Python Falcon – Waitress
Last Updated :
06 Dec, 2023
In this article, we will explore Python Falcon-Waitress, a powerful combination for building web APIs with Falcon, and we’ll illustrate its usage with practical examples. Falcon-Waitress offers seamless integration of the Falcon framework with the Waitress production-quality WSGI server, enabling you to create robust and performant web services effortlessly.
What is Falcon?
Falcon is a minimalistic, high-performance Python library designed for constructing web APIs and applications in Python. It is engineered to deliver speed, efficiency, and a lightweight footprint, making it an ideal choice for the development of APIs demanding high throughput and low latency. Falcon is tailored for the creation of RESTful APIs and web services.
What is a Waitress?
Waitress is a standout among Python’s Web Server Gateway Interface (WSGI) servers. This pure-Python server is characterized by its lightweight nature, exceptional efficiency, and a reputation for being a production-quality web server. It excels in the task of serving web applications developed in Python and is frequently chosen for deploying a wide spectrum of Python web applications, whether they are built using popular frameworks such as Flask, Django, or Falcon.
Key Features of Waitress
- Lightweight and Efficient: Waitress is designed to be lightweight and optimized for performance, making it an excellent choice for web applications with low resource requirements.
- Pure Python Implementation: Waitress is implemented entirely in Python, which ensures platform-independence. This means you can seamlessly use it on various operating systems, including Windows, Linux, and macOS, without compatibility issues.
- WSGI Compliance: Waitress adheres to the Web Server Gateway Interface (WSGI) standard, ensuring compatibility with a wide range of Python web frameworks like Flask, Django, and Falcon. It allows developers to deploy their web applications effortlessly.
- Production-Quality: Waitress is trusted for production environments, offering the reliability and stability needed to serve web applications with confidence. It can handle high traffic loads and deliver content with minimal latency.
- Ease of Deployment: Deploying web applications with Waitress is straightforward, as it provides a user-friendly and consistent way to serve your Python-based web projects. This feature simplifies the deployment process and reduces the overhead of server management.
Implementation
To set up a simple Falcon API and deploy it using Waitress, follow the steps below:
Step 1: Installation
Before we start, ensure you have Falcon and Waitress libraries installed. You can install them using pip:
pip install falcon
pip install waitress
Step 2: Syntax
To serve any application using the Waitress server class, you’ll need three essential parameters: server(appName, host, port)
. Here’s how you can use it:
For instance, if you want to serve your Falcon app on the localhost (127.0.0.1) and port 8000, you’ll need to add the following line in your code file. This line will instruct Waitress to serve your Falcon app on the specified host and port
serve(app, host="127.0.0.1", port=8000)
Step 3: How to run the code
For run the Python Falcon-Waitress code use the below command : e.g. main is our python file name.
python main:py
Example 1:
Inside “app.py,” define a Falcon resource class named “MyResource.” This class contains a single method, “on_get,” which is triggered when an HTTP GET request is made to this resource. In this case, the method sets the response status to 200 (OK), specifies the content type as text, and provides the response text as “Hello, GFG User!”—this text will be sent back to the client
Python3
from waitress import serve
import falcon
class MyResource:
def on_get( self , req, resp):
resp.status = falcon.HTTP_200
resp.content_type = falcon.MEDIA_TEXT
resp.text = "Hello, GFG User !"
app = falcon.App()
myResource = MyResource()
app.add_route( "/" , myResource)
if __name__ = = "__main__" :
serve(app, host = "127.0.0.1" , port = 8000 )
|
Output:
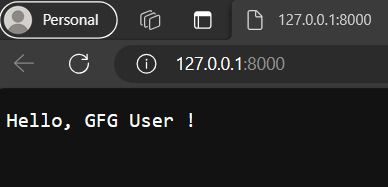
Output
Example 2:
Code defines a Falcon resource class, “UserResource,” which handles GET requests to a URL of the form ‘/user/{username}’ where ‘username’ is a dynamic field obtained from the URL. When a request is made to this URL, the ‘on_get’ method of the ‘UserResource’ class responds with a 200 OK status, a text content type, and a greeting message containing the provided ‘username.’ The code creates a Falcon application, adds a route mapping to the ‘UserResource’ instance, and uses Waitress to serve the application on ‘http://127.0.0.1:8000‘ if the script is executed as the main program. When the server starts, it listens for requests, and dynamic usernames in the URL will generate personalized responses.
Python3
from waitress import serve
import falcon
class UserResource:
def on_get( self , req, resp, username):
resp.status = falcon.HTTP_200
resp.content_type = falcon.MEDIA_TEXT
resp.text = f "Hello, {username} !"
app = falcon.App()
userResource = UserResource()
app.add_route( "/user/{username}" , userResource)
if __name__ = = "__main__" :
serve(app, host = "127.0.0.1" , port = 8000 )
|
Output:
.gif)
Output :
Advantages of Waitress:
- Open-Source Flexibility: Waitress is open-source, allowing users to customize and modify it to meet specific project requirements. This flexibility makes it a valuable choice for projects with unique needs, enabling tailored solutions.
- Efficiency and Performance: Waitress is designed for speed and efficiency, capable of handling a significant number of requests per second. This efficiency makes it well-suited for production environments, ensuring fast content delivery to clients.
- Easy Deployment: Deploying web applications with Waitress is straightforward. Developers can quickly get their applications up and running by directing Waitress to the WSGI application and specifying the host and port. This simplicity streamlines the deployment process. Waitress’s combination of open-source flexibility, efficiency, and easy deployment makes it a suitable choice for a wide range of web application projects, from simple to moderately complex ones.
Disadvantages of Waitress:
- Single-Threaded Nature: Waitress operates as a single-threaded server by default, meaning it can handle only one request at a time. While this may work well for many applications, it can lead to performance issues in high-concurrency situations without additional setup or the use of complementary tools.
- Limited Features: Waitress is relatively simple in terms of features. It is primarily designed to serve WSGI applications and does not provide the advanced functionalities often found in more complex web servers. This simplicity is beneficial for straightforward use cases but may limit its suitability for projects requiring specific server features.
- Concurrency Challenges: Due to its single-threaded nature, Waitress may not be ideal for scenarios that require handling a large number of concurrent requests simultaneously. Developers may need to consider alternative solutions or perform additional configuration to address these challenges effectively.
Conclusion:
Waitress is a lightweight and efficient choice for serving WSGI applications, offering advantages like open-source availability, speed, and ease of deployment. It’s well-suited for many web applications, particularly those with moderate to high levels of traffic. However, it may not be the best fit for extremely high-concurrency scenarios due to its single-threaded nature, and it lacks some of the advanced features provided by more complex web servers. Therefore, the choice of Waitress or any other web server should depend on the specific requirements and demands of your project.
Share your thoughts in the comments
Please Login to comment...