An API (Application Programming Interface) is essentially an intermediary, built within a set of protocols that allows communication and functionality between two software applications. A well-functioning web application is built on the base of multiple APIs each serving a unique purpose.
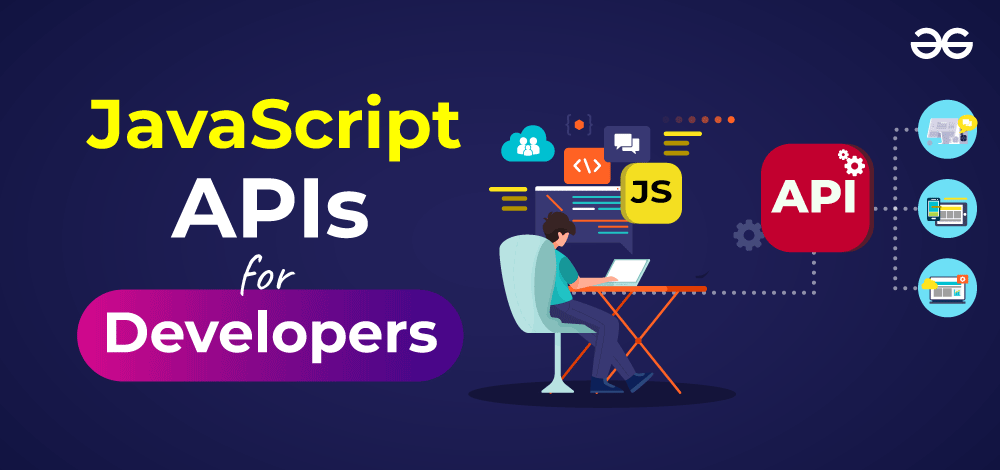
Javascript APIs are those that use Javascript code to establish this communication. As they play a crucial role in web development due to their vast capabilities and the widespread use of the language in the software development field, Web APIs are typically used with JavaScript. While there exist a good amount of APIs that can be employed by a developer, the below article compiles a list of the Top 10 JavaScript APIs.
Top Frontend JavaScript APIs For Frontend Developers
These APIs work on the client side of the application and are invoked to make the user experience seamless and interactive. These APIs are crucial for web developers because they let them fetch data, update what you see on the screen, and integrate with other services, making websites more dynamic and interactive.
1. JSONPlaceholder
JSONPlaceholder is a REST API which is a fantastic resource for developers to practice API interactions without setting up servers or databases. It provides dummy data in JSON format and allows easy access to posts, comments, users, todos, and albums. Using JavaScript’s fetch API, you can make HTTP requests to fetch, retrieve, create, update, and delete data.
We will learn how to perform basic CRUD operations using this API with practical code examples in JavaScript.
How to Use?
The fetch() method enclosed with the relevant URL can be used to call the JSONPlaceholder. The axios library (containing .get() and .post() methods) can also be used. We are making an HTTP request to an external API, fetch JSON data, and then log the retrieved data to the console.
Example:
Javascript
fetch( '...' )
.then(response => response.json())
.then(data => {
console.log(data);
})
. catch (error => console.error( 'Error fetching data:' , error));
|
Sample Output:
[
{
"userId": 1,
"id": 1,
"title": "Post Title",
"body": "Post content..."
},
{
"userId": 1,
"id": 2,
"title": "Another Post",
"body": "More content..."
},
// Additional posts...
]
The output of the provided code will be an array of posts fetched from the JSONPlaceholder API.
A few APIs that work similarly to the JSONPlaceholder are:
- ReqRes REST API
- Mockaroo API
- Postman API
- WireMock library
- JSON-Server
2. The MovieDB API
The MovieDB API is a RESTful web service that provides access to a comprehensive database of movies, TV shows, and actors. Performs HTTP operations to fetch information about movies, search for specific titles, retrieve images, and get details about actors and their filmography as well as conduct image retrievals.
How to Use?
API KEY: A unique identifier assigned to a user/project that authorizes the use of software, permits the requests sent from one software to another, and protects the project from hacking.
MovieDB API requires every developer to have an API key to be allowed access to its database. Hence, once the API key is attained we can use the fetch() method to make requests for Movie, actor, or related data. Once we receive a response from the API, we log the data into the console.
You can use the following link to generate your API key here.
