Navigate Component in React Router
Last Updated :
28 Mar, 2024
The navigate component is among the built-in components shipped in with React router version 6. It is a component similar to the useNavigate hook. Internally it uses useNavigate to redirect the location. The props passed to Navigate are the same as the arguments passed to the function returned by useNavigate.
While functional components in React support hooks, ES6 classes do not. Hence, when dealing with class-based React components, Navigate serves as a convenient alternative to useNavigate.
In this article, we are going to dive deeper into the Navigate component and how to efficiently use it to our advantage.
Now, go to the folder that houses the react project and install the react-router-dom package using the following command in the terminal:
# Using NPM
npm install react-router-dom@6
# Using Yarn
yarn add react-router-dom@6
# Using pnpm
pnpm add react-router-dom@6
Setting up React Router:
Before we use the components of React Router we need to set up its environment. To do this we need to import a top-level component and wrap our root component in it. To achieve this, BrowserRouter is used. If you are using React router version 6, wrap your root component in BrowserRouter as in the example below.
JavaScript
import React from "react";
import ReactDOM from "react-dom/client";
import { BrowserRouter as Router } from "react-router-dom";
import App from "./App";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<Router>
<App />
</Router>
</React.StrictMode>
);
JavaScript
import {BrowserRouter, Routers, Route} from "react-router-dom"
import Home from "./components/Home"
import Login from "./components/Login"
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={ <Home />} />
<Route path="/login" element = { <Login/> } />
// other routes
</Routes>
</BrowserRouter>
)
}
export default App;
Using the Navigate Component:
There are many built in components in React router version 6 and Navigate component is one of them. It is a wrapper for the useNavigate hook, and used to change the current location on rendering it.
Import the Navigate component to start using it:
import { Navigate } from "react-router-dom";
The Navigate component takes up three props:
<Navigate to="/login" state={{credentials: []}} replace = {true} />
- to – A required prop. It is the path to which we want to navigate
- replace – An optional boolean prop. Setting its value to true will replace the current entry in the history stack.
- state – An optional prop. It can be used to pass data from the component that renders Navigate.
The state can be accessed using useLocation like this:
const location = useLocation();
console.log(location.state);
// The default value of location.state is null if you don't pass any data.
The props you pass to the Navigate component are the same as the arguments required by the function returned by the useNavigate hook.
Example of Navigate Component
Example: In the following example, we’ve created a simple react application with a home page and a login page. When the user enters the details to login, a user is created and we navigate to the home page using the Navigate component.
JavaScript
// src/index.js
import React from "react";
import ReactDOM from "react-dom/client";
import { BrowserRouter as Router } from "react-router-dom";
import App from "./App";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<Router>
<App />
</Router>
</React.StrictMode>
);
JavaScript
// src/App.js
import { Route, Routes } from "react-router-dom";
import Home from "./components/Home";
import Login from "./components/Login";
function App() {
return (
<div className="App">
<Routes>
<Route path="/" element={<Home />} />
<Route path="/login" element={<Login />} />
</Routes>
</div>
);
}
export default App;
JavaScript
// src/components/Home.jsx
import React from "react";
const Home = () => {
return (
<div>
<h1>Home</h1>
</div>
);
};
export default Home;
JavaScript
// src/components/Login.jsx
import React, { useState } from "react";
import { Navigate } from "react-router-dom";
const Login = () => {
const [loginDetails, setLoginDetails] = useState({
email: "",
password: ""
});
const [user, setUser] = useState(null);
const handleChange = (e) => {
const { name, value } = e.target;
setLoginDetails((loginDetails) => ({
...loginDetails,
[name]: value
}));
};
const handleSubmit = (e) => {
e.preventDefault();
setUser(loginDetails);
};
return (
<div>
<form>
<label>
Email:
<input
type="email"
name="email"
value={loginDetails.email}
onChange={handleChange}
required
/>
</label>
<label>
Password:
<input
type="password"
name="password"
value={loginDetails.password}
onChange={handleChange}
required
/>
</label>
<button type="submit" onClick={handleSubmit}>
Login
</button>
</form>
{
user &&
<Navigate to="/" state={user} replace={true} />
}
</div>
);
};
export default Login;
Output:
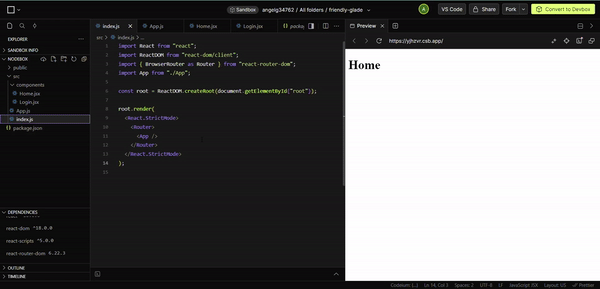
Live Display of Navigation
Share your thoughts in the comments
Please Login to comment...