Top 10 JavaScript Concepts for Node.js Developers
Last Updated :
12 Feb, 2024
In 2024, JavaScript remains the most important part of web development, with its significance in Node.js development reaching new heights. As Node.js evolves, developers need to stay updated on the latest JavaScript concepts and principles for creating efficient, scalable, and secure web applications.
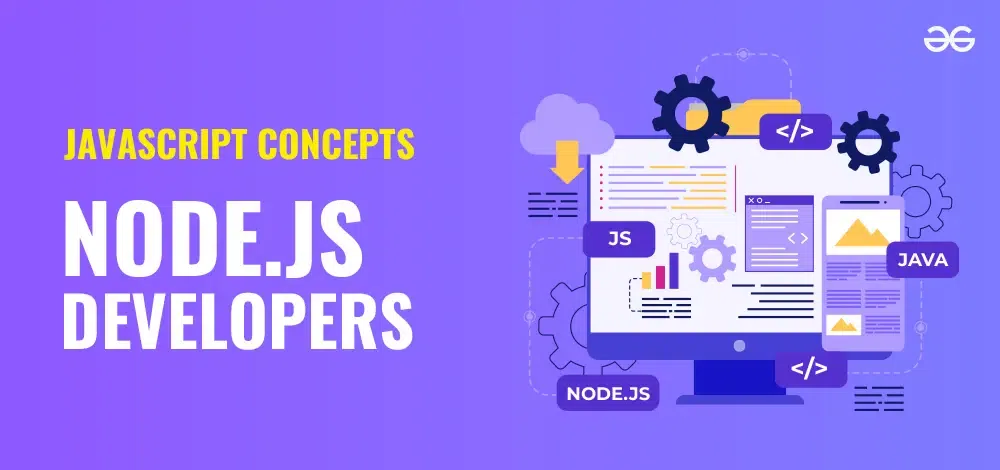
In this article, we will dive into the Top 10 JavaScript concepts essential for Node.js developers in 2024. We will be covering areas from asynchronous programming to security best practices, these concepts simply underpin modern Node.js development. Whether you’re an experienced Node.js developer or just a beginner, mastering these concepts will enrich your skills which enables you to build robust applications that align with the dynamic web environment of today.
Who are Node.js Developers?
Node.js developers are typically responsible for designing the backend of any websites and applications. They leverage Node.js which is a tool that enables server-side JavaScript coding to create the server-side components of web applications. Their role generally involves managing data by ensuring proper handling of user requests, and the overall functionality of the website runs smoothly.
These developers possess expertise in building fast and scalable applications. They often collaborate with other developers and teams in order to create websites that are capable of handling high user loads without compromising their performance. In essence, they are the unsung heroes working behind the scenes to ensure smooth operations across the web.
Top 10 JavaScript Concepts for Node.js Developers in 2024
Now let’s move on to the our main topic and discuss the most important topics that are needed to become a Node.js Developer.
1. Asynchronous JavaScript
In JavaScript, asynchronous code generally enables tasks to run independently that prevents one task from waiting for another to finish. This is generally very important for tasks like fetching data from a server, where waiting for each request to complete would slow down the program.
In Node.js, asynchronous code is particularly important for handling multiple requests simultaneously without blocking the program’s execution. This capability allows Node.js developers to write code that can manage numerous tasks concurrently which results in faster and more responsive applications.
2. Promises and Async/Await
- Promises:
- Promises are a way to handle asynchronous operations in JavaScript.
- They represent a value that may not be available yet but will be resolved at some point in the future.
- They have methods like
then()
and catch()
to handle success and error conditions respectively.
- Promises are used to avoid “callback hell” and make asynchronous code more readable and manageable.
- Async/Await:
- Async functions in JavaScript generally provide a way to write asynchronous code that appears synchronous.
- By using the
async
keyword before a function declaration and await
inside the function to wait for a Promise to resolve, developers can simply make asynchronous code more readable and manageable, particularly when handling multiple asynchronous operations.
- This approach basically allows developers to write code that resembles synchronous code in appearance but should behaves asynchronously which enhances overall code readability and maintainability.
3. Modules and Modularization
In Node.js, a modular system is used that treats each file as a separate module. Modules are like containers that hold related code that basically makes it reusable and easier to manage. Node.js uses CommonJS as its module format which simply allows developers to import modules using require()
and export functionality from a module using module.exports
. This modular approach helps keep code organized and maintainable
- ES6 Modules:
- With the arrival of ES6 (ECMAScript 2015), JavaScript eventually gained the support for a native module system.