In this code, we will use MoviesDB API to fetch the data of the Spiderman: Across The Spider-Verse movie using the API key and get the movie’s complete data like the overview, language, rating, popularity and etc.
Example:
Javascript
const options = {
method: 'GET' ,
headers: {
accept: 'application/json' ,
Authorization: '...'
}
};
fetch( '...Spiderman: Across The Spider-Verse' , options)
.then(response => response.json())
.then(response => console.log(response))
. catch (err => console.error(err));
|
In the above code, the user with the API key sends a request to discover a movie and gets the data of Spiderman: Across The Spider-Verse as a response.
Output:
{
"id": 569094,
"original_language": "en",
"original_title": "Spider-Man: Across the Spider-Verse",
"overview": "After reuniting with Gwen Stacy, Brooklyn's full-time, friendly neighborhood Spider-Man is catapulted across the Multiverse, where he encounters the Spider Society, a team of Spider-People charged with protecting the Multiverse's very existence. But when the heroes clash on how to handle a new threat, Miles finds himself pitted against the other Spiders and must set out on his own to save those he loves most.",
"popularity": 1281.799,
"poster_path": "/8Vt6mWEReuy4Of61Lnj5Xj704m8.jpg",
"release_date": "2023-05-31",
"title": "Spider-Man: Across the Spider-Verse",
"video": false,
"vote_average": 8.5,
"vote_count": 2241
}
Alternatives to the TMDB API include:
- Letterboxd API
- OTT Details API
- Utelly API
- GoWATCH API
3. Responsive Voice API
With this API you can integrate text-to-speech functionality into web applications and make the user experience enriching. It supports 51 languages and provides around 158 voices to choose from. This API makes use of HTTP requests such as GET, POST, etc. to send requests to the API endpoint and fetch that data and signals.
How to Use?
This API requires a stable internet connection to conduct its text-to-speech conversion as the functionality is dependent on the ResponsiveVoice server.
Let’s see how we can use ResponsiveVoice API to convert text-to-speech in real time. We create an element ‘script’ to link the original URL of the ResponsiveVoice API attached with the key and then the variable ‘textToSpeak’ is added that contains the text to be converted.
Example:
Javascript
javascript
var script = document.createElement( 'script' );
script.src = '...?key=YOUR_API_KEY' ;
document.head.appendChild(script);
function speak(text) {
responsiveVoice.speak(text);
}
var textToSpeak = 'Hey there! This is the ResponsiveVoice API converting text-to-speech.' ;
speak(textToSpeak);
|
Alternate tools that function similarly to the Responsive Voice API are
- Amazon Polly
- Microsoft Azure Speech Service
- Google Cloud Text-to-Speech
- IBM Watson Text to Speech
4. Count API
The Count API is a simple yet powerful tool for counting and tracking events. It allows you to create numeric counters and send a request to the API to increment and decrement the count and retrieve the count value as well as perform analytics on the collected data.
It works on the principle of IaaS – Integer as a Service.
How to Use?
This API too requires the generation of an API key before use can be authorized. Once generated, you can use JavaScript functions to retrieve the required data, analyze the site stats, etc.
Let’s see how CountAPI can be used to measure the number of site visits that have occurred on a webpage. The real-time output will be subjective to the webpage specified and the hits it has received.
Example:
Let’s say you want to display the number of page views a site received. You can use the following code to deduce that data.
HTML
< div id = "visits" >...</ div >
|
Use the following code to import the API into JavaScript and generate the result value
Javascript
import countapi from 'countapi-js' ;
countapi.visits().then((result) => {
console.log(result.value);
});
|
Output:
15
The above output shows that the Count API has been imported successfully and that the page has a total of 15 visits as recorded by the API. The real-time output will be subjective to the webpage specified and the hits it has received.
its
The following platforms and/or data visualization tools can be used to achieve the same feats as Count API;
- Count.io.
- Tableau
- Jakub Linowski
- Juggler.dev
- AbTest.io
5. Quotes API
The Quotes API provides a collection of famous quotes from various authors and categories. It is capable of fetching random quotes, fetching information about authors, or searching for quotes based on specific criteria.
How to Use?
HTTP requests are coded into the program and sent to the API’s endpoint by the developer to use Quotes API. You can program varying iterations of requests like specific quotes, quotes from a certain author, or a completely random quote.
This included with the user’s API key is enough to integrate Quotes API into the code.
We shall see in the below code how we use CountAPI to generate a quote and its author’s name at random.
