Python Falcon Introduction
Last Updated :
29 Sep, 2023
Python Falcon is a lightweight, high-performance web framework that’s well-suited for building RESTful APIs. It’s easy to learn, efficient, and perfect for projects where speed and simplicity are priorities. In this article, we introduced Falcon and created a basic “Hello World” application to help you get started with this powerful framework.
What is Python Falcon?
Python Falcon is a simple web framework designed to build efficient APIs. If you’re looking for a system that prioritizes speed and performance while having a simple and easy-to-understand design, Falcon could be the right choice for your project
Features of Python Falcon
Below are some features of Python Falcon:
- Lightweight: Falcon is known for its minimalistic design. It doesn’t include unnecessary features or dependencies, making it a lightweight option. This focus on simplicity results in faster execution and lower memory overhead.
- High Performance: Falcon is optimized for high performance. It’s designed to handle a large number of requests efficiently, making it an excellent choice for building APIs where speed is crucial.
- RESTful by Design: Falcon is built with RESTful API development in mind. It encourages best practices for designing REST APIs and provides tools to help you create clean and maintainable code.
- Easy to Learn: Falcon’s simplicity makes it easy for developers to pick up quickly. If you’re familiar with Python and REST principles, you’ll find it straightforward to start building APIs with Falcon.
- Flexibility: Falcon applications are flexible and is very useful for applications with high complexity and need high-performance tuning.
Installation and Setup of Python Falcon
To get started with Falcon, you need to install Python, if not then install Python3 and Install pip. Then, you need to install Falcon using the following command
pip install falcon
Create First Project
We import the Falcon framework. We define a class called HelloWorldResource that will handle the GET requests to the ‘/hello’ endpoint. Inside the on_get method, we set the HTTP response status to 200 OK and provide the response text.
Python3
import falcon
from waitress import serve
class HelloWorldResource:
def on_get( self , req, resp):
resp.status = falcon.HTTP_200
resp.text = "Hello, Falcon World!"
app = falcon.App()
app.add_route( '/hello' , HelloWorldResource())
if __name__ = = '__main__' :
serve(app, host = '127.0.0.1' , port = 8000 )
|
Now, you can run your Falcon application. In your terminal, navigate to the directory containing app.py and run the following command:
gunicorn app:app
Output
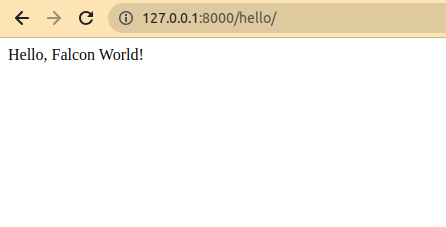
Advantages of Falcon
- Need for Speed: Falcon’s forte is speed and efficiency. If your project involves a high volume of requests or you’re working with limited resources, Falcon’s got your back.
- Simple and User-Friendly: The Falcon’s simplicity is one of its greatest assets. It doesn’t take long to get the hang of it, especially if you already know Python. This means you can easily jump right into building an API.
- Built to Scale: Falcon’s minimalistic architecture makes it highly scalable. Whether you’re deploying on a small virtual server or a massive cloud infrastructure, Falcon can handle it.
- Community and Documentation: While Falcon may not be as popular as some other programs, it boasts an active community and well-maintained documentation. You will not be left in the dark when you need help or resources.
Disadvantages of Falcon
- Features Lite: Falcon’s minimalistic approach has its drawbacks. It lacks some of the features you might find in more feature-rich frameworks like Django or Flask. If your project relies heavily on such features, Falcon may not be your best bet.
- Async Learning Curve: Although the Falcon supports asynchronous programming, it can be a bit difficult for newbies to understand. If your project involves a lot of asynchronous processing, be prepared to spend some time understanding this aspect of Falcon.
- Smaller Ecosystem: Falcon’s smaller user base compared to other Python frameworks means you’ll have access to fewer third-party extensions and libraries. You might need to build certain functionality from scratch or rely on custom solutions.
Share your thoughts in the comments
Please Login to comment...