNode;
return ;
}
Node last = head;
while (last.next != null ) {
last = last.next;
}
last.next = newNode;
}
public static void PrintList(Node head) {
Node current = head;
while (current != null ) {
Console.Write(current.data + " " );
current = current.next;
}
Console.WriteLine();
}
public static void SortList(Node head) {
int [] count = {0, 0, 0};
Node current = head;
while (current != null ) {
count[current.data]++;
current = current.next;
}
current = head;
int i = 0;
while (current != null ) {
if (count[i] == 0) {
i++;
} else {
current.data = i;
count[i]--;
current = current.next;
}
}
}
public static void Main() {
Node head = null ;
Push( ref head, 0);
Push( ref head, 1);
Push( ref head, 0);
Push( ref head, 2);
Push( ref head, 1);
Push( ref head, 1);
Push( ref head, 2);
Push( ref head, 1);
Push( ref head, 2);
Console.WriteLine( "Linked List before Sorting:" );
PrintList(head);
SortList(head);
Console.WriteLine( "Linked List after Sorting:" );
PrintList(head);
}
}
|
Javascript
class Node {
constructor(data) {
this .data = data;
this .next = null ;
}
}
function push(head, data) {
const new_node = new Node(data);
if (head === null ) {
head = new_node;
return head;
}
let last = head;
while (last.next !== null ) {
last = last.next;
}
last.next = new_node;
return head;
}
function printList(head) {
let current = head;temp= "" ;
while (current !== null ) {
temp = temp + current.data+ ' ' ;
current = current.next;
}
console.log(temp);
}
function sortList(head) {
const count = [0, 0, 0];
let current = head;
while (current !== null ) {
count[current.data] += 1;
current = current.next;
}
current = head;
let i = 0;
while (current !== null ) {
if (count[i] === 0) {
i += 1;
} else {
current.data = i;
count[i] -= 1;
current = current.next;
}
}
}
let head = null ;
head = push(head, 0);
head = push(head, 1);
head = push(head, 0);
head = push(head, 2);
head = push(head, 1);
head = push(head, 1);
head = push(head, 2);
head = push(head, 1);
head = push(head, 2);
console.log( "Linked List before Sorting:" );
printList(head);
sortList(head);
console.log( "Linked List after Sorting:" );
printList(head);
|
Output
Linked List before Sorting:
0 1 0 2 1 1 2 1 2
Linked List after Sorting:
0 0 1 1 1 1 2 2 2
Explanation of the above approach:
- The Node structure: This structure is used to define a node in a linked list. It contains an integer data to store the data of the node and a pointer next to the next node in the linked list.
- The push function: This function is used to insert a new node at the end of the linked list. It takes two parameters: a pointer to the head of the linked list and an integer data to be stored in the new node. The function first creates a new node with the given data and sets its next pointer to nullptr. If the linked list is empty, the function sets the head of the linked list to the new node. Otherwise, it iterates through the linked list to find the last node and inserts the new node after it.
- The printList function: This function is used to print the linked list. It takes one parameter, a pointer to the head of the linked list, and iterates through the list while printing the data of each node.
- The sortList function: This function is used to sort the linked list. It takes one parameter, a pointer to the head of the linked list. The function first initializes an array count of size 3 to store the count of 0’s, 1’s, and 2’s in the linked list. It then iterates through the linked list and increments the count of each element as it appears. Finally, it overwrites the linked list with the sorted elements. The function iterates through the linked list again and whenever it encounters a node with a value of 0, it sets its data to 0 and decrements the count of 0’s. Similarly, whenever it encounters a node with a value of 1, it sets its data to 1 and decrements the count of 1’s. And whenever it encounters a node with a value of 2, it sets its data to 2 and decrements the count of 2’s.
- The main function: This function is the entry point of the program. It first creates an empty linked list and inserts some elements into it. It then calls the sortList function to sort the linked list. Finally, it prints the original and sorted linked lists to verify that the sorting is correct.
Note: This is a simple implementation of the “Using a stable sort algorithm” approach for sorting a linked list containing only 0’s, 1’s, and 2’s. It may not be the most efficient implementation for large linked lists, but it should give you a good understanding of the basic idea
Time complexity: O(n),
The time complexity of this implementation is O(n), where n is the number of elements in the linked list. This is because the program iterates through the linked list twice: once to count the number of 0’s, 1’s, and 2’s, and once to overwrite the linked list with the sorted elements. The count operation takes O(n) time and the overwrite operation takes O(n) time, so the total time complexity is O(n) + O(n) = O(n).
Space complexity: O(1),
The space complexity of this implementation is O(1), because it uses only a constant amount of extra memory to store the count array of size 3. The linked list itself is not used to store any additional information during the sorting, so its space complexity remains the same.
Share your thoughts in the comments
Please Login to comment...