React.js, an open-source and free library, is a powerful tool for building front-end interfaces for websites. It’s not a framework or language, but a library that allows developers to create user interfaces (UI) that are component-based. The use of JavaScript in React is fundamental, and having a solid understanding of JavaScript concepts can make learning and using React.js much easier and faster.
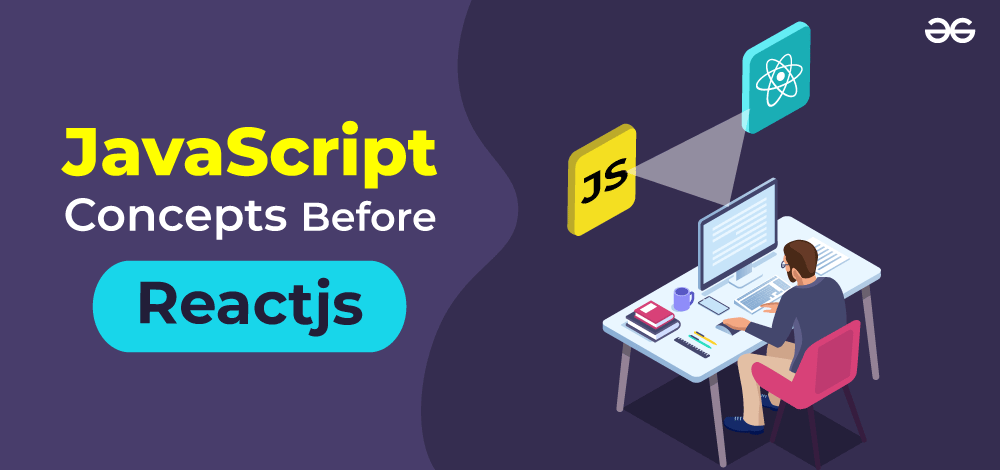
Now, we will see some JavaScript concepts that everyone should know before learning React.js.
JavaScript Concepts You Should Know Before Learning React
Since React.js is a library of JavaScript, it is very essential to know a clear understanding of JavaScript fundamentals and Syntax. Having a good knowledge of the JavaScript foundation will help you learn React.js easily and faster. Here are some must-know top JavaScript Concepts before learning React.js
1. Variables in JavaScript
Variables are used to store data that can be accessed and used in code. But before learning this you should know every basic concept like – the data types, block scope, if-else statement, and some unique features of JavaScript like ‘==’ and ‘===’ operators. Now coming to JavaScript variables, they are dynamic means they can change their value and data type during runtime.
To assign variables, we generally use these keywords:
- Var – Once a declared using the ‘var’ keyword, it can be updated and reassigned in the scope many times.
- let – Once a variable is created using the ‘let’ keyword, it can be updated but cannot be redeclared.
- const – Once a variable is created with the ‘const’ keyword, it can neither be updated, nor redeclared.
Example:
Javascript
// Var Example
var age = 25;
console.log(age);
// Variable can be redeclared and updated
var age = 30;
console.log(age);
// Let Example
let name = "gfg";
console.log(name);
// Variable can be reassigned
name = "GeeksforGeeks";
console.log(name);
Output25
30
gfg
GeeksforGeeks
Example:
Javascript
// Const Example
const pi = 3.14159;
console.log(pi);
// Error: Assignment to a constant variable
pi = 3.14;
Output
3.14159
Error: Assignment to a constant variable.
2. Functions and Arrow Functions in JavaScript
Functions are used to apply the DRY(Do Not Repeat Yourself) principle. To work efficiently with React.js it is very important for you to learn Functions and arrow functions in JavaScript because React Hooks are only implemented by functions and they can only be in functional components. Since as the functionality of an application grows the codebase will grow simultaneously, so with the use of function and arrow function it is easy to encapsulate logic and maintain the readability and reusability of code. Also, it will help in Event Handling.
A function declaration is a named function written with the function keyword. They are hoisted in JavaScript. They have a return statement to specify the function output. An arrow function is an unnamed function, and an easy alternative to function expressions. It does not have a ‘this‘ keyword, neither does it have a function keyword.
Example:
Javascript
// Function Declaration
function multiply(a,b){
return (a*b); //returning the multiplication of two numbers
}
console.log(multiply(3,5));
// Arrow Function
let addition = (a,b)=>{
return (a+b);
}
console.log(addition(3,5));
3. JavaScript Objects
Having a good understanding of objects is very crucial because React components are nothing but JavaScript objects, so to get a better understanding of the structure of React components, objects should be known.
Methods are used as event handlers in React components and having knowledge of objects allows you to handle events and perform an action because objects have methods, which are functions attached to them.
Example:
Javascript
let gfg ={
fullName: "GeeksforGeeks",
type: "edTech",
location: "Noida"
}
console.log(gfg)
Output{ fullName: 'GeeksforGeeks', type: 'edTech', location: 'Noida' }
4. JavaScript Destructuring
Destructuring is one of the important concepts of JavaScript which helps us to extract the needed data from any array or object. But before diving deep into it you should have knowledge of Array and its methods in JavaScript, to know about it, refer to the article – Array and its methods.
