Selenium Handling Radio Buttons
Last Updated :
06 Dec, 2023
Selenium is one of the most popular and widely used tools when it comes to automating web applications and testing web applications. Selenium allows developers and QA testers to automate certain tasks in web applications like filling out forms navigating to a certain page or testing if the functions are working as expected or not. Selenium helps in conducting automation tasks and automating web applications easily.
What is a Radio Button?
One of the most common tasks while interacting with the web application is handling radio buttons. Radio Buttons are the elements on the web page that allow the users to select a select option from a list and allow the users to select an option from the list of options.
Why are radio Buttons used?
Radio buttons are used to provide choices to the user, allowing them to pick a choice from the set of choices. For example, a user may be asked to state their gender from the list of genders, and he has to select his gender from the list of genders.
There are three steps to handle a Radio Button in Selenium they are-
- Locate a Radio Button.
- Check if the Radio Button is selected or not.
- Select a Radio Button.
To interact and handle a Radio Button we first have to find and locate a radio button on a web page. So In this section, I’ll show you a step-by-step guide to locate a Radio Button in Selenium.
Step 1: Installing the required Libraries:
Make sure to have Python installed in our system then You can install Selenium using the following command-
pip install selenium
Step 2: Import the Necessary modules.
We need to import the WebDriver, By, and NoSuchElementException from Selenium:
- WebDriver– Web Driver provides a way to interact with the browser programmatically. It acts as a bridge between your automation script and the browser you want to automate.
- By– By is a class that provides methods a way to locate the element on a web page using various locators.
- NoSuchElementException -NoSuchElementException is a common exception which occurs when selenium is unable to locate an element on a web page using the specified locator.
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
|
Step 3: Initializing the Web Driver and Navigating to the web page.
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
|
Explanation
- webDriver.Chrome()– It is used to set up the web Driver and Select chrome as out automation Browser.
- driver.get(url)– driver.get() is used to open the Chrome Browser and navigate to the given url.
Step 6: Selecting a Locating Strategy:
Now in order to locate a radio button on a web page we first need to select a locator which can uniquely identify that element on a web page. Selenium provides various types of Locators which we can use to find an element. They are-
- ID- ID locators are used when the target element has ID as a unique ID attribute. It is the fastest and most reliable locating strategy.
- NAME- Name Locators are used when the target element has name attribute which is unique among the other form elements.
- CLASS_NAME- CLASS_NAME locator is used when the target element which is unique among the other elements.
- TAG_NAME- TAG_NAME locators are used when you want to select all the element of a particular tag type.
- XPATH- XPath are one of the most powerful and reliable locating strategy used when we are unable to find a suitable identifier in a web page for locating.
- LINK_TEXT and Partial Link Texts- Link Text and partial link Texts locators are used for anchor elements when the anchor element has a unique text among the other elements.
- CSS Selectors- CSS Selects are another powerful and reliable locating strategy which we can use to use to locate the target element using CSS selectors.
To know more about Locating Strategies refer to Locating Strategies in Selenium:
Step 7: Locating the radio button.
As we can see in the inspect element of the web page the radio button has a name of “q1” which in unique among all the other elements, so we’ll use Name as Locating Strategy. Along with Locator we’ll use find_element() method in Selenium to locate an element on a web page.
find_element() is a method in Selenium which is used to locate an element on a web page using the specified locator. It returns the element if found else throws an NoSuchElementFound Exception, so we’ll try except block to handle the case when the element is not found.
