How to handle routing with Hooks?
Last Updated :
22 Feb, 2024
You can handle routing with hooks using libraries like React Router. It allows you to define different routes for different components and manage navigation between them. React Router provides several hooks for handling routing within functional components, such as useHistory
and useNavigate
.
Key Features of React Router:
- Declarative Routing: React Router enables declarative routing using JSX, simplifying routing structure management.
- Hooks-based API: React Router offers hooks like useNaviagtion for navigation within functional components.
- Component-based Routing: Routes are defined with <Route> components, rendering corresponding components based on URL paths.
- Nested Routing: Supports nesting <Route> components for creating hierarchical route structures.
- Navigation: <Link> component facilitates navigation between routes by generating appropriate anchor tags.
- Dynamic Routing: Supports dynamic route matching and parameter access via hooks like useParams.
Example: Below is a basic example of handling routing with Hooks.
Javascript
import React from 'react' ;
import {
BrowserRouter as Router,
Route,
Routes,
Link,
useNavigate
} from 'react-router-dom' ;
function Home() {
return <h2>Home</h2>;
}
function About() {
return <h2>About</h2>;
}
function Contact() {
const navigate = useNavigate();
const handleClick = () => {
navigate( '/about' );
};
return (
<div>
<h2>Contact</h2>
<button
onClick={handleClick}>
Go to About
</button>
</div>
);
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to= "/" >Home</Link>
</li>
<li>
<Link to= "/about" >About</Link>
</li>
<li>
<Link to= "/contact" >Contact</Link>
</li>
</ul>
</nav>
<Routes>
<Route path= "/"
element={<Home />} />
<Route path= "/about"
element={<About />} />
<Route path= "/contact"
element={<Contact />} />
</Routes>
</div>
</Router>
);
}
export default App;
|
Output:
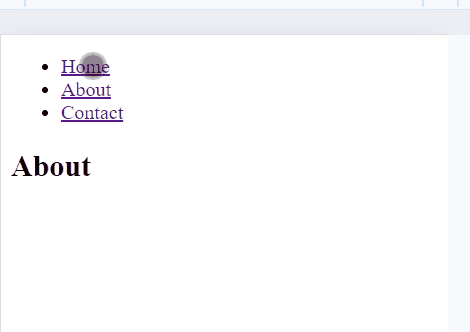
Output
Share your thoughts in the comments
Please Login to comment...