How to Reset a File Input in React.js ?
Last Updated :
06 Dec, 2023
To reset a file input in React JS, we can use the useRef Hook to create a reference to the input element and modify its properties using the DOM API.
Prerequisites:
Approach:
To reset a file input in react we will create a reset button and handleReset function. The function will access the input element using useRef Hook and reset the input value to an empty string.
The useRef is a React Hook that stores mutable values persistently without triggering a re-render. It can hold references to DOM nodes, counters, or timers.
Syntax:Â
inputFile.current.value = "";
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app file-input
Step 2: After creating your project folder, i.e. file-input, use the following command to navigate to it:
cd file-input
Project Structure:
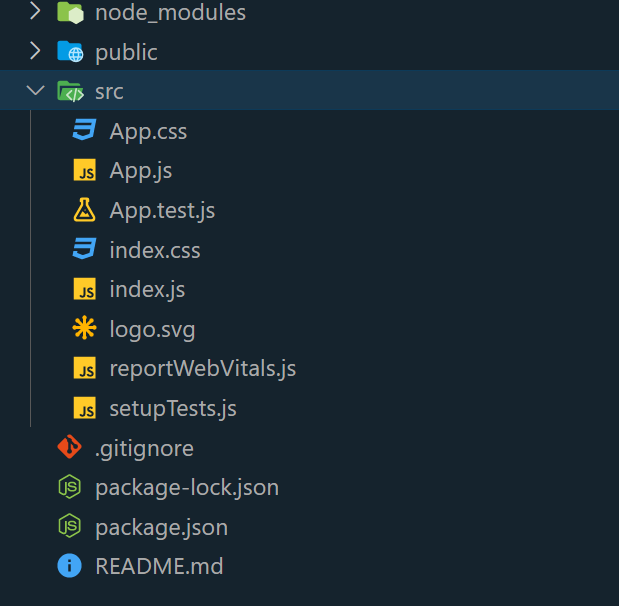
Example: This example uses the useRef hook to create reference and reset the corresponding file input.
Javascript
import React, { useRef } from "react" ;
export default function App() {
const container = {
margin: "5rem" ,
display: "flex" ,
flexDirection: "column" ,
alignItems: "center" ,
};
const input = {
width: "400px" ,
padding: "8px" ,
border: "1px solid #ccc" ,
borderRadius: "4px" ,
fontSize: "14px" ,
boxShadow: "0px 0px 10px 0px grey" ,
};
const button = {
marginTop: "1rem" ,
padding: "10px 10px 10px 10px" ,
border: "none" ,
borderRadius: "8px" ,
backgroundColor: "green" ,
color: "#fff" ,
fontSize: "1rem" ,
cursor: "pointer" ,
transition: "all linear 0.5s" ,
boxShadow: " 0px 0px 10px 0px grey" ,
};
const inputFile = useRef( null );
const handleReset = () => {
if (inputFile.current) {
inputFile.current.value = "" ;
inputFile.current.type = "text" ;
inputFile.current.type = "file" ;
}
};
return (
<div style={container}>
<input style={input}
type= "file" ref={inputFile} />
<button style={button}
onClick={handleReset}>
Reset Input
</button>
</div>
);
}
|
Step to Run the Application: Open the Terminal and enter the command listed below.
npm start
Output: The output will be visible on the http://localhost:3000/ on the browser window.
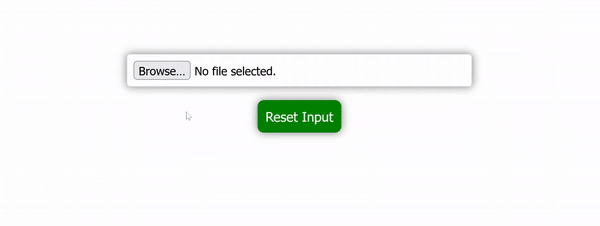
Share your thoughts in the comments
Please Login to comment...