Python
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
radioButton = driver.find_element(By.NAME, "q1" )
except :
print ( "Not Found" )
else :
print ( "Found" )
|
Output:
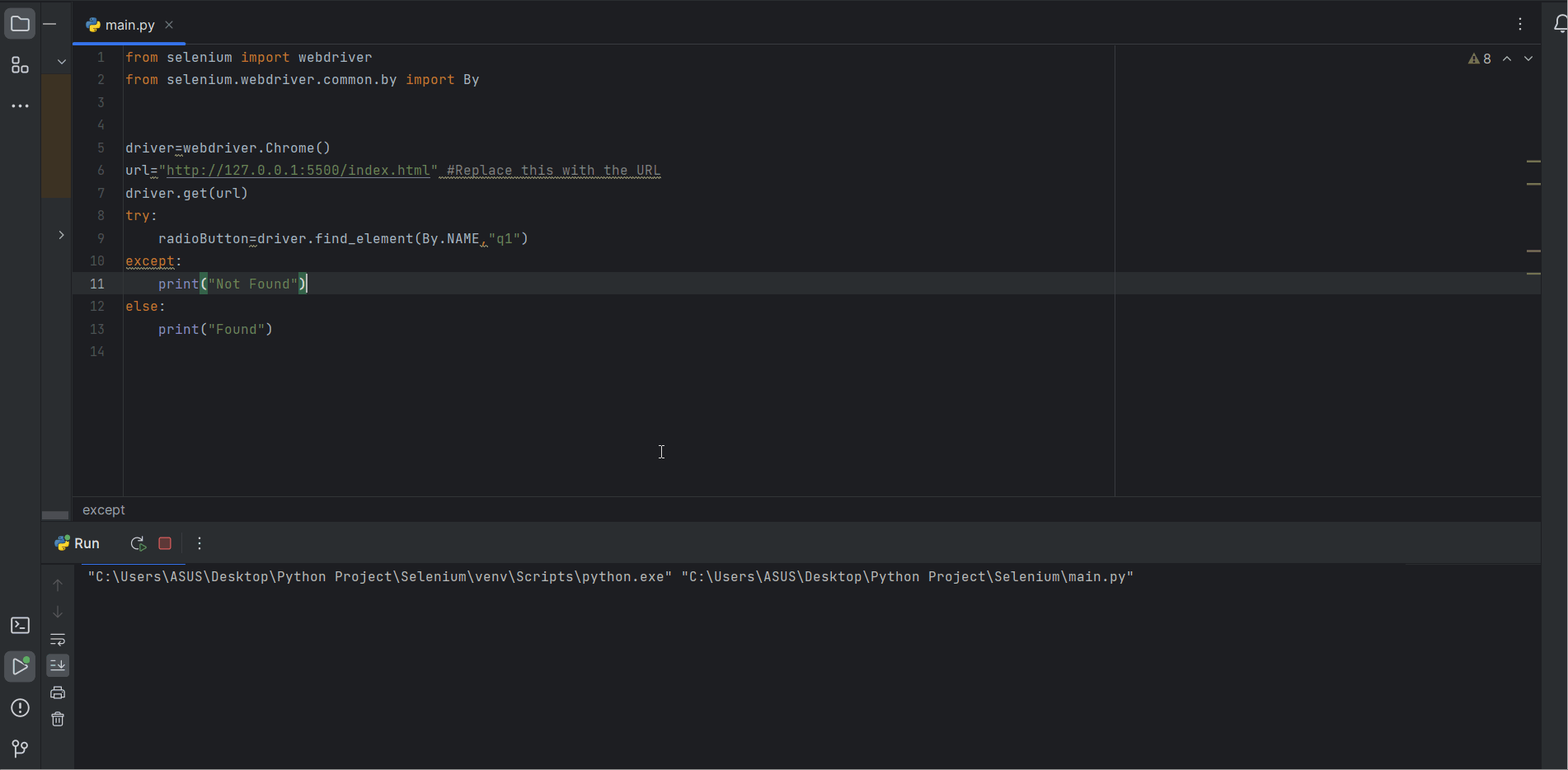
Selenium Handling Radio Buttons
Once we have successfully located the radio button we now need to check if the radio button is Selected or not.
Selenium has special methods to check if the radio button is selected or not, they are-
- is_selected()- is_selected() is a method in Selenium used to check if an element is selected or not. It returns a Boolean value.
