Implementing Undo & Redo Functionality in React Apps
Last Updated :
14 Mar, 2024
Undo/Redo is a feature used frequently in various applications. React Hooks is a concept to handle and respond to the change of state in React Components.
In this article, we will try to implement the Undo/Redo functionality using react hooks thereby increasing your understanding and knowledge of various hooks.
Understanding the Undo & Redo Functionality:
Undo helps in removing the changes that the user has done by mistake or the changes that are no longer required. Redo is reverting the undo action. React Hooks such as useState() and useRef() will be used in this article to implement this functionality. useState() Hook is used to initialize and set the state variables and helps in data binding and useRef() hook responds to the changes in states and helps to track changes without re-rendering the component.
Approach to Implement Undo & Redo Functionality:
- The
useState
hook is used to manage the state of the input value and an array to store the history of input changes. index
is a useRef
hook to keep track of the current index in the input history array.undoFunction
decrements the index to move back in the input history array and updates the input value accordingly.redoFunction
increments the index to move forward in the input history array and updates the input value accordingly.handleInputChange
function updates the input value, increments the index, and updates the input history array with the new value.- The input element’s value and onChange event handler are set to manage the input value and call
handleInputChange
function respectively. - Two buttons, “UNDO” and “REDO“, are provided to trigger the undo and redo functionality, respectively. They are disabled when the index is at the start or end of the input history array.
Steps to Implement Undo & Redo functionality in React App:
Step 1: Create a React App.
npx create-react-app my-react-app
Step 2: Switch to the project directory.
cd my-react-app
Project Strucutre:
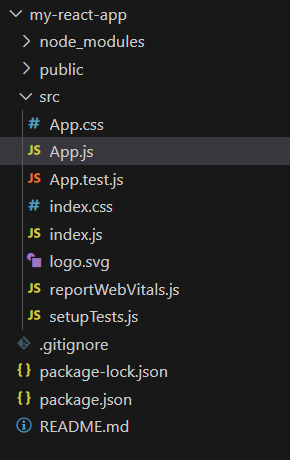
Project Structure
Example: Below is an example of implementing Undo/Redo Functionality with React Hooks.
Javascript
import React, {
useState,
useRef
} from 'react';
function App() {
const [input, setInput] = useState("");
const [inputArray, setInputArray] = useState([input]);
const index = useRef(0);
const undoFunction = () => {
if (index.current > 0) {
index.current = index.current - 1;
setInput(inputArray[index.current]);
}
};
const redoFunction = () => {
if (index.current < inputArray.length - 1) {
index.current = index.current + 1;
setInput(inputArray[index.current]);
}
};
const handleInputChange = (e) => {
const newValue = e.target.value;
setInput(newValue);
index.current = index.current + 1;
setInputArray(
[...inputArray.slice(0, index.current), newValue]
);
};
return (
<div>
<h1>Value: {input}</h1>
<button onClick={undoFunction}
disabled={index.current === 0}>
UNDO
</button>
<button onClick={redoFunction}
disabled={index.current === inputArray.length - 1}>
REDO
</button>
<input type="text"
value={input} onChange={handleInputChange} />
</div>
);
}
export default App;
Output:
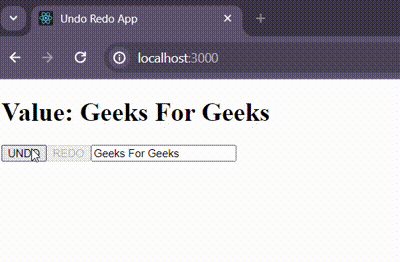
OUTPUT FOR UNDO REDO APP GIVEN IN ABOVE CODE SNIPPET
Share your thoughts in the comments
Please Login to comment...