How to Pass Value from One Child Component to Another in VueJS ?
Last Updated :
19 Jul, 2023
Vue.js is a JavaScript framework used in building powerful and beautiful user interfaces. The key feature of Vue.js is its component-based architecture that allows the developers to create reusable and modular components.
In this article, we will learn how to pass value from one child component to another in VueJS. There are sometimes when we need to pass data from one child component to another, either for communication or to update the state of the components.
Steps to Create Vue App:
Step 1: Install Vue modules using the below npm command
npm install vue
Step 2: Use Vue JS through CLI. Open your terminal or command prompt and run the below command.
npm install --global vue-cli
Step 3: Create the new project using the below command
vue init webpack myproject
Step 4: Change the directory to the newly created project
cd myproject
Project Structure:
The following project structure will be generated after completing the above-required steps:
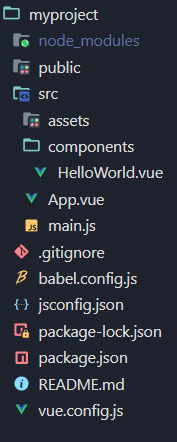
Approach 1: Using Props
In the first approach, we will first pass the value from the first child component to the parent component using props, and after which we will pass it from the parent component to the second child component.
Example: Below example demonstrates the passing of value from one child component to another using props in vuejs.
HTML
< template >
< div >
< h2 >I'm a Child Component</ h2 >
< p >Received Message: {{ msg }}</ p >
</ div >
</ template >
< script >
export default {
name: "ChildComponent",
props: {
msg: {
type: String,
required: true,
},
},
};
</ script >
|
HTML
< template >
< div >
< h1 style = "color: green" >GeeksforGeeks</ h1 >
< h2 >I'm an Parent Component</ h2 >
< p >Sent Message: {{ msg }}</ p >
< ChildComponent :msg = "msg" />
</ div >
</ template >
< script >
import ChildComponent from "./components/ChildComponent.vue";
export default {
name: "App",
data() {
return {
msg: "Welcome Geek from App",
};
},
components: {
ChildComponent,
},
};
</ script >
|
Output:
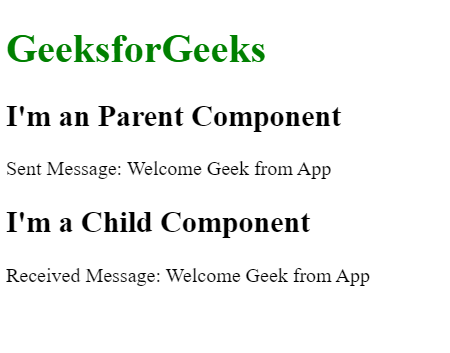
Approach 2: Using Event Bus
In the second approach, we will create a shared service or event bus using a separate Vue instance to facilitate communication between components.
Example: Below example demonstrates the passing of value from one child component to another using the event bus in vuejs.
HTML
< template >
< div >
< h2 >I'm a Child Component</ h2 >
< p >Message recieved from parent: {{ msg }}</ p >
</ div >
</ template >
< script >
export default {
name: "ChildComponent",
data() {
return {
msg: "",
};
},
methods: {
updateChildMessage() {
this.msg =
"I'm the updated message from child component";
},
},
};
</ script >
|
HTML
< template >
< div >
< h1 style = "color: green" >GeeksforGeeks</ h1 >
< h2 >I'm an Parent Component</ h2 >
< button @ click = "msgUpdate" >Update Message</ button >
< ChildComponent ref = "childComponent" />
</ div >
</ template >
< script >
import ChildComponent
from "./components/ChildComponent.vue";
export default {
name: "App",
methods: {
msgUpdate() {
this.msg =
"Updated Message from App Component";
this.$refs.childComponent.updateChildMessage();
},
},
components: {
ChildComponent,
},
};
</ script >
|
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...