What is the difference between anchor href vs angular routerlink in Angular ?
Last Updated :
08 Jan, 2024
Routing in Angular allows the users to create a single-page application with multiple views and allows navigation between them. Users can switch between these views without losing the application state and properties. In this example, we will learn the difference between anchor href vs angular routerlink in Angular.
href Attribute
The href attribute is a standard HTML attribute used in anchor (<a>) tags to specify the URL of the resource being linked to. When used in Angular without the Angular Router, it triggers a full page reload when navigating to a different route, resulting in a complete page refresh.
Syntax
<a href="/home">
Go to Home
</a>
Example: This example illustrates the usage of the href Attribute, where in this case, clicking on the links triggers a full page reload as the href attribute is used.
HTML
< head >
< link href =
rel = "stylesheet"
integrity =
"sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin = "anonymous" >
</ head >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >
What is the difference between anchor href
vs angular routerlink in Angular?
</ h3 >
< a href = "/home" > Home</ a >< br />
< a href = "/about" >About</ a >
< router-outlet ></ router-outlet >
|
HTML
< h3 style = "color:violet" >
Hi Geek!! Use the like button to vote
</ h3 >
|
HTML
< h3 style = "color: indianred;" >
Hi Geek!! Please vote for me for
Geeks Premier League
</ h3 >
|
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { AppComponent }
from './app.component' ;
import { AboutComponent }
from './about/about.component' ;
import { HomeComponent }
from './home/home.component' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { RouterModule, Routes }
from '@angular/router' ;
const routes: Routes = [
{ path: 'home' , component: HomeComponent },
{ path: 'about' , component: AboutComponent },
{ path: '' , redirectTo: '/home' , pathMatch: 'full' }
];
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent,
HomeComponent,
AboutComponent
],
imports: [
RouterModule.forRoot(routes),
BrowserModule,
AppRoutingModule
],
exports: [
RouterModule
]
})
export class AppModule { }
|
Output:
.gif)
routerLink Directive
The routerLink directive is part of the Angular Router and is used to navigate between routes within an Angular application. It provides a more dynamic and efficient way of handling navigation compared to traditional href links, as it updates the view without triggering a full page reload. The routerLink can also take an array to navigate with parameters or additional options.
Syntax
<a routerLink="/home">
Go to Home
</a>
Example: This example illustrates the usage of the routerLink Directive, where the navigation occurs within the Angular application without a full page reload.
HTML
< head >
< link href =
rel = "stylesheet"
integrity =
"sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin = "anonymous" >
</ head >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
What is the difference between anchor
href vs angular routerlink in Angular?
</ h3 >
< a routerLink = "/home" > Home</ a >< br />
< a routerLink = "/about" >About</ a >
< router-outlet ></ router-outlet >
|
HTML
< h3 style = "color:violet" >
Hi Geek!! Use the like button to vote
</ h3 >
|
HTML
< h3 style = "color: indianred;" >
Hi Geek!! Please vote for me for
Geeks Premier League
</ h3 >
|
Javascript
import { Component } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser'
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent { }
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { AppComponent }
from './app.component' ;
import { AboutComponent }
from './about/about.component' ;
import { HomeComponent }
from './home/home.component' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { RouterModule, Routes }
from '@angular/router' ;
const routes: Routes = [
{ path: 'home' , component: HomeComponent },
{ path: 'about' , component: AboutComponent },
{ path: '' , redirectTo: '/home' , pathMatch: 'full' }
];
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent,
HomeComponent,
AboutComponent
],
imports: [
RouterModule.forRoot(routes),
BrowserModule,
AppRoutingModule
],
exports: [
RouterModule
]
})
export class AppModule { }
|
Output:
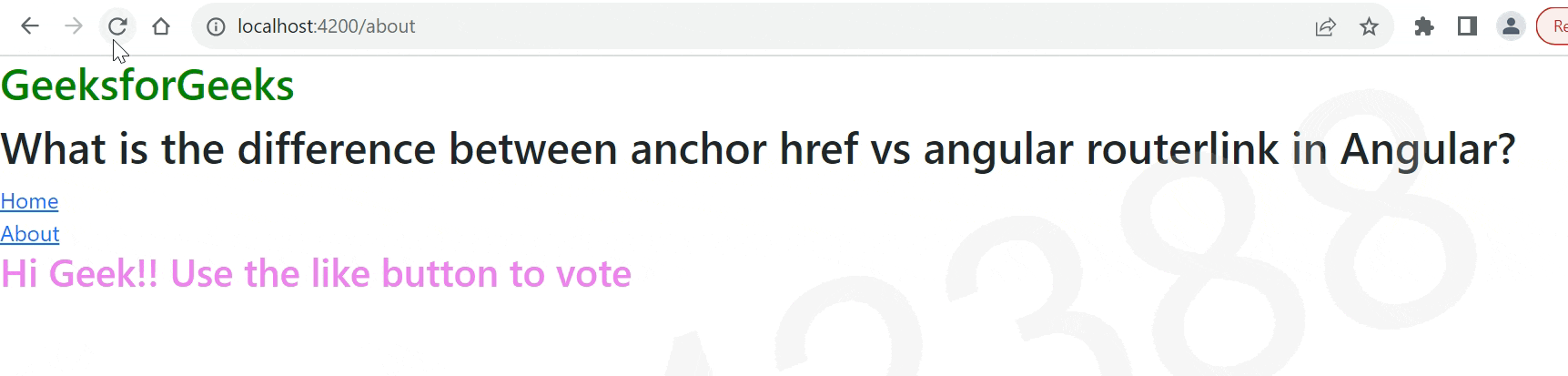
Difference between href and routerLink
Features |
href
|
routerLink
|
Navigation Mechanism |
It triggers a full page reload and performs a traditional navigation, fetching a completely new HTML page from the server. |
It uses Angular’s built-in router to navigate between views within a single-page application, updating the content dynamically without a full page reload. |
Page Reload |
Results in a complete page refresh, causing the loss of the current application state and potentially leading to a slower user experience. |
Navigates without reloading the entire page, maintaining the application state and providing a smoother user experience. |
Single Page Application (SPA) |
Originally designed for traditional multi-page applications, where each link corresponds to a separate HTML page. |
Tailored for SPAs, enabling seamless navigation between views without the need for full page reloads. |
Angular Router Features |
Lacks integration with Angular Router features, such as route parameters, route guards, and other advanced navigation options. |
Works seamlessly with Angular Router, allowing for the utilization of features like route parameters, query parameters, child routes, and route guards. |
Application Performance |
May introduce delays due to full page reloads and increased server requests. |
Enhances performance by loading only the necessary components and updating the view within the existing application, reducing latency. |
Dynamic Navigation |
Generally used for static links to external resources or other pages without involving dynamic Angular features. |
Facilitates dynamic navigation within the Angular application, supporting dynamic route generation based on components and data. |
Conclusion
The href is a standard HTML attribute for linking to external resources and causes a full page reload in Angular applications, whereas, the routerLink is a directive provided by the Angular Router specifically designed for navigating within the application without triggering a complete page refresh. Using routerLink is generally preferred in Angular applications for a smoother and more efficient user experience.
The href is a traditional HTML attribute for general-purpose navigation, while the routerLink is a specialized directive provided by Angular Router for efficient, dynamic, and state-preserving navigation within single-page applications. The choice between them depends on the specific requirements of your application and whether you are working within the context of a single-page application.
Share your thoughts in the comments
Please Login to comment...