Passing data from Child to Parent Component in Angular
Last Updated :
01 Apr, 2024
In Angular, passing data from a child component to its parent component involves emitting events. Essentially, the child component emits an event containing the data that the parent component needs to receive. This is typically achieved using Angular’s EventEmitter class, where the child component emits an event with the data payload, and the parent component listens for this event and handles the data accordingly.
By using event emission, Angular provides communication between components, allowing them to interact and share information seamlessly within the application.
Prerequisites:
Approach:
- Define an Output property in the child component: In the child component TypeScript file, create an Output property using Angular’s EventEmitter. This will emit an event to the parent component.
- Emit the event with data: Within the child component logic, when you want to pass data to the parent, emit an event using the EventEmitter’s emit() method. You can pass the data as an argument to this method.
- Handle the event in the parent component: In the parent component’s HTML template, bind to the event emitted by the child component using the event binding syntax. Then, create a method in the parent component to handle the emitted event.
- Receive the data in the parent component: In the method created to handle the event in the parent component TypeScript file, access the data passed by the child component through the event object.
Steps to create app:
Step 1: Create a new Angular project
ng new child-to-parent
Step 2: Navigate to the project directory
cd child-to-parent
Step 3: Serve the application
ng serve
Project Structure:
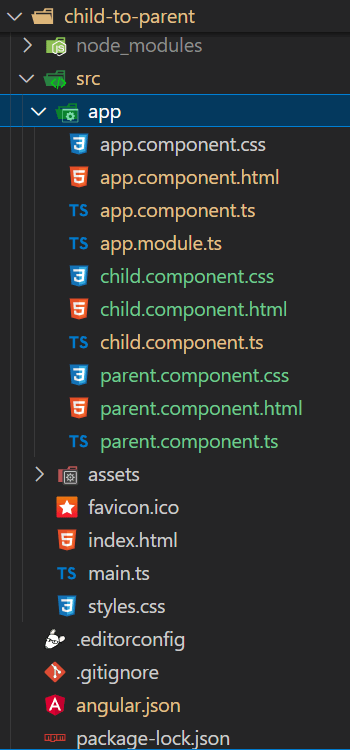
Dependencies:
"dependencies": {
"@angular/animations": "^16.0.0",
"@angular/common": "^16.0.0",
"@angular/compiler": "^16.0.0",
"@angular/core": "^16.0.0",
"@angular/forms": "^16.0.0",
"@angular/platform-browser": "^16.0.0",
"@angular/platform-browser-dynamic": "^16.0.0",
"@angular/router": "^16.0.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.13.0"
}
Example: Create the required files as shown in folder structure and add the following codes.
HTML
<!-- app.component.html -->
<app-parent></app-parent>
HTML
<!-- parent.component.html -->
<div class="parent-container">
<h2>Parent Component</h2>
<p>Data received from child: {{ receivedData }}</p>
<app-child (dataEvent)=
"handleDataFromChild($event)"></app-child>
</div>
HTML
<!-- child.component.html -->
<div class="child-container">
<button class="child-button" (click)="sendDataToParent()">
Send Data to Parent
</button>
</div>
CSS
/*parent.component.css*/
.parent-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
min-height: 100vh;
}
h2 {
color: #333;
font-size: 24px;
margin-bottom: 10px;
}
p {
color: #666;
font-size: 18px;
}
CSS
/*child.component.css*/
.child-container {
display: flex;
align-items: center;
justify-content: center;
}
.child-button {
background-color: #007bff;
color: #fff;
border: none;
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.child-button:hover {
background-color: #0056b3;
}
JavaScript
//app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
})
export class AppComponent { }
JavaScript
//app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { ParentComponent } from './parent.component';
import { ChildComponent } from './child.component';
@NgModule({
declarations: [
AppComponent,
ParentComponent,
ChildComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
JavaScript
//parent.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
templateUrl: './parent.component.html',
styleUrls: ['./parent.component.css']
})
export class ParentComponent {
receivedData: string;
constructor() {
this.receivedData = '';
}
handleDataFromChild(data: string) {
this.receivedData = data;
console.log('Data received in parent:', this.receivedData);
}
}
JavaScript
//child.component.ts
import { Component, EventEmitter, Output } from '@angular/core';
@Component({
selector: 'app-child',
templateUrl: './child.component.html',
styleUrls: ['./child.component.css']
})
export class ChildComponent {
@Output() dataEvent = new EventEmitter<string>();
sendDataToParent() {
const data = 'Hello from child';
this.dataEvent.emit(data);
}
}
Output:
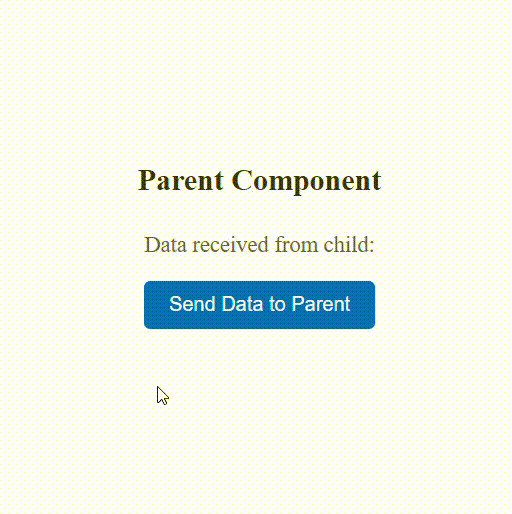
Share your thoughts in the comments
Please Login to comment...