Python Falcon – Jinja2 Template
Last Updated :
11 Dec, 2023
Python Falcon is a lightweight and minimalist web framework designed for building web APIs, with a particular emphasis on simplicity, speed, and efficiency. Falcon is developed to handle HTTP requests efficiently and is optimized for performance, making it a suitable choice for developers who prioritize building high-performance APIs. It aims to provide the essential features needed for API development without unnecessary overhead, making it a clean and efficient option for projects where simplicity and speed are key considerations. In this article, we will discuss the Python Falcon- Jinja2 Template
Python Falcon – Jinja2 Template
Jinja2 is a template engine for Python that facilitates the creation of dynamic and reusable templates for web applications. It is widely used in web development to separate the logic of the application from the presentation layer. Jinja2 templates allow developers to embed dynamic content within static HTML files, making it easier to generate HTML pages with varying content based on data or user input.
Advantages of Falcon and Jinja2 Integration
The integration of Falcon and Jinja2 brings several advantages to web development:
- Efficiency: The combination of Falcon and Jinja2 results in efficient handling of HTTP requests and the seamless rendering of dynamic content. Falcon’s speed in processing API requests complements Jinja2’s ability to generate HTML dynamically, contributing to an overall efficient and responsive web application.
- Separation of Concerns: The integration allows for a clear separation between the application’s logic (handled by Falcon) and the presentation layer (handled by Jinja2 templates). This separation of concerns enhances the modularity of the codebase, making it easier for developers to understand and maintain. Developers can focus on the business logic in Falcon while leveraging Jinja2 for dynamic and reusable presentation components.
- Reusability: Jinja2 templates encourage the creation of reusable components, fostering a modular approach to web development. This emphasis on reusability enhances code maintainability and promotes a more efficient development process by allowing developers to leverage existing templates for different parts of the application. Overall, the Falcon and Jinja2 integration supports a streamlined and modular development workflow.
Integrate Falcon – Jinja2 Template
Step 1: Install libraries
To initiate a project, the essential Falcon and Jinja2 libraries are required. These can be easily installed using the following commands :
pip install falcon
pip install jinja2
Step 2: Create Project directory
After installing the necessary libraries, create a project directory with the desired name, for example, ‘FalconJinja‘. Within this directory, establish a ‘templates’ directory to house HTML templates. Additionally, include the ‘app.py’ file, which will serve as the main file for the Falcon framework. The structure of the project directory will resemble the following:
File Structure
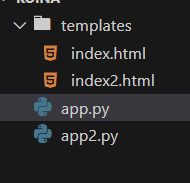
File Structure
Python Falcon – Jinja2 Dynamic Template
index.html : This Falcon and Jinja2 HTML template generates a simple webpage with dynamic content placeholders for ‘id’ and ‘name,’ obtained from the server. The page title is “Falcon and Jinja2 Example.” The ‘id’ is displayed in an h3 element, and the ‘name’ is in an h1 element with green text, enabling dynamic content rendering on the client side based on server data.
HTML
<!DOCTYPE html>< html >
< head >
< title >Falcon and Jinja2 Example</ title ></ head >
< body >
< h3 >Id : {{ id }}</ h3 >
< h1 style = "color: green" >Hello, {{ name }} !</ h1 >
</ body ></ html >
|
app.py : This Python code establishes a Falcon web app, responding to HTTP GET requests by rendering an HTML template through Jinja2. The template, “index.html,” incorporates dynamic data (‘id’ and ‘name’). The app runs locally on http://127.0.0.1:8000 using the WSGI server. Executing the code continuously serves the Falcon app, displaying the rendered HTML content at http://127.0.0.1:8000 in a web browser.
Python3
import falcon
from jinja2 import Environment, FileSystemLoader
app = falcon.App()
jinja2_env = Environment(loader = FileSystemLoader( "templates" ))
class MyResource:
def on_get( self , req, resp):
template = jinja2_env.get_template( "index.html" )
rendered_template = template.render( id = 1 , name = "GFG User" )
resp.status = falcon.HTTP_200
resp.content_type = "text/html"
resp.text = rendered_template
app.add_route( "/" , MyResource())
if __name__ = = "__main__" :
from wsgiref import simple_server
httpd = simple_server.make_server( "127.0.0.1" , 8000 , app)
httpd.serve_forever()
|
Output
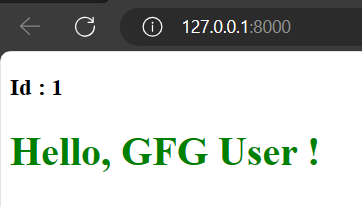
Output
Python Falcon – Jinja2 with Conditional statement Template
index2.html : This HTML code serves as a template using the Falcon web framework and Jinja2. It dynamically displays content based on server-provided data. If the ‘listFlag’ is true, it generates an unordered list of items retrieved from the ‘items’ list. If ‘listFlag’ is false, it indicates that there are no items in the list. The title of the page is set to “Falcon and Jinja2 Example 2,” and the structure allows for conditional rendering of content depending on the server data.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Falcon and Jinja2 Example 2</ title >
</ head >
< body >
{% if listFlag %}
< h2 >Items in list are as follows : </ h2 >
{% for item in items %}
< li >{{item}}</ li >
{% endfor %}
{% else %}
< h2 >No item in list</ h2 >
{% endif %}
</ body >
</ html >
|
app2.py : This Python code establishes a Falcon web app with a single resource, MyResource
, responding to HTTP GET requests by rendering an HTML template, “index2.html,” using Jinja2. The template incorporates a conditional check (‘listFlag’) and shows an unordered list of items [“One”, “Two”, “Three”] if the flag is true; otherwise, it indicates an empty list. The app runs on a local server at http://127.0.0.1:8000 using the WSGI server, continuously serving the Falcon app, and displaying the dynamic list at http://127.0.0.1:8000 in a web browser.
Python3
import falcon
from jinja2 import Environment, FileSystemLoader
app = falcon.App()
jinja2_env = Environment(loader = FileSystemLoader( "templates" ))
class MyResource:
def on_get( self , req, resp):
template = jinja2_env.get_template( "index2.html" )
rendered_template = template.render(listFlag = True , items = [ "One" , "Two" , "Three" ])
resp.status = falcon.HTTP_200
resp.content_type = "text/html"
resp.text = rendered_template
app.add_route( "/" , MyResource())
if __name__ = = "__main__" :
from wsgiref import simple_server
httpd = simple_server.make_server( "127.0.0.1" , 8000 , app)
httpd.serve_forever()
|
Output
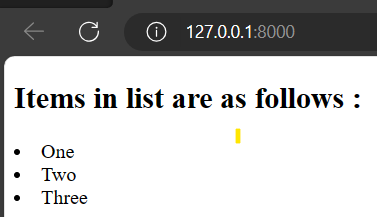
Output
Conclusion
In conclusion, the fusion of Python Falcon and Jinja2 templates creates a potent synergy, combining Falcon’s lightweight yet efficient API framework with Jinja2’s dynamic and reusable template engine. This integration results in streamlined web development, featuring efficient HTTP request handling, a clear separation of concerns, and a focus on modular, reusable components. The Falcon-Jinja2 partnership stands out as a versatile and effective solution for developers, providing a straightforward and powerful approach to building high-performance APIs and dynamic web applications in Python.
Share your thoughts in the comments
Please Login to comment...