Python: MySQL Create Table
Last Updated :
10 Jan, 2021
MySQL is a Relational Database Management System (RDBMS) whereas the structured Query Language (SQL) is the language used for handling the RDBMS using commands i.e Creating, Inserting, Updating and Deleting the data from the databases. SQL commands are case insensitive i.e CREATE and create signify the same command.
Installation
Follow the below-mentioned process for installing the dependencies for python MySQL
- Navigate to the python script directory using the command prompt.
- Execute the command
pip install mysql-connector
Python Mysql Connector Module Methods
1. connect(): This function is used for establishing a connection with the MySQL server. The following are the arguments that are used to initiate a connection:
- user: User name associated with the MySQL server used to authenticate the connection
- password: Password associated with the user name for authentication
- database: Data base in the MySQL for creating the Table
2. cursor(): Cursor is the workspace created in the system memory when the SQL command is executed. This memory is temporary and the cursor connection is bounded for the entire session/lifetime and the commands are executed
3. execute(): The execute function takes a SQL query as an argument and executes. A query is an SQL command which is used to create, insert, retrieve, update, delete etc.
Data Base
The database is an organization of information structured into multiple tables. Databases are organized in such a way so that manipulating the data is easy i.e Creating, inserting, updating, and deleting etc.
SQL command for Creating Database :
CREATE DATABASE ;
Example: Consider the below example for creating a database in MySQL(Ex: college)
python
import mysql.connector as SQLC
DataBase = SQLC.connect(
host = "server name" ,
user = "user name" ,
password = "password"
)
Cursor = DataBase.cursor()
Cursor.execute( "CREATE DATABASE College" )
print ( "College Data base is created" )
|
Output :
College Data base is created
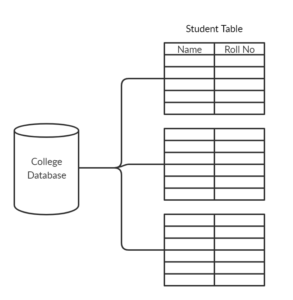
Table
- The table is a collection of data organized in the form of rows and columns. Table is present within a database.
- Rows are also called tuples
- Columns are called the attributes of the table
SQL command for Creating Table :
CREATE TABLE
(
column_name_1 column_Data_type,
column_name_2 column_Data_type,
:
:
column_name_n column_Data_type
);
SQL Data types
Data types are used for defining the type of data that will be stored in the cell of the table.
Different Types of the Datatypes
- Numeric
- Character/String
- Date/time.
- Unicode Character/String
- Binary
Apart from the above-mentioned datatypes, there are other miscellaneous data types in MySQL that include datatypes of CLOB, BLOB, JSON, XML.
Consider the below-mentioned python code for creating a table of the “student” which contains two Columns Name, Roll number in the database “college” previously created.
python
import mysql.connectors as SQLC
def CreateTable():
DataBase = SQLC.connect(
host = "server name" ,
user = "user name" ,
password = "password" ,
database = "College"
)
Cursor = DataBase.cursor()
TableName = "CREATE TABLE Student
(
Name VARCHAR( 255 ),
Roll_no int
);"
Cursor.execute(TableName)
print ( "Student Table is Created in the Database" )
return
CreateTable()
|
Output :
Student Table is Created in the Database
Share your thoughts in the comments
Please Login to comment...