Javascript is an object-oriented language that is lightweight and used for web development, web applications, game development, etc. It enables dynamic interactivity on static web pages, which cannot be done with HTML, and CSS only. Javascript is so vast that even mid-level professionals find it difficult to work and that might be possible because of the demand. Having different skill sets apart from writing functions, or class is the real game changer that helps in uplifting your career.
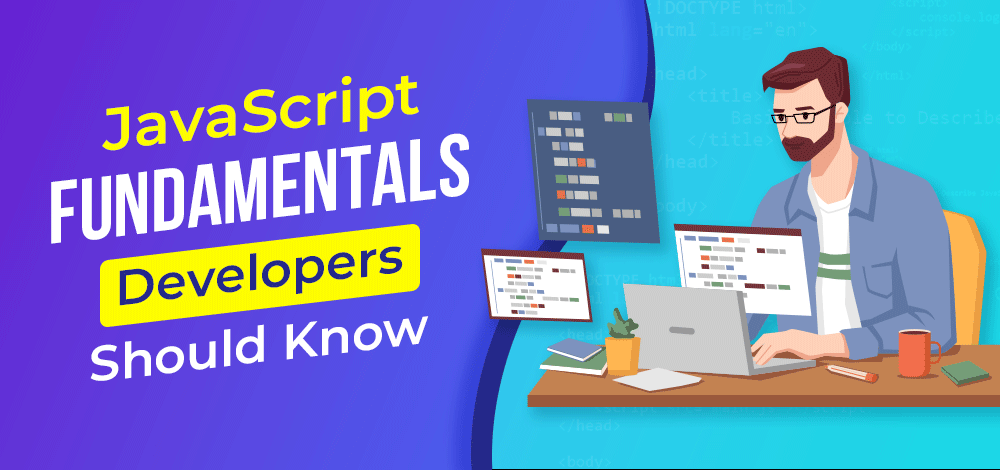
Having so many benefits of learning JavaScript and also being one of the most important and most-demanding languages, we’re going to discuss some of the Useful JavaScript fundamentals developers should know in 2023.
Top 10 JavaScript Fundamentals That Every Developer Should Know
1. Type Conversion
Mostly five kinds of datatypes are used in Javascript. They are:
- Number: This datatype represents numeric type values. Example: 100, 13
- String: This is made of characters. It is always kept in double quotation (“ ”). Example: “GeeksForGeeks”, “JavaScript”
- Boolean: In these datatypes, there are only two values true and false.
Example: true, false
- Undefined: It can only represent an undefined value which means it is not defined.
Example: undefined
- Object: It is basically data collection which is represented by key-value pairs.
Example: const person={
Name:” Ram”,
Age:30,
Language:” JavaScript”
}
Three types of functions we used in javascript for converting datatypes.
- Number()
- String()
- Boolean()
Moving ahead, we will briefly discuss these in-build functions.
A. Typecast to Number: The Number () function is used when we convert the given input value to the number type. But, If we want to convert the given input into an int or float type, then we have to use the parseInt() function to convert it into an int type and the parseFloat() function to convert it into a float type.
Syntax of the typecasting in number type:
Javascript
console.log( "Before conversion String type 1 and after conversion Number type" ,Number( "1" ));
console.log( "Before conversion Boolean type true and after conversion Number type" ,Number( true ));
|
Output
Before conversion String type 1 and after conversion Number type 1
Before conversion Boolean type true and after conversion Number type 1
B. Typecast to String: In javascript String is considered as an object. The String () function is used when we want to convert the given input value to the string type. If we pass any character, number, etc in this function then it will be converted into a String.
Syntax of the typecasting in String type:
Javascript
console.log( "Before conversion Number type 1 and after conversion String type" ,String(1));
console.log( "Before conversion Boolean type true and after conversion String type" ,String( true ));
|
Output
Before conversion Number type 1 and after conversion String type 1
Before conversion Boolean type true and after conversion String type true
C. Typecast to Boolean: Boolean() function is used when we need to convert the given input value to boolean type.
Syntax of the typecasting in Boolean type:
Javascript
console.log( "Before conversion Number type 1 and after conversion Boolean type" ,Boolean(1));
console.log( "Before conversion String type true and after conversion Boolean type" ,Boolean( "false" ));
|
Output
Before conversion Number type 1 and after conversion Boolean type true
Before conversion String type true and after conversion Boolean type true
2. Loops
If you want to print numbers from 1 to 10 then you have to write the same code 10 times again and again. But if you want to print numbers from 1 to 1000 that is impossible to write. Here is the need for a JavaScript loop.
