Python Falcon – Hello World
Last Updated :
25 Sep, 2023
Falcon is a powerful and minimalistic Python framework for building high-performance APIs. In this article, we’ll take a closer look at Falcon and build a simple “Hello World” application using the WSGI (Web Server Gateway Interface) specification.
What is Falcon?
Falcon is an open-source Python web framework that follows the minimalist philosophy. It doesn’t come with a lot of built-in features, but it provides a solid foundation for building RESTful APIs quickly. Falcon is designed to be lean and efficient, making it an excellent choice for microservices and applications where performance is critical.
Install Falcon
Before we dive into creating a “Hello World” application, you’ll need to install Falcon. You can do this using pip, Python’s package manager:
pip install falcon
Python Falcon – Hello World
Let’s create a minimal Falcon application that responds with “Hello, World!” when accessed. Let us put all these code fragments in hello_falcon.py
Code Explain
In the code above, we define a HelloWorldResource class with an on_get method. This method is called when a GET request is made to the root path (“/”) of our API. It sets the HTTP status code to 200 (OK) and sets the response body to “Hello, World!”.
We then create a Falcon app instance and add our HelloWorldResource to it using the app.add_route method.
Python3
import falcon
from wsgiref import simple_server
class HelloWorldResource:
def on_get( self , req, resp):
resp.status = falcon.HTTP_200
resp.text = "Hello, World!"
app = falcon.App()
app.add_route( "/" , HelloWorldResource())
if __name__ = = "__main__" :
httpd = simple_server.make_server( "localhost" , 8000 , app)
httpd.serve_forever()
|
Output
Run this code from the command prompt.
(falconenv) E:\falconenv>python hello_falcon.py
Serving on port 8000...
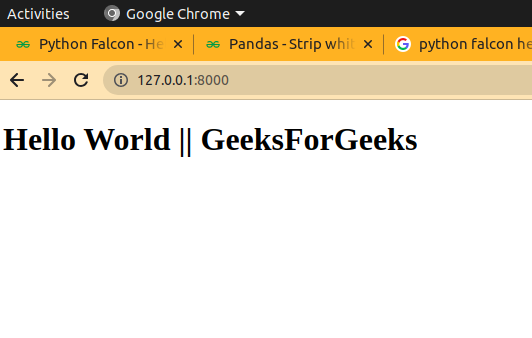
Share your thoughts in the comments
Please Login to comment...