Program to calculate area and perimeter of a rhombus whose diagonals are given
Last Updated :
23 Jul, 2022
Given the length of diagonals of a rhombus, d1 and d2. The task is to find the perimeter and the area of that rhombus.
A rhombus is a polygon having 4 equal sides in which both the opposite sides are parallel, and opposite angles are equal.
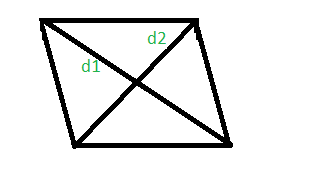
Examples:
Input: d1 = 2 and d2 = 4
Output: The area of rhombus with diagonals 2 and 4 is 4.
The perimeter of rhombus with diagonals 2 and 4 is 8.
Input: d1 = 100 and d2 = 500
Output: The area of rhombus with diagonals 100 and 500 is 25000.
The perimeter of rhombus with diagonals 100 and 500 is 1019.
Area of the rhombus = 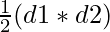
Perimeterof the rhombus = 
Below is the implementation of above approach:
C++
#include <iostream>
#include <math.h>
using namespace std;
int rhombusAreaPeri( int d1, int d2)
{
long long int area, perimeter;
area = (d1 * d2) / 2;
perimeter = 2 * sqrt ( pow (d1, 2) + pow (d2, 2));
cout << "The area of rhombus with diagonals "
<< d1 << " and " << d2 << " is " << area << "." << endl;
cout << "The perimeter of rhombus with diagonals "
<< d1 << " and " << d2 << " is " << perimeter << "." << endl;
}
int main()
{
int d1 = 2, d2 = 4;
rhombusAreaPeri(d1, d2);
return 0;
}
|
Java
import java.io.*;
class GFG {
static int rhombusAreaPeri( int d1, int d2)
{
int area, perimeter;
area = (d1 * d2) / 2 ;
perimeter = ( int )( 2 * Math.sqrt(Math.pow(d1, 2 ) + Math.pow(d2, 2 )));
System.out.println( "The area of rhombus with diagonals "
+ d1 + " and " + d2 + " is " + area + "." );
System.out.println( "The perimeter of rhombus with diagonals "
+d1 + " and " + d2 + " is " + perimeter + "." );
return 0 ;
}
public static void main (String[] args) {
int d1 = 2 , d2 = 4 ;
rhombusAreaPeri(d1, d2);
}
}
|
Python3
from math import sqrt, pow
def rhombusAreaPeri(d1, d2):
area = (d1 * d2) / 2
perimeter = 2 * sqrt( pow (d1, 2 ) +
pow (d2, 2 ))
print ( "The area of rhombus with diagonals" ,
d1, "and" , d2, "is" , area, "." )
print ( "The perimeter of rhombus with diagonals" ,
d1, "and" , d2, "is" , perimeter, "." )
if __name__ = = '__main__' :
d1 = 2
d2 = 4
rhombusAreaPeri(d1, d2)
|
C#
using System;
class GFG
{
static int rhombusAreaPeri( int d1,
int d2)
{
int area, perimeter;
area = (d1 * d2) / 2;
perimeter = ( int )(2 * Math.Sqrt(Math.Pow(d1, 2) +
Math.Pow(d2, 2)));
Console.WriteLine( "The area of rhombus with " +
"diagonals " + d1 + " and " +
d2 + " is " + area + "." );
Console.WriteLine( "The perimeter of rhombus " +
"with diagonals " + d1 + " and " +
d2 + " is " + perimeter + "." );
return 0;
}
public static void Main ()
{
int d1 = 2, d2 = 4;
rhombusAreaPeri(d1, d2);
}
}
|
PHP
<?php
function rhombusAreaPeri( $d1 , $d2 )
{
$area = ( $d1 * $d2 ) / 2;
$perimeter = 2 * sqrt(pow( $d1 , 2) +
pow( $d2 , 2));
echo "The area of rhombus with diagonals " . $d1 .
" and " . $d2 . " is " . $area . "." . "\n" ;
echo "The perimeter of rhombus with diagonals " . $d1 .
" and " . $d2 . " is " . $perimeter . "." . "\n" ;
}
$d1 = 2; $d2 = 4;
rhombusAreaPeri( $d1 , $d2 );
?>
|
Javascript
<script>
function rhombusAreaPeri(d1, d2)
{
let area, perimeter;
area = Math.floor((d1 * d2) / 2);
perimeter = Math.floor(2 * Math.sqrt(Math.pow(d1, 2) + Math.pow(d2, 2)));
document.write( "The area of rhombus with diagonals "
+ d1 + " and " + d2 + " is " + area + "." + "<br>" );
document.write( "The perimeter of rhombus with diagonals "
+ d1 + " and " + d2 + " is " + perimeter + "." + "<br>" );
}
let d1 = 2, d2 = 4;
rhombusAreaPeri(d1, d2);
</script>
|
Output: The area of rhombus with diagonals 2 and 4 is 4.
The perimeter of rhombus with diagonals 2 and 4 is 8.
Time Complexity: O(log(d12+d22))
Auxiliary Space: O(1), since no extra space has been taken.
Share your thoughts in the comments
Please Login to comment...