How to Call a Parent Method from a Child Component in Vue 3 ?
Last Updated :
26 Apr, 2024
Vue.js is a JavaScript framework for building user interfaces. In Vue 3 parent and child component can communicate with each other through props and events. Props are used to pass the data from the parent to the child component and events are used to pass the data from child to parent component.
These are the following approaches:
Setting Up the Project Environment
Step 1:Â Create a Vue.js application
npm create vue@latest
Step 2:Â Navigate to your project directory and install dependencies:
cd your-project-name
npm install
Step 4: Create the Parent Component
Inside the src/component directory create a new file called ParentComponent.vue.
Step 5: Create the Child Component
Inside the src/component directory create a new file called ChildComponent.vue.
Step 6: Import and Use Component in App.vue
Open src/App.vue and import both child and parent component.
Project Structure:
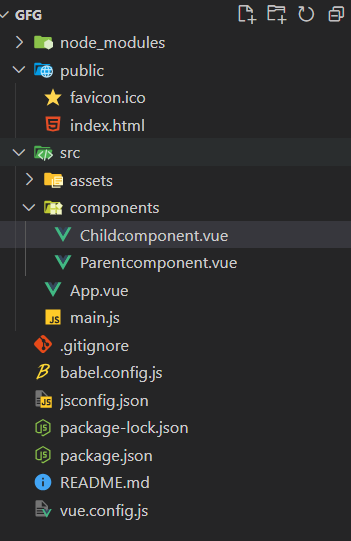
Using $emit to emit an event from the child component
This approach is recommended approach which follows the principle of Vues component communication. In this approach the child component use $emit instance method to emit an event with an optional payload and in parent component you listen for the emitted event using the v-on directive and define a method to handle the event.
Example: This example shows the implementation of the above-explained approach.
JavaScript
// Parent Component
<template>
<div>
<child-component @greet="handleGreetMessage">
</child-component>
<h2 style="text-align: center;">
{{ greetMessage }}</h2>
</div>
</template>
<script>
import ChildComponent from './Childcomponent.vue';
export default {
name: 'ParentComponent',
components: {
ChildComponent
},
data() {
return {
greetMessage: ''
}
},
methods: {
handleGreetMessage(message) {
this.greetMessage = `Parent says: ${message}`;
}
}
}
</script>
JavaScript
// Child Component
<template>
<div>
<button style="display:block;margin:auto;"
@click="emitEvent('hello')">Say Hello</button>
</div>
</template>
<script>
export default {
name: 'ChildComponent',
methods: {
emitEvent(message) {
this.$emit('greet', message);
}
}
}
</script>
Output:
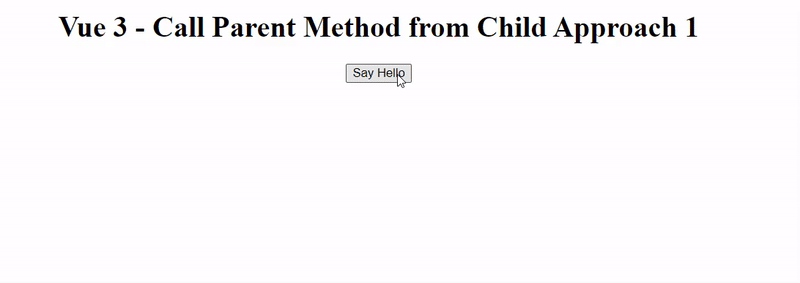
Step 7: Run the Project
Run the project in terminal using following command
npm run serve
Using $parent or $root to access the parent component directly
This approach is not recommended as it tightly couples the child component to its parent which make it harder to maintain and reuse the child component in other parts of the application. In this approach the child component parent component method using $parent or $root instance properties.
Example: This example shows the implementation of the above-explained approach.
JavaScript
<!-- ParentComponent.vue -->
<template>
<div>
<child-component></child-component>
</div>
<h2 style="text-align: center;">
{{ greetMessage }}</h2>
</template>
<script>
import ChildComponent from './Childcomponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
greetMessage: ''
}
},
methods: {
parentMethod() {
this.greetMessage = 'Hello from Parent Component';
}
}
}
</script>
JavaScript
<!-- ChildComponent.vue -->
<template>
<div>
<button style="display: block; margin: auto;"
@click="callParentMethod">
Call Parent Method</button>
</div>
</template>
<script>
export default {
methods: {
callParentMethod() {
this.$parent.parentMethod();
}
}
}
</script>
Output:
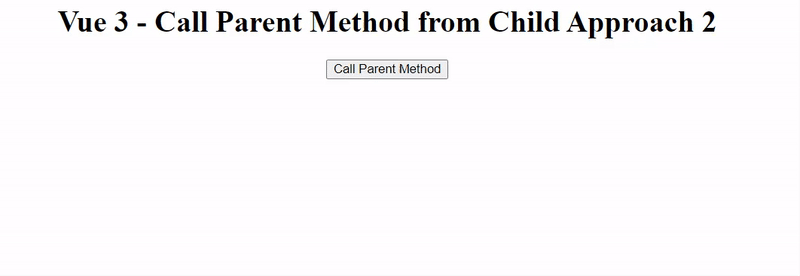
Share your thoughts in the comments
Please Login to comment...