Python Falcon – get POST data
Last Updated :
12 Dec, 2023
In this discussion, we will delve into retrieving Python Falcon – get POST data. Falcon is a lightweight and speedy web framework explicitly crafted for constructing RESTful APIs. Effective handling of POST data is essential in API development, enabling the creation, updating, or manipulation of resources. This article process of skillfully retrieving POST data using Falcon.
Python Falcon – get POST data
The following are key concepts related to obtaining Python Falcon – get POST data.
- Falcon Request Object:
- In Python Falcon, the Request object is a component that encapsulates the details of an incoming HTTP request.
- This object holds information about the request, such as headers, parameters, and importantly, any data sent in the request body by the client.
- For methods like POST, where clients can send data to the server, the Request object becomes crucial for accessing and processing this incoming information.
- Handling POST Requests:
- To handle POST requests in Falcon, you need to define a resource class. This class should have a method that corresponds to the HTTP POST method.
- Falcon, being a web framework, routes incoming requests to the appropriate method within the defined resource class based on both the HTTP method (POST, GET, etc.) and the URL specified in the program code.
- This allows developers to organize their code in a structured manner, with different methods in the resource class handling different types of HTTP requests.
- Parsing POST Data:
- Parsing POST data is a crucial step in extracting meaningful information from the incoming request.
- Falcon supports various media types for request and response bodies, including JSON, form data, and more.
- The choice of media type depends on how the client sends the data. In the context of the explanation, the focus is on JSON data for simplicity. This means that clients are expected to send data in JSON format, and Falcon provides functionality to parse and work with this JSON data within the resource class.
Example: Falcon Task API
This Python code defines a RESTful API using the Falcon web framework. The API handles POST requests to create new tasks. The `TaskResource` class, when triggered by a POST request, retrieves and parses JSON data from the request body. It then extracts task details such as task ID, title, and completion status. If both task ID and title are present, it processes the task (in this case, printing the details) and responds with a success message and the task details in JSON format. If either the JSON data is invalid, or if task ID or title is missing, appropriate error responses are generated. The Falcon application sets up a route at ‘/tasks‘ for the `TaskResource` class, and the development server is initiated to run the API on ‘http://127.0.0.1:8000/’. The server listens for incoming requests, and upon execution, it prints the server address and serves the API indefinitely.
Python3
import falcon
import json
class TaskResource:
def on_post( self , req, resp):
try :
task_data = json.loads(req.bounded_stream.read().decode( 'utf-8' ))
task_id = task_data.get( 'task_id' )
title = task_data.get( 'title' )
completed = task_data.get( 'completed' , False )
if task_id is not None and title is not None :
print (f "New Task - ID: {task_id}, Title: {title}, Completed: {completed}" )
response_data = {
'message' : 'Task created successfully' ,
'task_details' : {
'task_id' : task_id,
'title' : title,
'completed' : completed
}
}
resp.status = falcon.HTTP_201
resp.text = json.dumps(response_data)
else :
raise ValueError( "Both task_id and title are required in the request JSON." )
except json.JSONDecodeError as e:
resp.status = falcon.HTTP_400
resp.text = json.dumps({ 'error' : 'Invalid JSON format' })
except ValueError as e:
resp.status = falcon.HTTP_400
resp.text = json.dumps({ 'error' : str (e)})
except Exception as e:
resp.status = falcon.HTTP_500
resp.text = json.dumps({ 'error' : 'Internal Server Error' })
app = falcon.App()
app.add_route( '/tasks' , TaskResource())
if __name__ = = '__main__' :
from wsgiref import simple_server
host = '127.0.0.1'
port = 8000
httpd = simple_server.make_server(host, port, app)
print (f "Serving on http://{host}:{port}" )
httpd.serve_forever()
|
Run the server
python app.py
Serving on http://127.0.0.1:8000/tasks
Output:
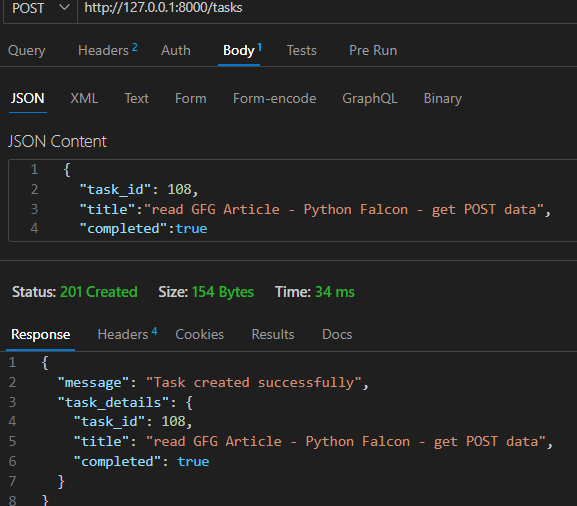
Output
Video demonstration
Conclusion
In conclusion, the provided Python Falcon code demonstrates the handling of POST requests to create tasks in a RESTful API. Leveraging the Falcon web framework, the code efficiently retrieves and parses JSON data from the request body, extracting essential task details. Error handling is implemented to address scenarios such as invalid JSON format or missing task information. The resulting API, accessible at ‘/tasks’, showcases Falcon’s simplicity and effectiveness in building lightweight and performant RESTful services. The code is well-structured, emphasizing the framework’s capabilities in handling HTTP requests and responses seamlessly.
Share your thoughts in the comments
Please Login to comment...