Optimizing Performance of List Rendering with useMemo Hook in React
Last Updated :
05 Apr, 2024
List rendering is a common task in web development, especially in React applications where components often display dynamic lists of data. However, rendering large lists can lead to performance issues if not handled efficiently. In this article, we’ll explore how to optimize the performance of list rendering in React using the useMemo
hook.
Understanding the Need for Optimization
When rendering a list of items in React, each item typically corresponds to a component. If the list is extensive and components are re-rendered unnecessarily, it can impact performance and user experience. The useMemo
hook helps mitigate this issue by memoizing values based on dependencies, preventing unnecessary re-renders.
What is useMemo
Hook
The useMemo
hook in React is used to memoize computationally expensive values and calculations. It takes a function and an array of dependencies and returns a memoized value. When the dependencies change, useMemo
re-execute the function to update the memoized value.
Benefits of usememo hook
- Boosts Performance: useMemo speeds up your app by avoiding unnecessary computations during re-renders.
- Reduces Re-renders: It helps prevent re-renders for components with costly operations, unless their dependencies change.
- Saves Resources: By avoiding repetitive processing, useMemo conserves system resources for efficient data handling.
- Improves User Experience: This hook leads to smoother interactions and faster response times, enhancing user satisfaction.
- Increases Control: useMemo offers precise control over when specific computations should run, optimizing component behavior.
Leveraging useMemo to Optimize List Rendering
To address this issue, we can utilize the useMemo hook provided by React. useMemo allows us to memoize the result of expensive computations, ensuring that they are only recalculated when the dependencies change. By memoizing the list data, we can prevent unnecessary recalculations and improve the overall rendering performance of our application.
Approach
- State Initialization: The component ItemList starts by defining its state using useState. It keeps track of two pieces of state: searchTerm for storing the user’s search input and count for a numeric value that can be incremented.
- List Definition: A static list named itemList is defined inside the component. This list is an array of objects, where each object represents an item with an id and name.
- Memoizing Filtered List: To optimize performance, useMemo is employed to memoize the filtered version of itemList based on the search term. The memoization ensures that the filtering operation (which could be computationally expensive) is only executed when searchTerm changes, thus avoiding unnecessary recalculations on every render.
- Dynamic Search Implementation: The component renders an input field that updates searchTerm on change. This interaction triggers the memoized filtering of itemList, displaying only the items that match the search term.
- Increment Counter: Besides the search functionality, there’s a button that increments a counter (count). This part demonstrates that updating count with the setCount function does not trigger the memoized filtering operation, showcasing the efficiency of useMemo in avoiding unnecessary recalculations of the filtered list when unrelated state changes.
Example: This example showcase the optimization performance of list rendering.
Javascript
import React, { useState, useMemo } from 'react';
const ItemList = () => {
// Define the list of items
const itemList = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
// Add more items as needed
];
// Use state to track changes in the component
const [searchTerm, setSearchTerm] = useState('');
const [count, setCount] = useState(0);
// Filter the list of items based on the search term
const filteredItems = useMemo(() => {
console.log('filtered items rendered');
return itemList.filter(item =>
item.name.toLowerCase().includes(searchTerm.toLowerCase())
);
}, [searchTerm]);
return (
<div>
<h1>Item List</h1>
<input
type="text"
placeholder="Search"
value={searchTerm}
onChange={e => setSearchTerm(e.target.value)}
/>
<ul>
{filteredItems.map(item => (
<li key={item.id}>
{item.name}
</li>
))}
</ul>
<h1>{count}</h1>
<button onClick={
() => setCount(count + 1)}>Inc.
</button>
</div>
);
};
export default ItemList;
Output:
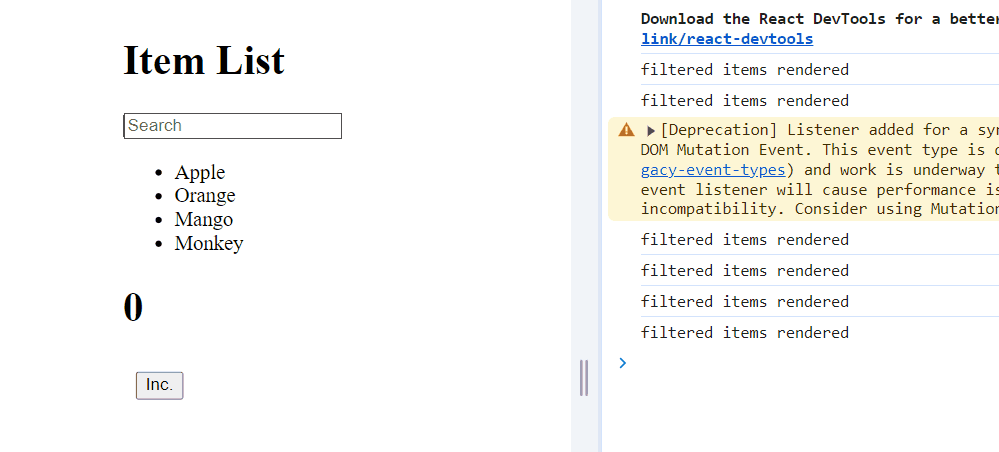
Conclusion:
By utilizing the useMemo hook, we can optimize the performance of list rendering in React applications. Memoizing expensive computations ensures that they are only recalculated when necessary, leading to improved rendering performance and a smoother user experience. With the simple example provided in this article, you can easily implement useMemo to enhance the performance of list rendering in your React components.
Share your thoughts in the comments
Please Login to comment...