Python
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
radioButton = driver.find_element(By.NAME, "q1" )
except :
print ( "Not Found" )
else :
if (radioButton.is_selected()):
print ( "element is already selected" )
else :
print ( "element is not selected" )
|
Output:
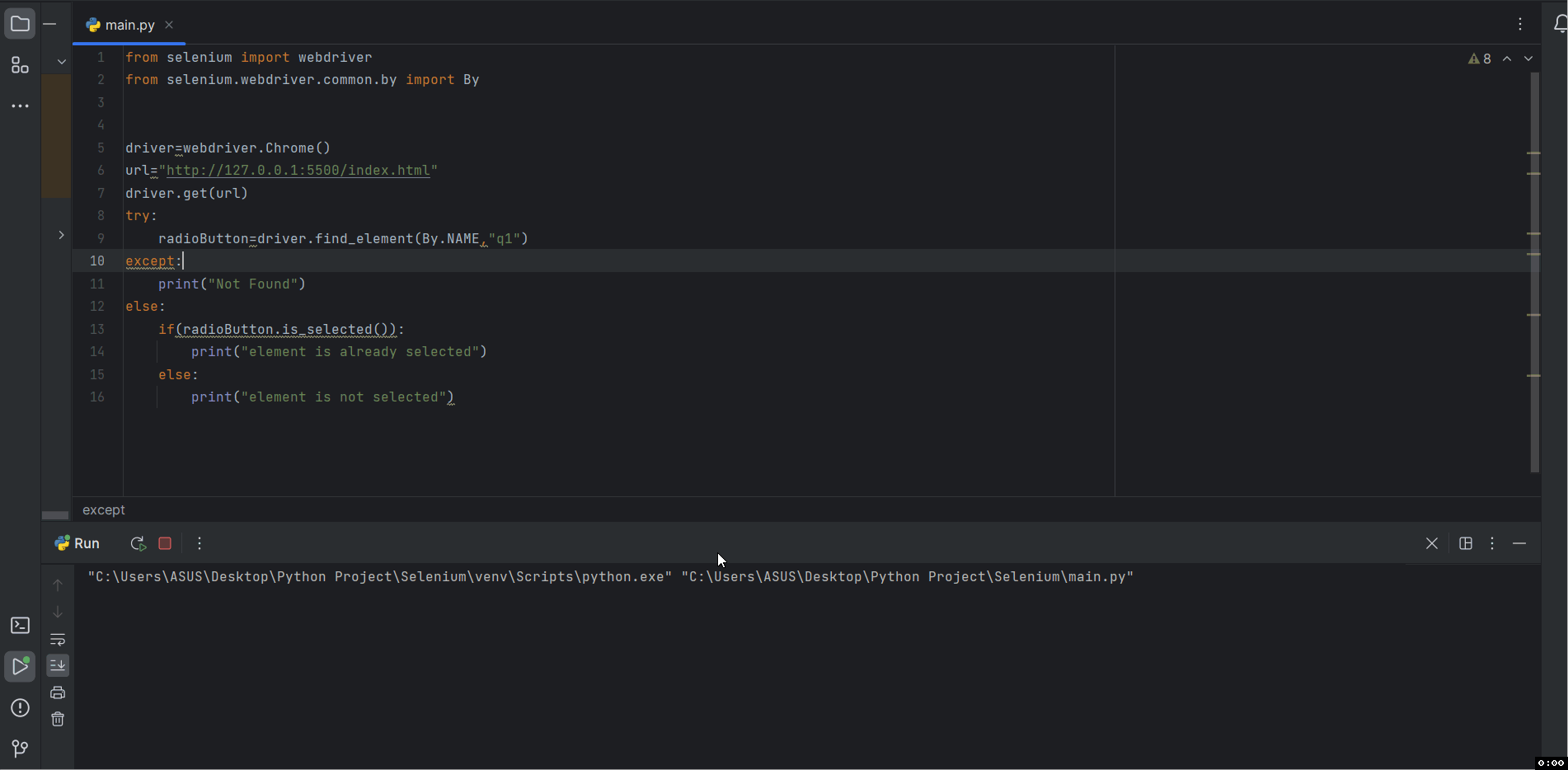
Selenium Handling Radio Buttons
As the element was not selected by default so it prints “element is not selected“
Before interacting with the Radio button make sure to follow the above steps correctly of Locating an element on a webpage and validating the radio Button. Now that we have done with the locating and validating the radio button, we can now Select a radio button according to out need . Selenium has click() method which is used to click on an element on a web page to select it.
