useRoutes Hook in React Router
Last Updated :
05 Mar, 2024
React Router is a library that is used for handling navigation and routing in React applications. It provides a way to navigate between different components or pages while maintaining a single-page application (SPA) structure. One of the key features is the useRoutes hook. It allows you to define routes in a declarative manner. In this article, we will learn about useRoutes hook with its syntax and example.
What is useRoutes Hook ?
useRoutes hook is a part of the React Router library introduced in React Router v6 and is used to define and configure routes in a React application. It provides a flexible approach to defining routing configurations programmatically. It is a functional equivalent of <Routes>. You can define a route configuration JavaScript object that maps specific paths to React components.
Syntax of useRoutes Hook:
import { useRoutes } from 'react-router-dom';
const routeConfig = [
{ path: '/', element: <Home /> },
{ path: '/about', element: <About /> },
// Add more route configurations as needed
];
const App = () => {
const routeResult = useRoutes(routeConfig);
return routeResult;
};
Features of useRoutes Hook ?
- It allows you to define routes in a declarative manner using a configuration JavaScript object.
- It allows you to define nested routes.
- It can be defined with dynamic parameters such as product/:id.
- It supports wildcard routes using the * path for handling 404 errors.
Uses of useRoutes Hook
- Making Routes Flexible: It helps you create routes that can change based on what’s happening in your app. So, if your app needs to show different pages or components depending on what the user does or what data is available,
useRoutes
can handle that smoothly.
- Protecting Routes: If you’ve got parts of your app that should only be seen by certain users, like maybe admin pages or account settings,
useRoutes
can help you manage who gets to see what. You can use it to check if a user is logged in or has the right permissions before showing certain pages.
- Dealing with Tricky Routing: Sometimes, your app’s navigation needs to get a bit fancy. Maybe you’ve got pages within pages, or URLs that change based on what’s selected.
useRoutes
is your friend here too. It helps you handle these complex setups and keep your app’s navigation smooth and user-friendly.
Installation
To use a useRoutes hook, You have to install a React Router library by using following command:
npm install react-router-dom
Steps to Create Implement useRoutes Hook
Step 1: Create a React application using the following command:
npx create-react-app useroutes
Step 2: After creating your project folder(i.e. gfg), move to it by using the following command:
cd useroutes
Step 3: Install React Router library
npm install react-router-dom
Folder Structure:
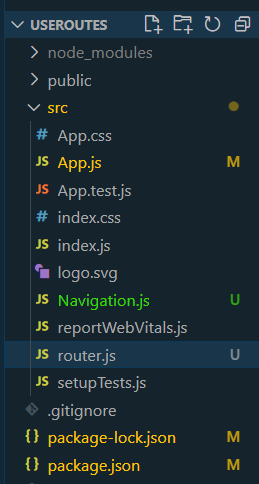
Dependencies: The updated dependencies in package.json file will look like.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.2",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: This below example demonstrate the use of useRoutes hook.
Javascript
import "./App.css" ;
import { BrowserRouter } from "react-router-dom" ;
import Router from './router'
export default function App() {
return (
<BrowserRouter>
<div className= "App" >
<h1 style={{ color: "green" }}>
GeeksForGeeks | useRoutes Example</h1>
<Router />
</div>
</BrowserRouter>
);
}
|
Javascript
import { Link, Outlet } from "react-router-dom" ;
export default function Navigation() {
return (
<>
<nav>
<ul>
<li>
<Link to= "/" >Home</Link>
</li>
<li>
<Link to= "about" >About</Link>
</li>
<li>
<Link to= "course" >Course</Link>
</li>
</ul>
</nav>
<Outlet />
</>
);
}
|
Javascript
import { useRoutes } from "react-router-dom" ;
import Navigation from "./Navigation" ;
const Home = () => <h1>Home Page</h1>
const About = () => <h1>About Page</h1>
const Course = () => <h1>Course Page</h1>
export default function Router() {
let element = useRoutes([
{
element: <Navigation />,
children: [
{ path: "/" , element: <Home /> },
{ path: "about" , element: <About /> }
]
},
{
element: <Navigation />,
children: [
{ path: "course" , element: <Course /> }
]
},
{
path: '*' ,
element: <div>404 Not Found</div>
},
]);
return element;
}
|
To run the application use the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
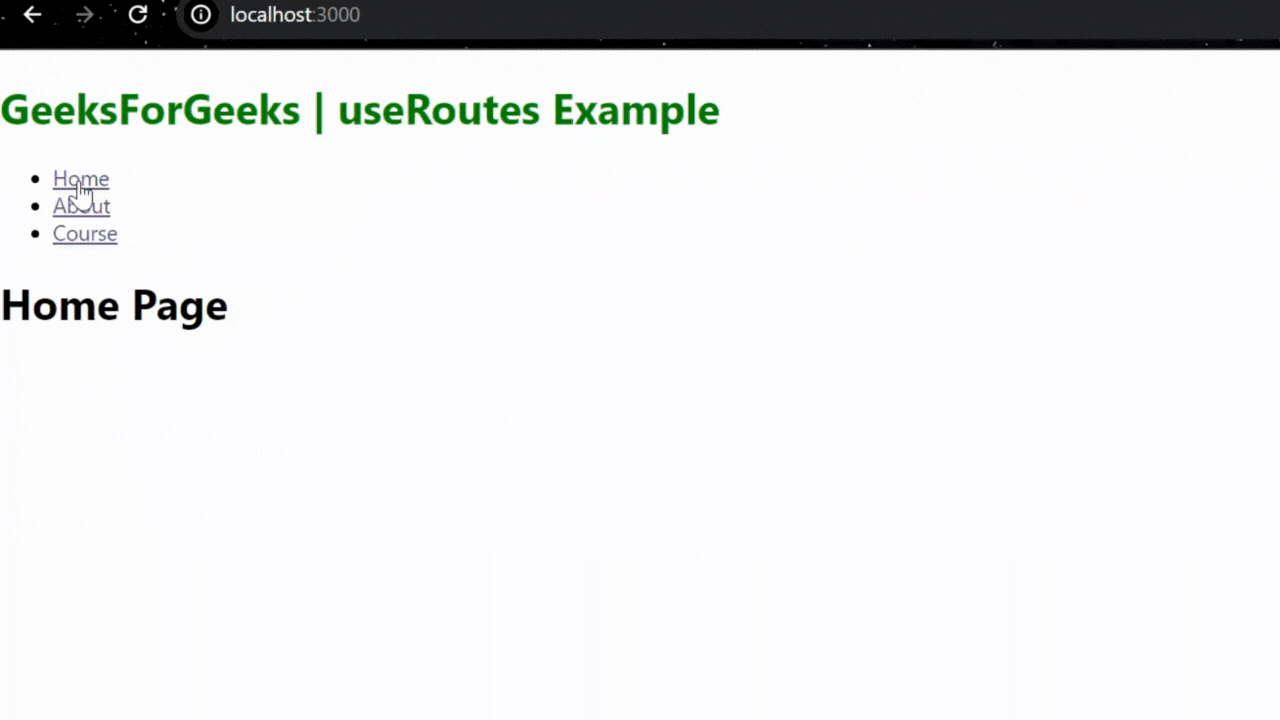
Share your thoughts in the comments
Please Login to comment...