Example:
Javascript
function getQuote() {
return fetch( '...' ).then(response => {
return response.json()
}).then(jsonResponse => {
if (!jsonResponse.length) {
return []
}
return jsonResponse[Math.floor(Math.random() * jsonResponse.length)]
})
}
getQuote().then(quote => {
const text = quote.text;
const author = quote.author;
console.log(text, author)
})
|
The output in the console will display a randomly fetched quote along with its author and display the category as well.
Sample Output:
[
{
Life is a learning experience, only if you learn. Yogi Berra
}
]
Quotes API holds a sizeable bank of categories to enlist quotes. You can view the list as well as try generating a quote of your own here.
The following APIs function similarly:
- Programming Quotes API
- Random Famous Quotes API
- They Said So API
- Advice Slip API
- Forismatic API
- FavQs API
Top Backend JavaScript APIs For Backend Developers
Backend APIs are used on the server side of web applications, handling server-side logic and interactions with databases or external services. These APIs facilitate tasks such as accessing image libraries, enabling native sharing functionality, making HTTP requests to external APIs, retrieving the user’s location, or handling payment transactions.
1. Unsplash API
The Unsplash API is a powerful tool that allows developers to access a vast collection of high-quality images to integrate into their applications. You can use it to retrieve and access images by sending requests. This JSON API returns the URLs of the photos along with their metadata and works completely royalty-free.
How to Use?
This API also requires the developer to request an API Key from Unsplash. The API key can then be used to authorize HTTP requests for images and their related data.
In this example, the fetch() function is used to send a GET request to the /photos endpoint of the Unsplash API. The metadata of the pictures will be returned followed by the pictures.
Example:
Javascript
javascript
fetch( '...?client_id=YOUR_ACCESS_KEY' )
.then(response => response.json())
});
|
The following platforms can be considered alternatives to the Unsplash API. Most of them provide their APIs as well.
- Flickr
- Pexels
- Pixabay
- Getty Images
- Burst
- Openverse
2. Web Share API
The Web Share API easily implements the native sharing functionality on the user’s device directly from a web application i.e. the web application is permitted to use the sharing capabilities built into the user’s device. It uses the Navigator.Share() method to share content. This contains an object containing all the data to be shared, such as the text containing the message, URLs, and even files.
How to Use?
You must first authenticate your access by providing your token (Web Share API Key). Then, the navigator.share property checks if Web Share API is supported by the browser.
If supported, you can employ the Navigator.canShare() method or the Navigator.canShare() method to prompt the native sharing interface.
Let us use the Navigator.Share() method to share a link to a Coding Environment with another user.
Example:
Javascript
if (navigator.share){
const shareData = {
title: "Check out this Website!" ,
text: "Practice coding in any language you need." ,
};
const btn = document.querySelector( "button" );
const resultPara = document.querySelector( ".result" );
btn.addEventListener( "click" , async () => {
try {
await navigator.share(shareData);
resultPara.textContent = "Successfully shared" ;
} catch (err) {
resultPara.textContent = `Error: ${err}`;
}
}
});
}
else {
console.log( 'The browser you’re currently using does not support Web Share API' );
}
|
Output:
The above example generates a share button and the following message is shared to the recipient on their device once the share button is clicked, provided Web Share API is supported by the browser.
Check out this Website!
Practice coding in any language you need.
https://practice.geeksforgeeks.org/
If the process is carried out successfully the developer receives the following message:
Successfully shared
While the alternatives for the Web Share API are currently few and far between the following methods can be used to get similar results:
- Custom Sharing Functionality
- Social Media SDKs (Software Development Kits)
3. The Fetch API
The Fetch API is an interface that allows asynchronous HTTP requests to servers from web browsers. It is a better, more powerful, and more flexible replacement for the older XMLHttpRequest (XHR) object. It supports HTTP methods like GET, POST, PUT, DELETE, etc. Methods like .json(), .text(), .blob(), etc. can be used to extract the response.
How to Use?
Fetch works on the concept of Promises and Responses.
The Fetch API is invoked by calling the fetch() method, which returns a Promise that resolves into a Response object.
You can call the fetch function and specify the URL. Promises can also be used for other functions like setting request headers, handling response data, handling errors, etc.
Let us see how we can request JSON data from a URL using the Fetch API. Once the Request is sent, the response is recorded in .json() form and logged into the console.