Python
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
radioButton = driver.find_element(By.NAME, "q1" )
except :
print ( "Not Found" )
else :
if (radioButton.is_selected()):
print ( "element is already selected" )
else :
radioButton.click()
print ( "Radio Button Selected" )
|
Output:
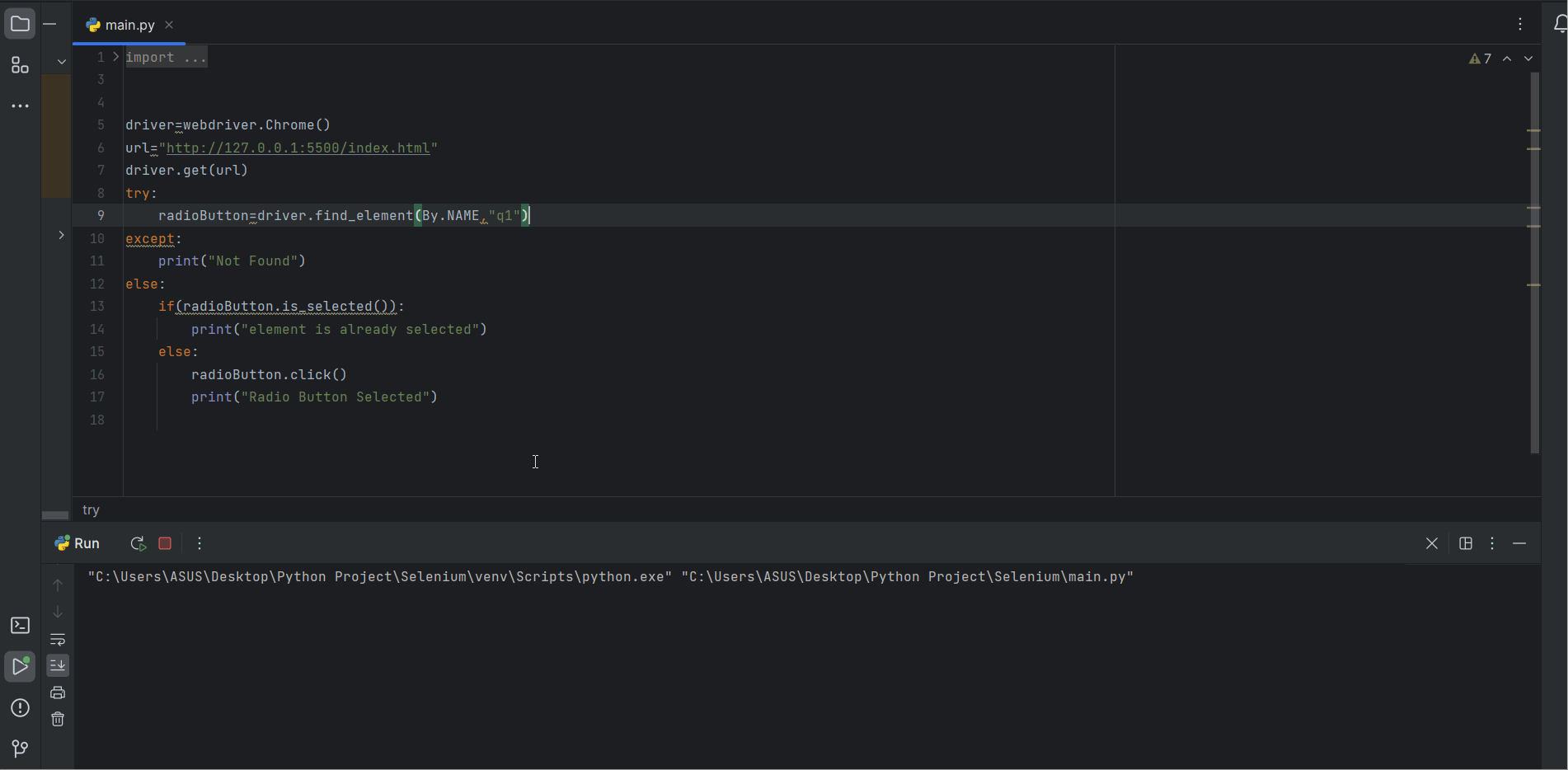
Selenium Handling Radio Buttons
Working with Radio Buttons Groups:
While we are dealing with radio buttons its very common to come across a radio button groups in a web form. Radio Button groups are a group or a set of radio buttons in which we can select only one radio Button and all the radio buttons within a same group have a common name attribute to group the radio buttons. So In this section I’ll show you a step by step tutorial on how to Work with Radio Buttons Group.
