Difference between React.memo() and useMemo() in React.
Last Updated :
29 Feb, 2024
In React applications, optimizing performance is crucial for delivering a smooth user experience. Two key tools for achieving this are React.memo() and useMemo(). While both help improve performance, they serve different purposes and are used in distinct scenarios.
What is React.memo() ?
React.memo() is a higher-order component (HOC) provided by React that memoizes functional components. It means that it caches the result of the component’s rendering based on its props, and re-renders only when the props have changed.
Syntax of React.memo():
const MemoizedComponent = React.memo(FunctionalComponent);
Features of React.memo():
- Automatic Prop Comparison: React.memo() automatically compares the props of the component to determine whether it needs to re-render.
- Memoization of Functional Components: It memoizes the functional component, preventing re-renders if the props remain unchanged.
- Enhanced Performance: By avoiding unnecessary re-renders, React.memo() contributes to improved performance in React applications.
- Easy Implementation: Implementing React.memo() is straightforward, requiring only a single higher-order component.
Example of React.memo()
Example: Below is the code example for the React.memo().
Javascript
import React from 'react' ;
const MyComponent = React.memo((props) => {
console.log( 'Rendering MyComponent' );
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>{props.message}</p>
</div>
);
});
function App() {
const [count, setCount] = React.useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<button onClick={handleClick}>
Update Count
</button>
<MyComponent name= "React"
message= "Welcome to React world!" />
</div>
);
}
export default App;
|
Step to run the app:
npm start
Output:
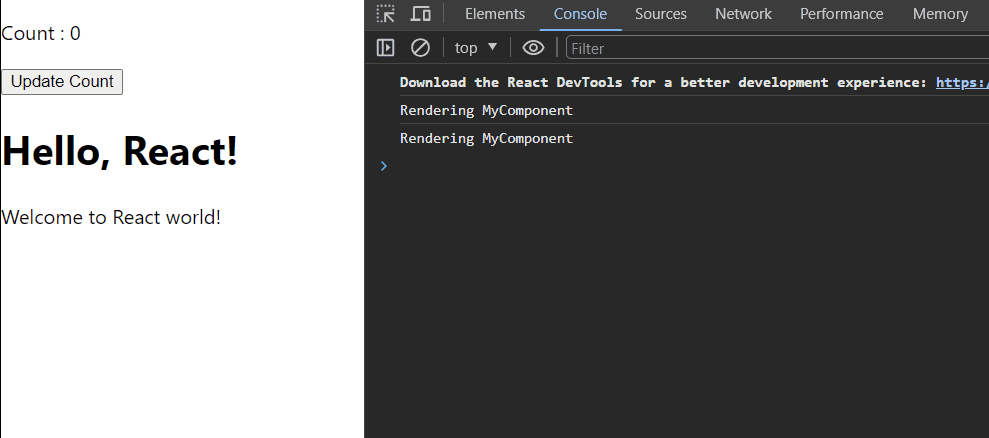
What is useMemo() ?
useMemo() is a React Hook used to memoize the result of expensive computations within functional components. It memorizes the value returned by a provided function and re-calculates it only when the dependencies change.
Syntax of useMemo():
const memoizedValue = useMemo(() => computeExpensiveValue(dep1, dep2), [dep1, dep2]);
Features of useMemo():
- Custom Dependency Management: useMemo() allows specifying dependencies, ensuring that the memoized value is recalculated only when these dependencies change.
- Memoization of Expensive Computations: It’s suitable for memoizing values derived from computationally intensive operations.
- Performance Optimization: By caching the result of expensive computations, useMemo() optimizes the performance of functional components.
- Flexible Usage: useMemo() can be used with any value that needs to be memoized, not just React components.
Example of useMemo()
Example: Below is the code example for the use.memo().
Javascript
import React,
{
useState,
useMemo
} from 'react' ;
const WithMemo = () => {
const [count, setCount] = useState(0);
const [renderCount, setRenderCount] = useState(0);
const computeExpensiveValue = (num) => {
console.log( "Computing..." );
let result = 0;
for (let i = 0; i < 1000000000; i++) {
result += num;
}
return result;
};
const result = useMemo(
() =>
computeExpensiveValue(count), [count]
);
return (
<div>
<h2>With Memo Example</h2>
<p>Count: {count}</p>
<p>Result: {result}</p>
<p>Render Count: {renderCount}</p>
<button
onClick={
() => setCount(count + 1)
}>
Increment Count
</button>
<button onClick={
() =>
setRenderCount(renderCount + 1)
}>
Increment Render Count
</button>
</div>
);
};
export default WithMemo;
|
Step to run the app:
npm start
Output:
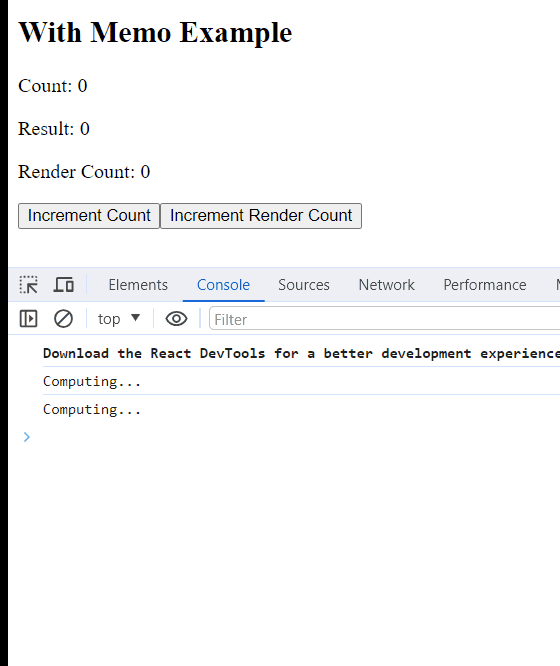
Difference Between React.memo() and useMemo():
Feature
|
React.memo()
|
useMemo()
|
Purpose
|
Memoizes functional components
|
Memoizes the result of expensive computations
|
Dependency
|
Props
|
Custom dependencies array
|
Syntax
|
HOC wrapping
|
Hook invocation
|
Return Value
|
Memoized component
|
Memoized value
|
Typical Use Case
|
Memoizing functional components based on props
|
Memoizing expensive computations
|
Conclusion:
React.memo() and useMemo() are essential tools for optimizing React applications. While React.memo() focuses on memoizing functional components to prevent unnecessary re-renders based on props, useMemo() is used to memoize expensive computations within functional components. Understanding their differences and appropriate usage is key to effectively improving performance in React applications.
Share your thoughts in the comments
Please Login to comment...