Three types of loops are primarily used in javascript are:
- For Loop
- While Loop
- Do-while Loop
A. for Loop
There are three things in for loop. First is an initial expression, then a condition, and at last an updated expression. In the initial expression, we initialize or declare a variable and it executes for only one time. Condition is checked in every iteration. The block of code inside the for loop is executed when the condition statement is true. If the condition is false then the loop will be terminated. The update expression is used to update the initial expression in every iteration.
Syntax:
for (initial expression; condition; update expression) {
//code block of the loop;
}
Example
Javascript
for (let num = 0; num < 5; num++) {
console.log(num);
}
|
B. while Loop
There is a condition in the while loop if that condition is false then the loop will be terminated, if the condition is true then the execution of the block of code inside the while loop will be continued.
Syntax:
while(condition){
//code block of the loop;
}
Example:
Javascript
let i = 1;
while (i <= 5) {
console.log(i);
i += 1;
}
|
C. do-while Loop
In the do-while loop, a block of code is executed at first then the condition is evaluated. If the condition is true, then only the code of the block is executed again; if the condition is false, then the loop will be terminated.
Syntax:
do{
//code block of the loop;
}while(condition)
Example:
Javascript
let i = 1;
do {
console.log(i);
i++;
} while (i >= 5);
|
3. Arrays
Arrays is a non-primitive datatype in javascript which used to store multiple values. There are two ways to create an array. The easiest way is to create it using an array literal [], and another way is to create using a new keyword.
- The code to create using an array literal: const arr1 = [“javascript”, “java”];
- The code to create using new keyword: const arr2 = new Array(“geeksforgeeks”, “coding”);
4. Function
The function is a block of code that mainly helps to avoid repeating the same code again and again. It makes code more readable and increases its efficiency.
Syntax of regular function:
let a = function function_name(parameters)
{
// block of code
}
Example:
Javascript
function add(x, y)
{
console.log( "The sum is: " ,(x + y));
}
add(1,2);
|
In javascript, there is another type of function which is called the Arrow function. It is one of the useful features of the ES6 version. This function is more readable than a regular function.
Syntax of arrow function:
let a = (parameters) =>
{
// block of code
};
Example:
Javascript
let add = (a, b) => {
let ans = a + b;
return ans;
}
let result = add(5,7);
console.log( "sum is: " ,result);
|
5. Event Listeners
This is an inbuild function in javascript that to used to attach an event handler to an element. Events can be generated in two ways, one is by the user and another is by API. This method is a procedure that waits for the event’s occurrence. When an event occurs, a web page responds according to the event.
Syntax:
addEventListener(event, function, useCapture)
Example:
const element = document.querySelector(".btn")
element.addEventListener("click", () => {
console.log("Button clicked.");
})
According to the above lines of code, If you click the particular button which has a .btn class, then the block of code inside the arrow function will be executed. Do read JavaScript addEventListener() with Examples
6. Error Handling
Here, the main code is in the try block. If there is any error in the try block, then the catch block is executed. If there is no error in the try block, then the code of the try block is executed but the code of the catch block is skipped. But the finally block is executed always if there is any error or not in the try block.
Syntax:
try {
// code of try block
}
catch(error) {
// code of catch block
}
finally() {
// code of finally block
}
7. setTimeOut() and setInterval():
This method executes a block of code only for one time. It is executed after a particular time which is represented in milliseconds.
Syntax:
setTimeout(function, milliseconds);
If you want to cancel this method before it happens then you have to use the clearTimeout() method.
Syntax of clearTimeout () method
clearInterval(intervalID);
Here intervalID is the return value of the setTimeout() method.
Also Read: JavaScript Errors Throw and Try to Catch
8. Objects
This is a nonprimitive datatype. Javascript object part is different than other programming languages. Here, For creating an object, you do not need to create any class.
Syntax of object:
const car = {
Name: 'BMW',
speed: 200
};
9. Class
Class is one of the most important features introduced first in the ES6 version in javascript. Class is a blueprint of its object. You can create many objects from one class.
Syntax of car:
// creating car class
class Car {
constructor(name) {
this.name = name;
}
}
// creating two objects of car class
const car1 = new Person(‘BMW’);
const car2 = new Person(‘Tesla’);
10. JSON
JSON stands for Javascript Object Notation. This is basically a data format that is text-based. This is a data collection that is made of key-value pairs and these pairs are separated by a comma(,). This is language-independent but the syntax of JSON is derived from Javascript Object Notation Syntax.
Syntax:
// Syntax of JSON
{
"course": "Javascript",
"Articles": 15,
"Duration": "two-month",
}
Javascript is supportable in most popular web browsers and also in various operating systems such as Windows, macOS, etc. This provides good control to the users while using browsers. So, you can learn javascript because most of the websites of today’s world are used javascript a lot.
Share your thoughts in the comments
Please Login to comment...