Step 1: Installing the required Libraries (Similar to Step 1 of How to Locate Radio Button)
Make sure that you have python installed in your system then you can install Selenium using the following command.
pip install selenium
Step 2: Importing the Necessary Modules (Similar to Step 2 of How to Locate Radio Button)
We need to import WebDriver, By, and NoSuchException
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
|
Step 3: Initializing the web Driver and Navigating to the Web Page (Similar to Step 3 of How to Locate Radio Button)
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
|
Step 4: Selecting a Locating Strategy
Now in order to locate a radio button on a web page we first need to select a locator which can uniquely identify that element on a web page. Selenium provides various types of Locators which we can use to find a element. They are-
- ID– ID locators are used when the target element has ID as a unique ID attribute. It is the fastest and most reliable locating strategy.
- NAME– Name Locators are used when the target element has name attribute which is unique among the other form elements.
- CLASS_NAME– CLASS_NAME locator is used when the target element which is unique among the other elements.
- TAG_NAME– TAG_NAME locators are used when you want to select all the element of a particular tag type.
- XPATH– XPath are one of the most powerful and reliable locating strategy used when we are unable to find a suitable identifier in a web page for locating.
- LINK_TEXT and Partial Link Texts– Link Text and partial link Texts locators are used for anchor elements when the anchor element has a unique text among the other elements.
- CSS Selectors- CSS Selects are another powerful and reliable locating strategy which we can use to use to locate the target element using CSS selectors.
To know more about Locating Strategies refer to Locating Strategies in Selenium
Step 5: Locating a Radio Button Group
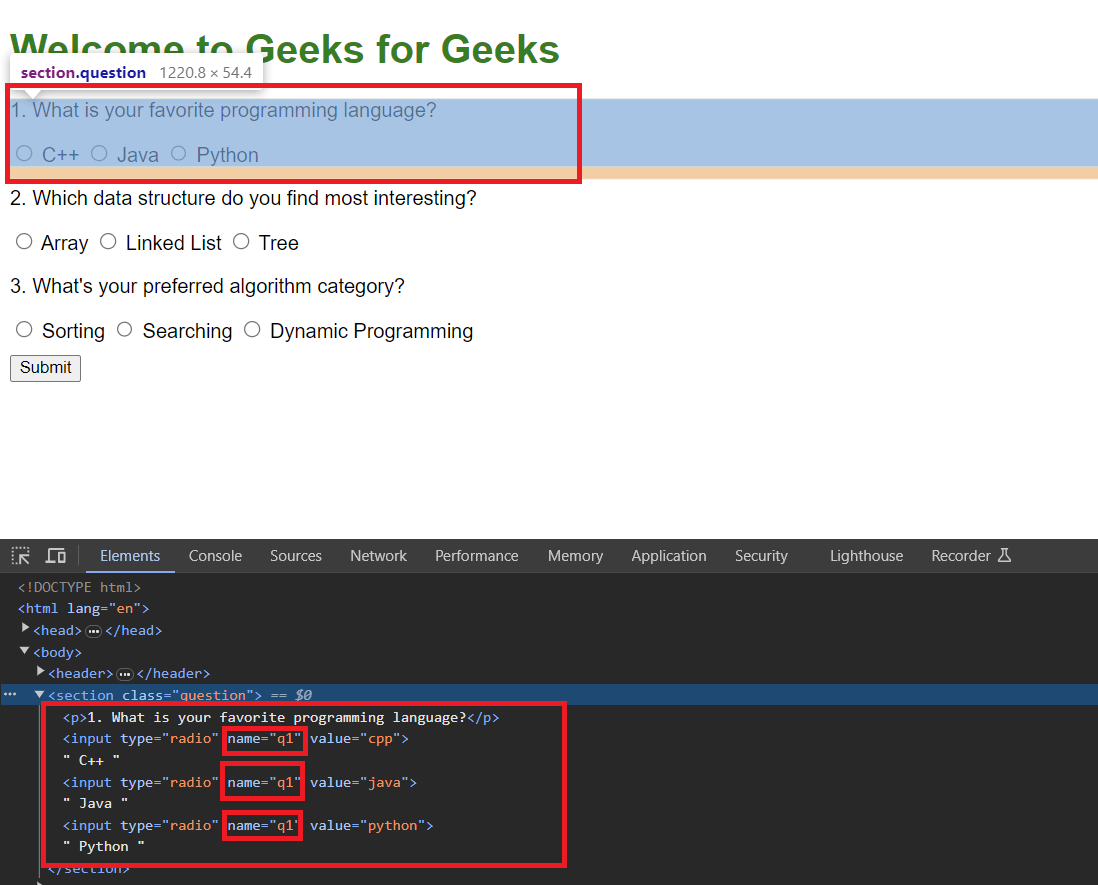
As the name attribute is common for all the elements in a radio button group and its unique for every radio button group so this makes the Name locator as our ideal choice, but you can go other locators as well. In order to locate multiple elements, we’ll have to use another method known as find_elements().
- find_elements()- find_elements() is a similar to find_element but intead of return only the first element which matches, find_elements returns a list of elements which matches the given condition. It is used for finding multiple elements on a web page like finding all radio button in a group etc. find_elements() return the list of all the element that matches the given condition.