Example of Array Destructuring:
Javascript
const vehicles = ['Swift', 'Blackhawk', 'Fortuner'];
const [car, truck, suv] = vehicles;
console.log("Car is", car);
console.log("Truck is", truck);
OutputCar is Swift
Truck is Blackhawk
Object destructuring is similar to array destructuring. Suppose you want to destructure an object named “gfg”, it can be done like this
Example of Object Destructuring:
Javascript
let gfg ={
fullName: "GeeksforGeeks",
type: "eduTech",
location: "Noida"
}
let {fullName: CompanyName, location: cLocation}=gfg;
console.log("company Name is " + CompanyName + " which is located in "+cLocation);
Outputcompany Name is GeeksforGeeks which is located in Noida
It reduces the index reference and increases the code reusability. In React.js, when components receive props as objects, and we need specific data then destructuring makes it easy to do that.
Using destructuring will not only make your code more expressive and readable but add multiple functionalities such as concise syntax and variable renaming. It will improve your ability to work with React components and handle data extraction efficiently.
5. Array Methods in JavaScript
Array object is used to store multiple items, under a single variable name. It is similar to other programming languages. In JavaScript, there are some built-in functions that we can apply to the array, known as array methods. It is very useful to learn before React because it can increase your efficiency and performance. Some of them are:
map – it creates a new array from calling a function iteratively on every array element.
Syntax:
array.map(function(currentValue, index, arr), thisValue)
Example:
Javascript
const array = [1, 2, 3, 4];
function func(num) {
return num + 1;
}
//create a new array with value of each element is increased to 1
const newArr = array.map(func);
console.log("Old array is: ", array);
console.log("New array is: ", newArr);
OutputOld array is: [ 1, 2, 3, 4 ]
New array is: [ 2, 3, 4, 5 ]
filter – this array method creates a new array contained with the elements that are filtered based on the function condition while iterating on the array.
array.filter(function(currentValue, index, arr), thisValue)
Example:
Javascript
const salaries = [10500, 33000, 1600, 4000];
function checkSalary(salary) {
return salary >= 10000;
}
const resultArray = salaries.filter(checkSalary);
console.log("Salaries greater than 10000 are: ", resultArray);
OutputSalaries greater than 10000 are: [ 10500, 33000 ]
reduce – this array method returns a single resultant value by applying a function on every element of the array.
Syntax:
array.reduce( function(total, currentValue, currentIndex, arr), initialValue )
Example:
Javascript
let arr = [10, 20, 30, 40, 50, 60];
function sumofArray(sum, num) {
return sum + num;
}
function myGeeks(item) {
console.log(arr.reduce(sumofArray));
}
myGeeks()
6. Rest and Spread Operator in JavaScript
Both the Rest and Spread Operators are represented by three dots(…). But their use case is completely opposite to each other. The rest operator puts together individual elements into an array while the spread operator expands the array into individual elements.
Example of Spread Operator:
Javascript
const aboutWork = ["help", "students"];
const aboutUs = ["GeeksforGeeks", ...aboutWork, "grow."];
console.log(aboutUs);
Output[ 'GeeksforGeeks', 'help', 'students', 'grow.' ]
Example of Rest operator:
Javascript
function rest(...theArgs) { // it combines all the input in an array
console.log(theArgs.length);
}
rest(); // 0
rest(5); // 1
rest(5, 6, 7); // 3
7. Callback Functions in JavaScript
A callback is a function passed as an argument while calling another function. JavaScript allows us this amazing functionality to handle asynchronous operations and event handling. React components use callback functions to perform an action whenever any event occurs. The callback will help you understand advanced topics like Promises and async and await, which are used for handling asynchronous tasks
Example:
Javascript
// Define the callback function
function callbackFunction() {
console.log("This is Callback function");
}
// Use setTimeout to delay the execution of the callback function
setTimeout(callbackFunction, 5000); // Delay execution for 2 seconds (2000 milliseconds)
console.log("Hi, Geeks");
OutputHi, Geeks
This is Callback function
8. Promises in JavaScript
Because of the callback hell and Inversion of control(IOC) problem, we use Promises to handle asynchronous tasks in JavaScript and make our application scalable. It is used in error handling and overall increases the reliability of our code. It used methods like then(), catch(), and finally() for chaining a series of actions. It allows parallel execution of asynchronous operations while leading to high performance and making our application efficient. It is the foundation of advanced asynchronous features like ‘async’ and ‘await’.
Example:
Javascript
let myPromise = new Promise(function(myResolve, myReject) {
// a new promise named myPromise is created
let x = "GeeksforGeeks";
if (x == "GeeksforGeeks") {
myResolve("Yes it is GeeksforGeeks");
} else { //if gives any error
myReject("Error");
}
});
myPromise.then(
function(value) {console.log("Your Code is working fine");},
function(error) {console.log("Your Code is giving error");;}
);
OutputYour Code is working fine
9. Closure in JavaScript
Closure is the feature of JavaScript that allows an inner function to have access to its current scope as well as its lexical parent scope. It is a very fundamental, basic yet important concept of JavaScript. The closure has the property of encapsulation, code organization, and memorization of its lexical parent scope. In react.js privacy can be achieved using closures, by creating encapsulated logic inside components.
