Mastering JWT authentication in Express
Last Updated :
25 Jan, 2024
While creating any application it is very important to add authentication to ensure that only authorized users can access the protected resources. AÂ JSON Web Token (JWT)Â is a JSON object utilized to securely transmit information between two parties over the web. Primarily employed in authentication systems, JWTs can also facilitate secure data exchange. In this tutorial, we’ll see the process of implementing JWT-based authentication in Express.js.
Prerequisites
Steps to Implement Authentication
Step 1: Initialize your Node.js project
mkdir my-jwt-auth
cd my-jwt-auth
npm init -y
Step 2: Install the required dependencies.
npm install express mysql cors jsonwebtoken
Step 3: Set up your MySQL database
Create a MySQL database and table for storing user information using this query:
CREATE DATABASE jwt_db;
USE jwt_db;
CREATE TABLE `user` (
`id` int(11) NOT NULL,
`username` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL
)
Folder Structure:
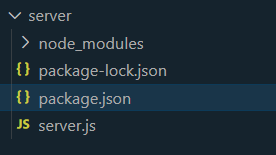
The updated dependencies in package.json file will look like:
"dependencies": {
"cors": "^2.8.5",
"express": "^4.18.2",
"jsonwebtoken": "^9.0.2",
"mysql": "^2.18.1"
}
Step 4: Set up your server
Javascript
const express = require( "express" );
const mysql = require( "mysql" );
const app = express();
const PORT = 3000;
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
const db = mysql.createConnection({
host: "localhost" ,
user: "root" ,
password: "1234" ,
database: "jwt_db" ,
});
db.connect((err) => {
if (err) {
console.error( "Error connecting to MySQL:" , err);
return ;
}
console.log( "Connected to MySQL" );
});
app.get( "/" , (req, res) => {
res.send( "Hello Geek!" );
});
app.post( "/register" , (req, res) => {
const { username, email, password } = req.body;
var sql = `INSERT INTO user (username, email, password)
VALUES( '${username}' , '${email}' , '${password}' )`;
db.query(sql, (err) => {
if (err) {
console.error( "Error in SQL query:" , err);
return res.status(500)
.json({ error: "Internal Server Error" });
} else {
res.status(200)
.json({ message: "Registered Successfully" });
}
});
});
app.listen(PORT, () => {
console.log( "App is running on port 3000" );
});
|
Step 5: To start the server run the following command.
node server.js
Step 6: Create a new collection in Postman and test the API.
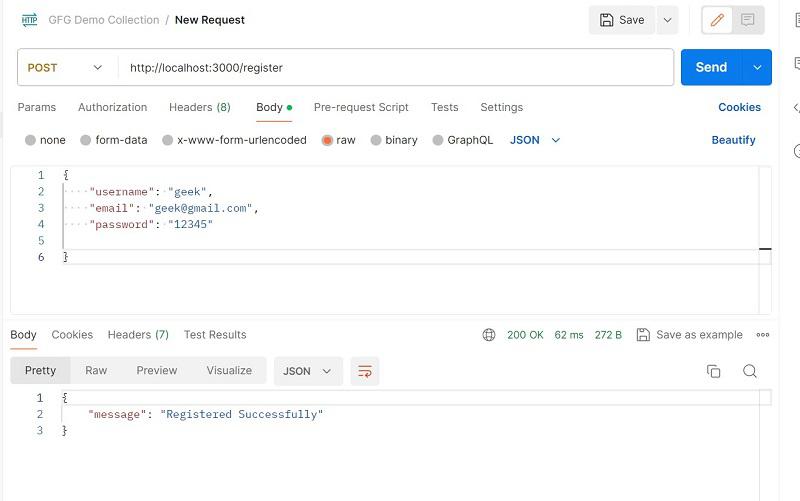
Register API testing using postman
Step 7: Create API for login and Implement JWT Authentication
app.post('/login', (req, res) => {
const { username, password } = req.body;
var sql = 'SELECT * FROM user WHERE username = ? AND password = ?';
db.query(sql, [username, password], (err, result) => {
if (err) {
console.error("Error in SQL query:", err);
return res.status(500).json({ error: 'Internal Server Error' });
}
if (result.length > 0) {
console.log("User found:", result);
res.json({ message: 'Login successful' });
} else {
console.log("User not found");
res.status(401).json({ error: 'Invalid credentials' });
}
});
});
Define a secret key for JWT. For example:
const secretKey = process.env.JWT_SECRET || 'your_secret_key';
Generate a JWT token when user found
const payload = { userId: 123, username: 'example_user' };
const secretKey = 'your_secret_key'; // Replace with a secure key
const expiration = '1h'; // Token will expire in 1 hour
const token = jwt.sign(payload, secretKey, { expiresIn: expiration });
console.log('JWT Token:', token);
Now, Test the login API using postman application.