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
radioButtons = driver.find_elements(By.NAME, "q1" )
except NoSuchElementException:
print ( "Not Found" )
else :
for button in radioButtons:
print (button.get_attribute( 'value' ))
|
Output:
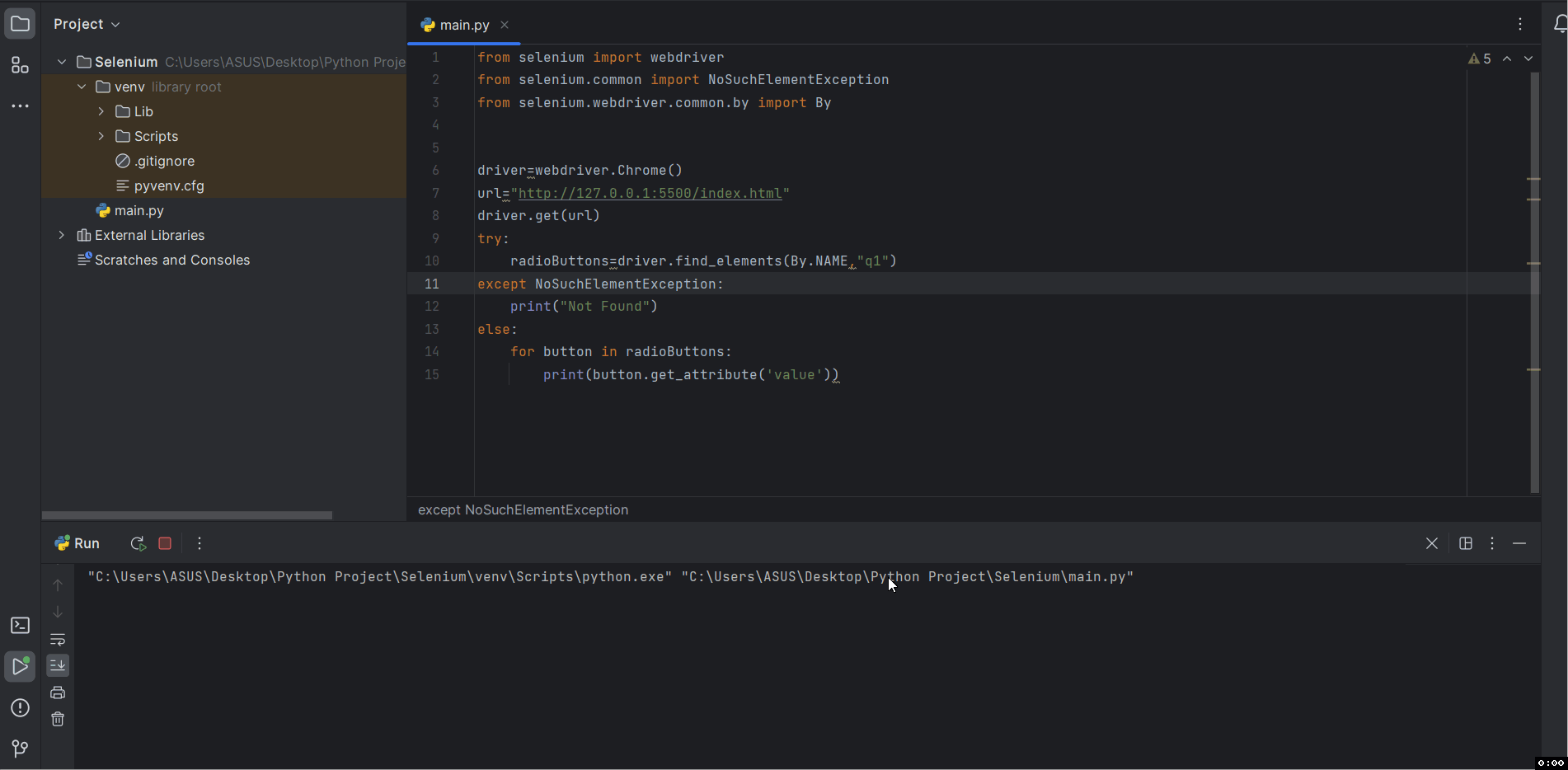
Selenium Handling Radio Buttons
Explanation–
Here we have used find_elements() to get a list of the radio buttons in the group. We have used try and except block to handle the NoSuchElementExcept along with the else block which means if the program gets executed without getting error the the else block will be excuted. In the else block we have run a for Each loop for all the radio Buttons in the list to get its value. button.get_attribute(‘value’) returns the attribute of the html element such as value attributes contains the text in the radio button.
Step 5: Selecting a radio Button from the group.
In order to select a radio button from the list we’ll have to use.click() method which is used to click on the target element to select the radio button. We’ll also use a get_attribute(‘value’) to get the value of the radio button along with a if else block to click on the element we want to select.
