How to use jQuery in Angular ?
Last Updated :
20 Dec, 2023
In this tutorial, we will learn how we can use jQuery with Angular. There are two ways in which we can use jQuery with Angular as discussed below:
By installing jQuery using the npm command
You can install the jQuery in your current project folder using the below commands
npm install --save jquery
Now add the below HTML code to your app.component.html file.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Jquery in Angular</ title >
</ head >
< body >
< h1 style = "color:green" >GeeksforGeeks</ h1 >
< h2 >Jquery in Angular</ h2 >
< button >click me </ button >
< p id = "result" ></ p >
</ body >
</ html >
|
After adding the HTML code add the below Angular code to app.component.ts file to use jQuery in Angular.
Javascript
import { Component, OnInit } from '@angular/core' ;
import $ from 'jquery' ;
@Component({
selector: 'my-app' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
ngOnInit()
{
$( 'button' ).click( function () {
$( '#result' ).html( '<b>jQuery used in angular by installation.</b>' );
});
}
}
|
Output:
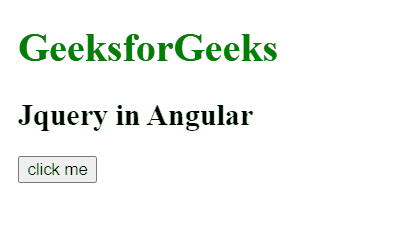
Using jQuery CDN to use it
You can use the jQuery in Angular by simply adding the jQuery CDN in head tag of the index.html file.
CDN Link:
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"
integrity=
"sha512-v2CJ7UaYy4JwqLDIrZUI/4hqeoQieOmAZNXBeQyjo21dadnwR+8ZaIJVT8EE2iyI61OV8e6M8PP2/4hpQINQ/g=="
crossorigin="anonymous" referrerpolicy="no-referrer">
</script>
Add the below HTML code to you index.html file.
html
<!DOCTYPE html>
< html >
< head >
< title >Jquery in Angular</ title >
</ head >
< body >
< h1 style = "color:green" >GeeksforGeeks</ h1 >
< h2 >Jquery in Angular</ h2 >
< button >click me</ button >
< p id = "result" ></ p >
< script src =
integrity =
"sha512-v2CJ7UaYy4JwqLDIrZUI/4hqeoQieOmAZNXBeQyjo21dadnwR+8ZaIJVT8EE2iyI61OV8e6M8PP2/4hpQINQ/g=="
crossorigin = "anonymous"
referrerpolicy = "no-referrer"
></ script >
< my-app >loading</ my-app >
</ body >
</ html >
|
Now, addbelow code to your app.component.ts file to see the use of jQuery.
html
import { Component, OnInit } from '@angular/core';
// import $ from 'jquery';
declare var $: any;
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements OnInit {
ngOnInit() {
$('button').click(function () {
$('#result').html('< b >jQuery used in angular by installation.</ b >');
});
}
}
|
Output:
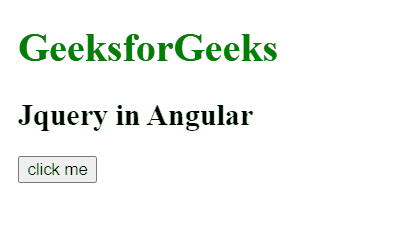
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”. You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...