Example:
Javascript
javascript
fetch( '...' )
.then(response => response.json())
.then(data => console.log(data))
. catch (error => console.error(error));
|
While the given example is a simple data request, complex requests can also be made using the Fetch API. Methods such as PUT, POST, and DELETE can be used to achieve that. Fetch also allows you to customize your request headers.
You might note that most of the examples quoted in this article invoke the fetch() method, i.e. use the Fetch API.
Libraries and platforms that work as alternates include:
- Got
- Axios
- SuperAgent
- Requestify
4. The Geolocation API
This API determines the location of the device on the user’s end. It accesses the device’s GPS and returns the latitude, longitude, and altitude. It also states the accuracy of the retrieved location and the timestamp of when the location was retrieved.
How to Use?
The navigator.geolocation object available in JavaScript is employed to use the Geolocation API. The presence of this object indicates whether or not Geolocation services are available.
You can employ the getCurrentPosition() and watchPosition() methods to access the services of this API.
The below code provides the coordinates – latitude, and longitude – of the user device’s current location:
Example:
Javascript
function geoFindMe() {
const status = document.querySelector( "#status" );
const mapLink = document.querySelector( "#map-link" );
mapLink.href = "" ;
mapLink.textContent = "" ;
function success(position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
status.textContent = "" ;
mapLink.href = `.../${latitude}/${longitude}`;
mapLink.textContent = `Latitude: ${latitude} °, Longitude: ${longitude} °`;
}
function error() {
status.textContent = "Unable to retrieve your location" ;
}
if (!navigator.geolocation) {
status.textContent = "Geolocation is not supported by your browser" ;
} else {
status.textContent = "Locating..." ;
navigator.geolocation.getCurrentPosition(success, error);
}
}
document.querySelector( "#find-me" ).addEventListener( "click" , geoFindMe);
|
Output:
Latitude: 12.9820049 °, Longitude: 80.2016977 °
The above output is generated after the successful compilation of the code and the hyperlink is activated with an openstreetmap.org URL that showcases the Location on the map.
Here are a few APIs and platforms that can act as alternatives for the Geolocation API:
- IP Geolocation API
- Google Places API
- Mapbox
- OpenStreetMap
- HERE Geocoding and Search API
- MaxMind GeoIP2
5. Payment Request API
The Payment Request API integrates payment methods into the application. It fetches the payment method, details, shipping info, and status of payment of a user. It supports varying payment methods from debit and credit cards to digital wallets and even includes UPIs which makes it one of its kind.
How to Use?
The Payment Request API is invoked by the use of the following constructor:
PaymentRequest(methodData, details)
Example:
Javascript
async function doPaymentRequest() {
try {
const request = new PaymentRequest(methodData, details, options);
const response = await request.show();
await validateResponse(response);
} catch (err) {
console.error(err);
}
}
async function validateResponse(response) {
try {
const errors = await checkAllValuesAreGood(response);
if (errors.length) {
await response.retry(errors);
return validateResponse(response);
}
await response.complete( "Payment successful" );
} catch (err) {
await response.complete( "Failed" );
}
}
doPaymentRequest();
|
Output:
Payment successful
The following platforms can be seen as alternatives to the Payment Request API:
- Stripe
- PayPal
- Braintree
- Authorize.Net
- Square
Conclusion
This summarizes the list of the top 10 Javascript APIs frontend and backend developer when building web applications. Familiarising one’s self with these APIs will aid in incorporating immersive functionalities, engaging features, and interactive elements into their applications, thereby providing an enriching experience to the end user.
FAQs on JavaScript APIs
Q1. What are JavaScript APIs, and why are they important for developers?
Answer:
JavaScript APIs (Application Programming Interfaces) are sets of functions and methods that allow developers to interact with and utilize the features and capabilities of various technologies, libraries, and platforms. They are important to developers as they are essential tools for building dynamic and feature-rich web applications.
Q2. What are the Top Frontend Javascript APIs?
Answer:
The Top Frontend javascript APIs are as follows:
- JSONPlaceholder
- MovieDB API
- Responsive Voice API
- Count API
- Quotes API
Q3. What are the Top Backend Javascript APIs?
Answer:
The Top Frontend javascript APIs are as follows:
- Unsplash API
- Web Share API
- Fetch API
- Geolocation API
- Payment Request API
Share your thoughts in the comments
Please Login to comment...