- ES6 modules generally utilize the
import
and export
statements to specify dependencies and export functionality. This approach basically offers a modern and standardized method for managing different modules in JavaScript which complements the CommonJS format used in Node.js.
- Benefits of Modularization:
- Breaking down the code into smaller, reusable parts with the mechanism of modularization makes it easier to maintain and perform tests.
- It also promotes a clear separation of concerns, with each module focusing on a specific task or functionality.
- Additionally, modules can be shared across different parts of an application or even between different applications which encourages code reuse and consistency across projects.
- Using Modules in Node.js:
- In Node.js, we basically import modules into our code using the
require()
function.
- Once imported, we can then utilize the functionality exported by the module in our own code.
- Using this modular architecture, Node.js generally provides a broad ecosystem of built-in and third-party modules that can be smoothly integrated into our applications.
- Best Practices:
- Keeping modules small and focused on specific responsibilities is generally the best practice in Node.js development.
- Clear interfaces and well-defined boundaries should characterize modules that makes them easier to comprehend and maintain.
- Effectively using these modules can result in a more organized and scalable codebase for Node.js applications.
4. Error Handling
Error handling is a very critical and important part of writing a robust and reliable Node.js application. It generally involves dealing with faults or unexpected occurrences that may occur during the execution of code. Proper error handling usually ensures that the application can effectively handle errors and recover from them without crashing.
In Node.js, errors can occur due to variety of reasons like network problems, database failures, or coding mistakes. To manage these issues effectively, developers generally use different strategies such as try/catch blocks for synchronous code and error-first callbacks for asynchronous operations. These methods usually help ensure the application can handle errors effectively and continue functioning smoothly..
Best Practices for Error Handling in Node.js
- Use Error Objects: Whenever you encounter an error, it is very important to create an Error object that describes the problem with details like an error message and stack trace. This information is basically very important for debugging and troubleshooting, as it simply helps you to understand what went wrong and where.
- Handle Errors Asynchronously: Since Node.js is typically famous for its asynchronous operations, it is crucial to handle errors in asynchronous code the right way. Typically, this means passing an error object as the first argument to a callback function or using Promises to manage errors in a series of asynchronous tasks.
- Centralized Error Handling:It is a great practice to centralize your error handling in one spot, like a middleware function in an Express.js application. This generally makes sure that you handle errors consistently across your entire program.
- Graceful Degradation: If an error isn’t very critical to how your application works, it’s often best to handle it gracefully instead of letting it crash the whole thing. By this way, your application can keep running smoothly even if there are minor issues involves.
- Logging Errors: Also, by logging errors to a file or monitoring system, you can gain valuable insights into how your application is performing. This can be really helpful in spotting any recurring issues and making your application more stable overall.
5. Event Emitters and Event Loop
Node.js generally follows the event-driven architecture is a fundamental concept that allows developers to build scalable and efficient server applications. Two key components of this architecture are event emitters and the event loop.
- Event Emitters:
- An event emitter is a Node.js object that basically can emit named events.
- These events can be custom-defined which simply allows developers to create their own event-driven logic.
- In Node.js, event emitters are typically used to handle asynchronous events like HTTP requests, file system actions, and database queries.
- Event Loop:
- The event loop typically serves as the foundation of Node.js’ asynchronous, non-blocking I/O model..
- When an event occurs, it continuously checks for it and executes the related callback routines.
- This feature generally allows Node.js to handle multiple concurrent operations efficiently without blocking the execution of other tasks.
- Non-Blocking I/O:
- Non-blocking I/O is commonly used by Node.js to manage I/O tasks such as reading from files or performing network requests..
- When an I/O operation is started, Node.js doesn’t wait for it to complete but, it continues to executing other tasks.
- When the I/O operation is finished, an event is emitted, and the associated callback function then executed by the event loop.
6. Streams and Buffers
In Node.js, streams and buffers are very important for handling data in an efficient manner, mostly in scenarios when we are working with large datasets or performing I/O operations.
- Buffers:
- In Node.js, buffers are used to represent binary data..
- They are basically the instances of the Buffer class, which is a global object in Node.js.
- Buffers are generally very important for dealing with binary data, such as reading from or writing to files, as well as network protocols.