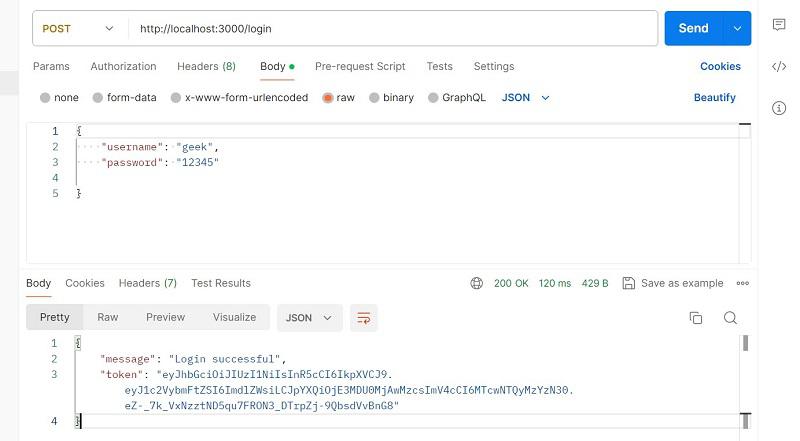
Login Success and token generated
Now, you have implemented jwt successfully.
Secure your routes using middleware
you can create a middleware function to verify the JWT token before allowing access to protected routes. Add this middleware to routes that require authentication.
Javascript
const authenticateJWT = (req, res, next) => {
const token = req.header( 'Authorization' );
if (!token) {
return res.status(401).json({ error: 'Unauthorized' });
}
jwt.verify(token.split( ' ' )[1], secretKey, (err, user) => {
if (err) {
return res.status(403).json({ error: 'Forbidden' });
}
req.user = user;
next();
});
};
app.get( '/protected' , authenticateJWT, (req, res) => {
res.json({ message: 'Access granted' });
});
|
Now , Test your proctered route using postman. Here’s an example of how the Postman request might look:
URL: http://localhost:3000/protected
Method: GET
Headers:
Key: Authorization
Value: Bearer <paste_the_copied_token>
Output Without Login:
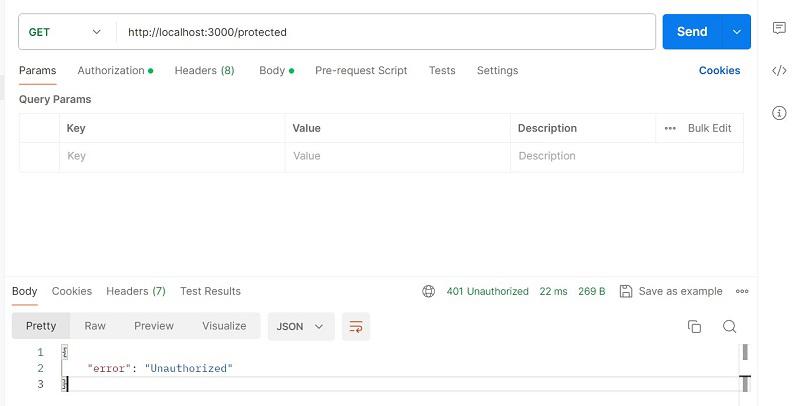
Protected routes without login
Output With Login:
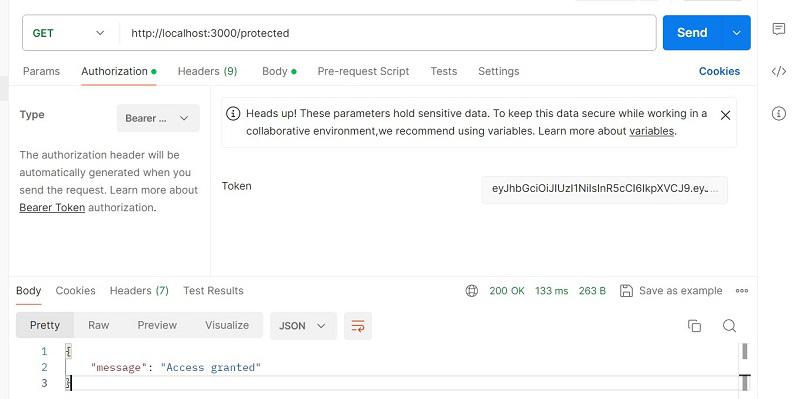
Protected routes with login
Share your thoughts in the comments
Please Login to comment...