Render HTML Forms (GET & POST) in Django
Last Updated :
16 Aug, 2021
Django is often called “Batteries Included Framework” because it has a default setting for everything and has features that can help anyone develop a website rapidly. Talking about forms, In HTML, a form is a collection of elements inside <form>…</form> that allow a visitor to do things like entering text, select options, manipulate objects or controls, and so on, and then send that information back to the server. Basically, it is a collection of data for processing it for any purpose including saving it in the database or fetching data from the database. Django supports all types of HTML forms and rendering data from them to a view for processing using various logical operations.
To know more about HTML forms, visit HTML | form Tag.
Django also provides a built-in feature of Django Forms just like Django Models. One can create forms in Django and use them to fetch data from the user in a convenient manner.
To begin with forms, one needs to be familiar with GET and POST requests in forms.
- GET: GET, by contrast, bundles the submitted data into a string, and uses this to compose a URL. The URL contains the address where the data must be sent, as well as the data keys and values. You can see this in action if you do a search in the Django documentation, which will produce a URL of the form https://docs.djangoproject.com/search/?q=forms&release=1.
- POST: Any request that could be used to change the state of the system – for example, a request that makes changes in the database – should use POST.
Render HTML Forms in Django Explanation
Illustration of Django Forms using an Example. Consider a project named geeksforgeeks having an app named geeks.
Refer to the following articles to check how to create a project and an app in Django.
Let’s create a simple HTML form to show how can you input the data from a user and use it in your view. Enter following code in geeks > templates > home.html
HTML
< form action = "" method = "get" >
< label for = "your_name" >Your name: </ label >
< input id = "your_name" type = "text" name = "your_name" >
< input type = "submit" value = "OK" >
</ form >
|
Now to render it in our view we need to modify urls.py for geeks app.
Enter the following code in geeksforgeeks > urls.py
Python3
from django.urls import path
from .views import geeks_view
urlpatterns = [
path('', home_view ),
]
|
Now, let’s move to our home_view and start checking how are we going to get the data. Entire data from an HTML form in Django is transferred as a JSON object called a request. Let’s create a view first and then we will try all methods to fetch data from the form.
Python3
from django.shortcuts import render
def home_view(request):
return render(request, "home.html" )
|
As we have everything set up let us run Python manage.py run server and check if the form is there on the home page.
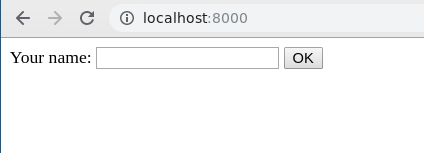
By default every form ever written in HTML makes a GET request to the back end of an application, a GET request normally works using queries in the URL. Let’s demonstrate it using the above form, Fill up the form using your name, and let’s check what happens.
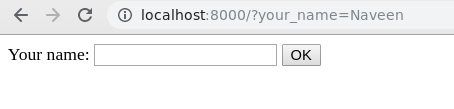
The above URL is appended with a name attribute of the input tag and the name entered in the form. This is how the GET request works whatever be the number of inputs they would be appended to the URL to send the data to the back end of an application. Let’s check how to finally get this data in our view so that logic could be applied based on input.
In views.py
Python3
from django.shortcuts import render
def home_view(request):
print (request.GET)
return render(request, "home.html" )
|
Now when we fill the form we can see the output in the terminal as below:
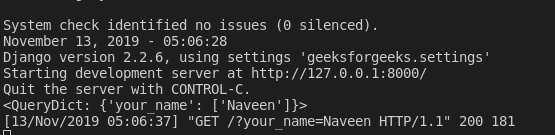
request.GET returns a query dictionary that one can access like any other python dictionary and finally use its data for applying some logic.
Similarly, if the method of transmission is POST, you can use request.POST as query dictionary for rendering the data from the form into views.
In home.html
HTML
< form action = "" method = "POST" >
{% csrf_token %}
< label for = "your_name" >Your name: </ label >
< input id = "your_name" type = "text" name = "your_name" >
< input type = "submit" value = "OK" >
</ form >
|
Note that whenever we create a form request, Django requires you to add {% csrf_token %} in form for security purposes
Now, in views.py let’s check what request.POST has got.
Python3
from django.shortcuts import render
def home_view(request):
print (request.POST)
return render(request, "home.html" )
|
Now when we submit the form it shows the data as below.

This way one can use this data for querying into the database or for processing using some logical operation and pass using the context dictionary to the template.
Share your thoughts in the comments
Please Login to comment...