- Streams:
- Streams are objects that allows reading or writing data in a continuos manner.
- They are instances of the stream module in Node.js that can be readable, writable, or both.
- Streams are typically used for handling I/O operations efficiently, especially at the time of dealing with large files or network data.
Benefits of Streams and Buffers
- Efficiency: Streams and buffers are very important for efficiently manage data, particularly when dealing with large volumes of it. They typically enables the handling of data in manageable chunks by optimizing performance.
- Memory Management: Buffers play a key role in efficient memory management by storing data in smaller segments, rather than all at once. This approach basically helps in better utilization of memory resources.
- Asynchronous Operations: Streams are essential for handling asynchronous I/O operations in which data is processed as it becomes available. This approach generally avoids the need to wait for the entire dataset to be loaded into memory which enhances the efficiency in handling asynchronous tasks.
7. JavaScript Prototype and Inheritance
JavaScript Prototypes
- In JavaScript, every object has a prototype. The prototype is an object from which other objects inherit properties. Whenever you call a property or method on an object, JavaScript first checks if that property or method exists on the object itself. If it doesn’t, it looks at the prototype of the object, and if the property or method is found there, it is used.
- Prototypes are used to implement inheritance in JavaScript which allows objects to inherit properties and methods from other objects.
Prototypal Inheritance
- JavaScript uses prototypal inheritance rather than classical inheritance that are found in languages like Java or C++. In prototypal inheritance, objects inherit directly from other objects. This generally means that objects can be created based on other objects, and any type of changes made to the prototype object will be reflected in all its instances.
- Prototypal inheritance is a very important feature in JavaScript that basically enables flexible and dynamic object interactions.
Advantage of JavaScript Prototype and Inheritance
- Knowing how prototypes and inheritance generally work in JavaScript can help Node.js developers to write cleaner and more maintainable code by utilizing inheritance to create reusable components.
- Understanding prototypal inheritance can also help with debugging and troubleshooting code since it explains how objects are related and how attributes and methods basically are passed down.
8. Authentication and Authorization
Authentication in Node.js verifies the identity of users or systems accessing an application. It generally involves different types of strategies like username/password authentication, JSON Web Tokens (JWT), or OAuth for secure access. Proper authentication is very important for preventing unauthorized access and ensuring user privacy.
Authorization in Node.js determines what actions a user is allowed to perform after authentication. It enforces access control rules which is based on user roles or permissions. By using middleware for authorization, Node.js applications can protect sensitive resources and maintain a secure environment.
9. Testing & Debugging
Testing and debugging are like checking your work and fixing mistakes while writing code in JavaScript and Node.js. They basically help ensure that your code works correctly & doesn’t have any unwanted hidden problems.
Testing
- Testing generally involves running your code with different inputs in order to see if it behaves as expected.
- In JavaScript, you can write tests using different frameworks like Jasmine, Mocha, or Jest, which provide tools for defining tests and checking if the code produces the expected results or not.
- Testing typically helps to catch errors early that ensures that changes to your code don’t break existing functionality, and makes your code more robust and reliable.
Debugging
- Debugging is generally the process of identifying and resolving errors in your code.
- In JavaScript, you can utilize the developer tools of the browser or Node.js’s built-in debugging features to navigate throughout your code in order to examine variables, and pinpoint issues.
- Effective debugging is ver essential for finding the reasons behind unexpected behavior or errors in your code which allows you to rectify them and enhance your code’s reliability.
10. Performance optimization
Performance optimization in JavaScript and Node.js is generally about improving the overall speed and efficiency of your code. It basically involves identifying and fixing slow parts of your application that are lagging behind, such as inefficient algorithms or resource-heavy operations. By using tools to measure performance and applying techniques like optimizing I/O operations and caching data, you can eventually make your code run faster and use fewer resources. This not only enhances your applications’ overall efficiency but also assures that they can manage additional users and data without slowing down.
Conclusion
In conclusion, JavaScript is very important for web development, particularly in Node.js. Understanding key concepts like asynchronous programming, error handling, and authentication is generally very important for building efficient and secure applications. These concepts basically act as building blocks of modern Node.js development which allows developers to create strong, adaptable applications for the web’s changing needs. Whether you are new to Node.js or an experienced developer, learning these concepts is very important for success in web development’s dynamic world.
Share your thoughts in the comments
Please Login to comment...