How useMemo Hook optimizes performance in React Component ?
Last Updated :
18 Mar, 2024
The useMemo hook in React optimizes performance by memoizing the result of a function and caching it. This caching ensures that expensive computations inside the useMemo callback are only re-executed when the dependencies change, preventing unnecessary recalculations and improving the rendering performance of the component.
By memoizing the result of a function computation, ‘useMemo‘ helps prevent unnecessary re-execution of expensive computations in a React component. This optimization avoids redundant calculations during renders, improving performance, especially with complex computations and large components.
Approach to implement useMemo Hook in React Component:
- In the below example define
calculateFactorial
function to compute factorial and use useMemo
to memoize based on number
dependency. - Ensure the factorial is recalculated only on
number
prop change, optimizing performance. - Add a counter button to increase
count
state, showing an unrelated state doesn’t trigger a recalculation. - Demonstrate
useMemo
maintaining memoized value during re-renders, showcasing efficiency. - Highlight performance benefits in scenarios with costly computations, improving overall app performance.
Example: The below example calculates the factorial of a number.
Javascript
import React, { useState, useMemo } from 'react';
const FactorialCalculator = () => {
const [number, setNumber] = useState(0)
const [count, setCount] = useState(0);
const calculateFactorial = (num) => {
console.log(`Calculating factorial of ${num}`);
let result = 1;
for (let i = 2; i <= num; i++) {
result *= i;
}
return result;
};
const factorial = useMemo(
() => calculateFactorial(number), [number]);
return (
<div>
<h2>Factorial Calculator</h2>
<p>Number:{number} </p>
<p>Factorial: {factorial}</p>
<button onClick={
() => setNumber(number + 1)}>Next factorial
</button>
<hr />
<p>Count: {count}</p>
<button onClick={
() => setCount(count + 1)}>Increment Count
</button>
</div>
);
};
export default FactorialCalculator;
Output:
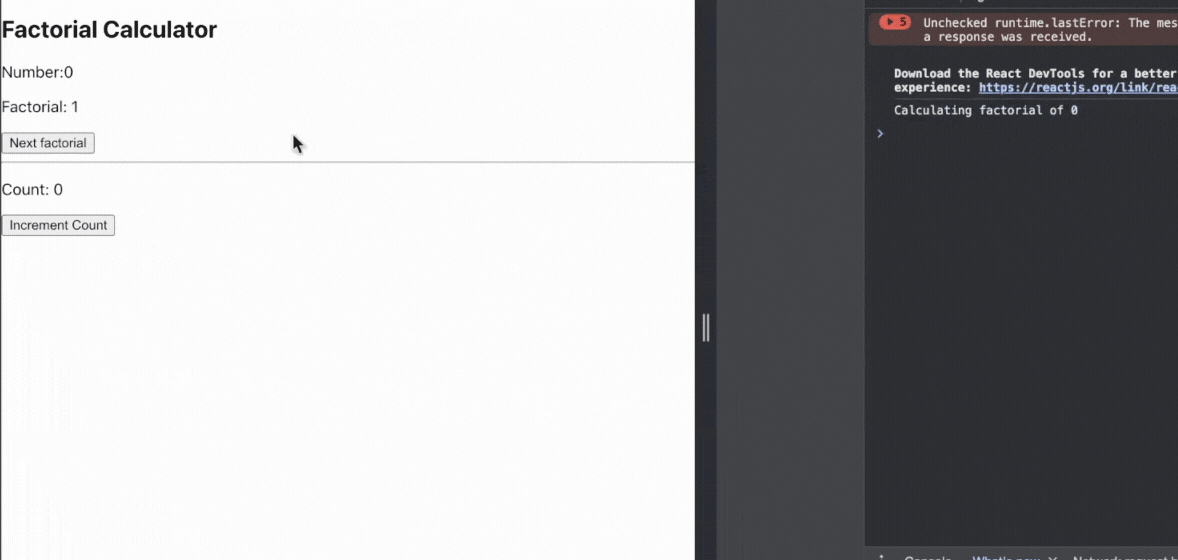
Conclusion:
The ‘useMemo’ hook in React is a powerful tool for optimizing performance by memoizing the result of a function computation. By using `useMemo`, you can prevent unnecessary re-renders of components and improve the overall efficiency of your React applications. Incorporating `useMemo` into your components can lead to smoother user experiences and better performance, especially in scenarios where heavy computations are involved.
Share your thoughts in the comments
Please Login to comment...