Python Falcon – Cookies
Last Updated :
04 Mar, 2024
In the world of web development, managing user sessions is a fundamental aspect, and cookies serve as a crucial tool in achieving this. Python Falcon, a lightweight and efficient web framework, allows developers to seamlessly handle cookies within their applications. In this article, we will learn about cookies in Python Falcon.
What are Falcon-Cookies in Python?
Cookies are small pieces of data stored on a user’s device by their web browser. These data snippets are sent between the client and the server with each HTTP request, enabling the server to maintain stateful information across multiple requests. Cookies are commonly used for tasks such as user authentication, session management, and personalization
Python Falcon – Cookies Types
Below are some of the examples to understand the Python Falcon Cookies in Python:
- Setting Cookies.
- Secure Attribute.
- Getting Cookies.
- SameSite Attribute.
Setting Cookies in Python Falcon
Setting cookies involves sending small data snippets from a web server to a user’s browser, where they are stored locally. This common web practice is vital for tasks like user authentication, session management, and personalized website experiences. By utilizing cookies, servers can maintain stateful information, enhancing user interactions across multiple sessions.
Example: The Falcon code sets cookies (‘username’ and ‘session_id’) with specific attributes upon receiving an HTTP GET request to “/set_cookies”. It responds with an HTTP 200 status and a success message. The application runs on http://127.0.0.1:8000 using a simple WSGI server.
Python3
import falcon
class CookieResource:
def on_get( self , req, resp):
resp.set_cookie( 'username' , 'JohnDoe' )
resp.set_cookie( 'session_id' , 'abc123' , max_age = 3600 , secure = True )
resp.status = falcon.HTTP_200
resp.body = 'Cookies set successfully'
app = falcon.App()
app.add_route( '/set_cookies' , CookieResource())
if __name__ = = '__main__' :
from wsgiref import simple_server
httpd = simple_server.make_server( '127.0.0.1' , 8000 , app)
httpd.serve_forever()
|
Output:
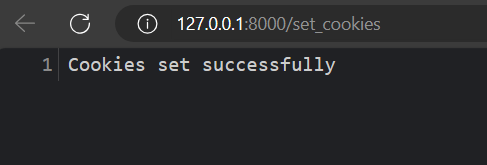
Secure Attribute in Python Falcon
The Secure attribute is an attribute that can be set for an HTTP cookie to enhance its security. When a cookie has the Secure attribute, it indicates that the cookie should only be sent over secure, encrypted connections, typically using HTTPS (HTTP Secure). This ensures that the cookie data is transmitted securely between the client (web browser) and the server.
Example: The Falcon code defines an API endpoint “/set_secure_cookie” that, upon receiving an HTTP GET request, sets a secure cookie named ‘session_id’ with the value ‘abc123’. The application runs on http://127.0.0.1:8000, and a message indicates that the Falcon app is running at the specified endpoint. The simple WSGI server continuously serves the Falcon application.
Python3
import falcon
class SecureCookieResource:
def on_get( self , req, resp):
resp.set_cookie( 'session_id' , 'abc123' , secure = True )
resp.status = falcon.HTTP_200
resp.body = 'Secure cookie set successfully'
app = falcon.App()
app.add_route( '/set_secure_cookie' , SecureCookieResource())
if __name__ = = '__main__' :
from wsgiref import simple_server
httpd = simple_server.make_server( '127.0.0.1' , 8000 , app)
httpd.serve_forever()
|
Output:
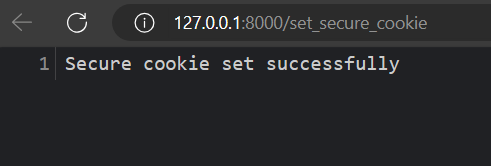
Getting Cookies in Python Falcon
In Python Falcon, “Getting Cookies” refers to the process of retrieving information stored in cookies from a user’s web browser. Cookies are small pieces of data sent by a web server to a user’s browser, where they are stored locally. Subsequently, these cookies can be included in HTTP requests sent back to the server, allowing the server to recognize and retrieve the associated data.
Example: This Python script uses the Falcon web framework to create a simple API with a single route /cookie
. The CookieResource
class handles GET requests, simulating a client request with a cookie. It parses and extracts individual cookies (‘username’ and ‘session_id’), responding with their values. The main
function sets up and runs the Falcon application on http://127.0.0.1:8000 using a WSGI server.
Python3
import falcon
from http.cookies import SimpleCookie
class CookieResource:
def on_get( self , req, resp):
request_headers = { 'Cookie' : 'username=JohnDoe; session_id=abc123' }
cookie = SimpleCookie()
cookie.load(request_headers.get( 'Cookie' , ''))
username = cookie.get(
'username' ).value if 'username' in cookie else None
session_id = cookie.get(
'session_id' ).value if 'session_id' in cookie else None
resp.text = f 'Username: {username}\nSession ID: {session_id}'
def main():
app = falcon.App()
app.add_route( '/cookie' , CookieResource())
from wsgiref import simple_server
httpd = simple_server.make_server( '127.0.0.1' , 8000 , app)
httpd.serve_forever()
if __name__ = = '__main__' :
main()
|
Output:
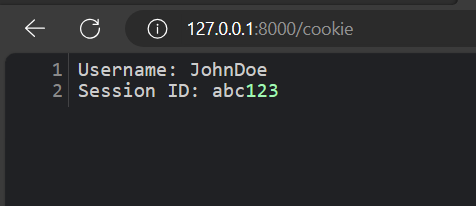
SameSite Attribute in Python Falcon
The SameSite attribute is an important aspect of web cookies that controls when cookies are sent in cross-site (third-party) requests. It aims to mitigate security and privacy risks associated with cross-site request forgery (CSRF) and cross-site scripting (XSS) attacks. The SameSite attribute can have three possible values: “Strict,” “Lax,” or “None.”
Example: This Python script uses the Falcon web framework to create an API with a single route ‘/cookie’. When accessed, the CookieResource
class sets a cookie named ‘my_cookie’ with the value ‘cookie_value’ and a SameSite attribute set to ‘Strict’. The main
function runs the Falcon application on http://127.0.0.1:8000, continuously serving the API and allowing access to the ‘/cookie’ route.
Python3
import falcon
from http.cookies import SimpleCookie
class CookieResource:
def on_get( self , req, resp):
cookie = SimpleCookie()
cookie[ 'my_cookie' ] = 'cookie_value'
cookie[ 'my_cookie' ][ 'SameSite' ] = 'Strict'
resp.text = str (cookie)
def main():
app = falcon.App()
app.add_route( '/cookie' , CookieResource())
from wsgiref import simple_server
httpd = simple_server.make_server( '127.0.0.1' , 8000 , app)
httpd.serve_forever()
if __name__ = = '__main__' :
main()
|
Output:

Share your thoughts in the comments
Please Login to comment...