Example:
Javascript
function start() {
var content = "GeeksforGeeks"; // content is a local variable created by start()
function company() {
// company() is the inner function, that forms the closure
console.log(content); // using variable declared in the parent function
}
company();
}
start();
10. Async/Await in JavaScript
JavaScript has asynchronous functionalities to handle operations that will take time to complete such as network requests and file operations. After callback and promises, async and await were introduced, and with this asynchronous code became more readable and simple to handle.
The async keyword is used to declare an asynchronous function that will return a promise. Inside which we will use await keyword which will asynchronously wait for the complete resolution and rejection of the returned promise.
Example:
Javascript
async function getData() {
let promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ name: "GeeksforGeeks"});
}, 200);
});
let Data = await promise;
console.log(Data);
}
getData();
Output{ name: 'GeeksforGeeks' }
11. OOPS(Class and Functional) in JavaScript
OOPs, can be implemented in 2 ways in JavaScript by using functions and classes. Yes, before the introduction of class-based OOps, function-based OOPs were implemented in JS.
class-based OOP: JavaScript introduced class syntax in ECMAScript 2015(ES6) to provide a more familiar OOP approach. In this paradigm, you define classes using the ‘class‘ keyword.
Example:
Javascript
class Rectangle {
constructor(width, height) {
this.width = width;
this.height = height;
}
area() {
return this.width * this.height;
}
perimeter() {
return 2 * (this.width + this.height);
}
}
const rect = new Rectangle(5, 10);
console.log(rect.area()); // Output: 50
console.log(rect.perimeter()); // Output: 30
In this example, the Rectangle class has properties (width and height) and methods (area() and perimeter()). Use the ‘new’ keyword to create an instance and call their methods.
functional-based OOP: it is a prototype inheritance. Before the introduction of classes in JavaScript, it is the only way of implementing OOP. In this paradigm, objects are created by defining functions and attaching methods to their prototypes.
Example:
Javascript
function Rectangle(width, height) {
this.width = width;
this.height = height;
}
Rectangle.prototype.area = function() {
return this.width * this.height;
}
Rectangle.prototype.perimeter = function() {
return 2 * (this.width + this.height);
}
const rect = new Rectangle(5, 10);
console.log(rect.area()); // Output: 50
console.log(rect.perimeter()); // Output: 30
Here, the constructor is a Rectangle function and methods are attached to its prototype. To create instances new keyword is used and methods of these instances can be called.
12. Modules in JavaScript
While coding for a Web Application, it is very important to maintain your code and to make sure that the codes are organized, and JavaScript modules are being used. It breaks the code into separate files, which makes it organized and reusable as well. It is very important to know how JavaScript modules work before learning React.js because it helps in managing dependencies and importing external libraries including React.js.
To learn JavaScript modules properly, you should have knowledge of import and export in JavaScript. Exports can be used in multiple ways, here is one of the syntaxes where we export the members of the module, in the end.
let variable="It is a Variable";
class GfG{
constructor(name){
this.gfg= "Welcome to " +name;
}
}
export {variable, GfG};
Similarly, you can import any useful module into your current file using the ‘import’ keyword. There are multiple ways to import members in a file, one of them is to import a complete file:
import * as syntax from 'syntax.js'
// use 'syntax.variable' to feth the value of variable;
// use 'syntax.GfG' to use GfG class;
Useful Resources
Conclusion
React is a library of JavaScript and is used by many large organizations around the world due to its ease to use and high productivity. React can be used to make a fast User interface very easily, but for this, you should have a sound knowledge of JavaScript. In this article, we have discussed the top JavaScript concepts you should know before starting React.js.
Because having knowledge of JavaScript will make the learning process of React very smooth and easy. Basic fundamentals should be clear and the working of JavaScript like call stack and global execution context will make your interest in learning this verse of JavaScript. Some core topics like destructuring and asynchronous handling of data using promises, Async, and Await will help you a lot in React. These are some of the most known topics and further you can learn multiple concepts along the way.
FAQs on Top JavaScript Concepts For React Beginners
What are the Top Important JavaScript Concepts to know before learning Reactjs
- Variables in JavaScript
- Functions and Arrow Functions
- Objects in JavaScript
- Destructuring
- Array Methods
- Rest and Spread Operators
- Callback Functions
- Promises
- Async / Await in JavaScript
- OOPs in JavaScript
Is React easier than JavaScript?
Learning JavaScript is a prerequisite for React, it becomes easier when we know all the concepts of JavaScript.
Can I learn ReactJS in 10 days?
It depends upon the knowledge of JavaScript, Having a good fundamental knowledge in Javascript takes less time to learn React.
Share your thoughts in the comments
Please Login to comment...