For example: Suppose we have to select the java radio button then
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
radioButtons = driver.find_elements(By.NAME, "q1" )
except NoSuchElementException:
print ( "Not Found" )
else :
target_option = "java"
for button in radioButtons:
if (button.get_attribute( 'value' ) = = target_option):
button.click()
print (f "{button.get_attribute('value')} is selected" )
|
Output:
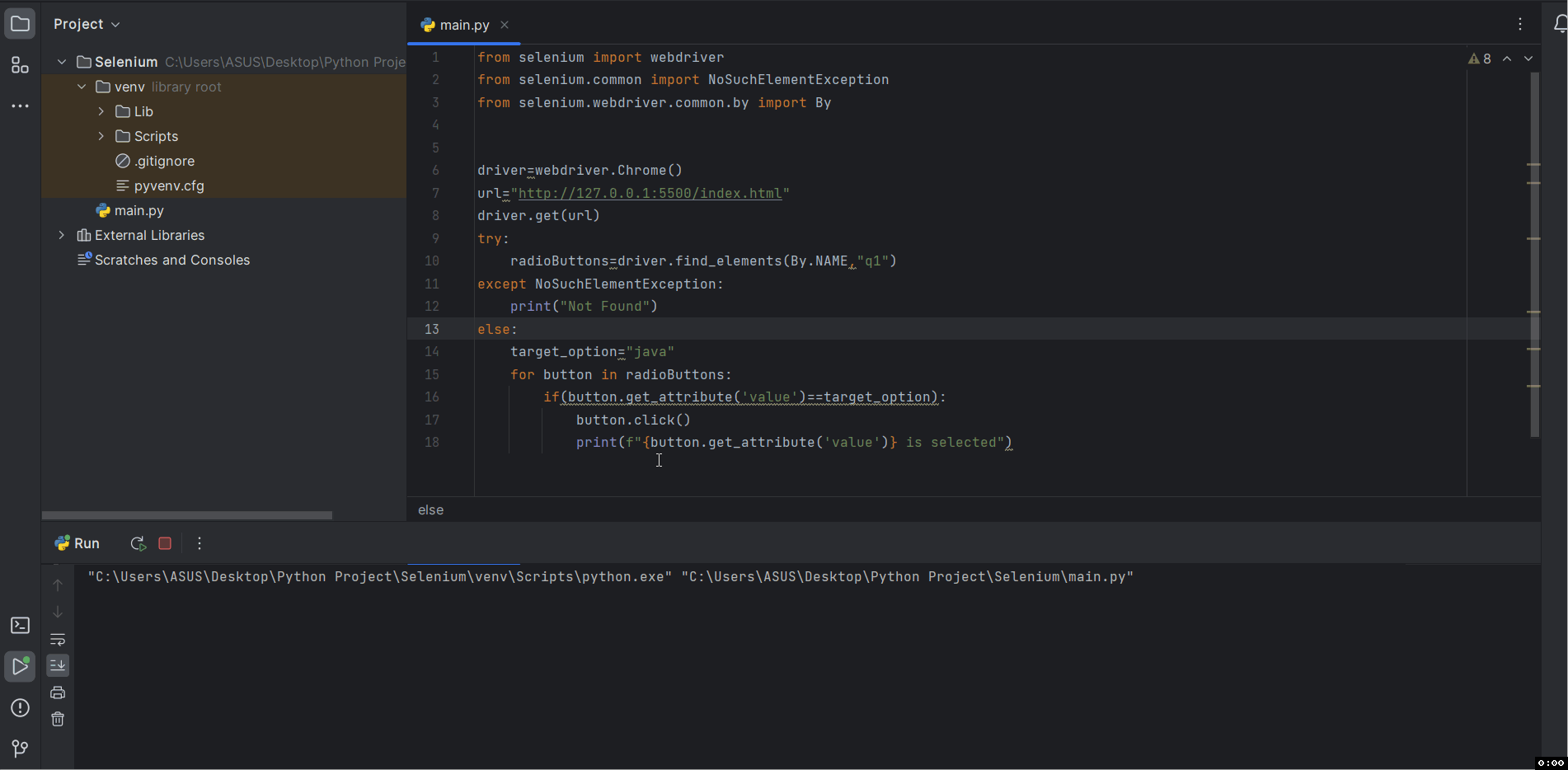
Selenium Handling Radio Button
Conclusion:
Selenium is one of the most popular and powerful tools for automation web applications Selenium is widely used by developers and QA testers to be automating user interactions such as filling out the forms, clicking on a button etc. While we are dealing with web forms handling one of the most common elements of web forms are radio buttons and knowing how to handle a radio button will sure come in handy. Handling a radio button in Selenium is a step by step process right from locating the radio button to interacting with the radio elements from a group . Make sure you follow the each and every step correctly in order to handle and interact with radio buttons smoothly.
Share your thoughts in the comments
Please Login to comment...