How to use Handlebars to Render HTML Templates ?
Last Updated :
10 Apr, 2024
Handlebars is a powerful template engine that allows us to create dynamic HTML templates. It helps to render HTML templates easily. One of the advantages of Handlebars is its simplicity, flexibility, and compatibility with various web development frameworks.
These are the following methods:
Using npm package
Steps to use Handlebars to render HTML templates
Step 1: Create a new server using the following command.
npm init -y
Step 2: Install the necessary package in your application using the following command.
npm install express
npm install hbs
Step 3 : For using a Handlebars in the Express.js code you must create a folder name “views” and in there create a file which must be end with “.hbs”. Otherwise you got error.
Example: abc.hbs
Folder Structure:
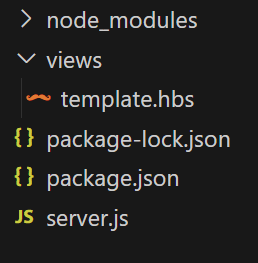
Folder structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.3",
"hbs": "^4.2.0"
}
}
Example: Write the following code in the files created as shown in folder structure
HTML
{{!-- template.hbs code... --}}
<html>
<head>
<title>{{ title }}</title>
<style>
body {
font-family: 'Segoe UI', Tahoma,
Geneva, Verdana, sans-serif;
background-color: #f8f9fa;
margin: 0;
padding: 0;
height: 100vh;
color: #444;
line-height: 1.6;
}
.container {
display: flex;
justify-content: space-between;
max-width: 900px;
padding: 20px;
width: 55vw;
}
.card {
flex: 0 0 calc(33.33% - 20px);
background-color: #58e724;
margin-bottom: 20px;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease;
margin-left: 9rem;
}
.card:hover {
transform: translateY(-5px);
}
h2 {
color: #fff;
margin-bottom: 10px;
text-align: center;
}
p {
color: #666;
text-align: center;
}
.author {
font-style: italic;
color: #888;
}
.button {
display: block;
width: 100%;
margin-top: 20px;
padding: 5px;
text-align: center;
color: #fff;
background-color: #007bff;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
text-decoration: none;
}
.button:hover {
background-color: #0056b3;
}
.main_heading {
text-align: center;
margin-top: 5rem;
margin-bottom: 20px;
color: rgb(27, 230, 91);
}
</style>
</head>
<body>
<h1 class="main_heading">
This is a Example to use Handlebars
to render HTML templates
</h1>
<div class="container">
{{#each books}}
<div class="card">
<h2>{{ this.title }}</h2>
<p class="author">
Written by: {{ this.author }}
</p>
<div class="body">
{{{ this.synopsis }}}
</div>
<a href="#" class="button">
Read More...
</a>
</div>
{{/each}}
</div>
</body>
</html>
JavaScript
// server.js code....
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.set('view engine', 'hbs');
const data = {
title: 'Handlebars Example',
books: [
{
title: 'Book 1',
author: 'John Doe',
synopsis: 'This is the synopsis of book 1.'
},
{
title: 'Book 2',
author: 'Jane Smith',
synopsis: 'This is the synopsis of book 2.'
},
{
title: 'Book 3',
author: 'Bob Johnson',
synopsis: 'This is the synopsis of book 3.'
}
]
};
// Define a route to render a template
app.get('/', (req, res) => {
res.render('template', data);
});
app.listen(PORT, (err) => {
if (err) {
console.log(err);
} else {
console.log(`Server is running on port ${PORT}`);
}
});
To run the code wite in the command prompt
node server.js
And open a new tab of your browser and type the following command to show the output
http://localhost:3000/
Output:
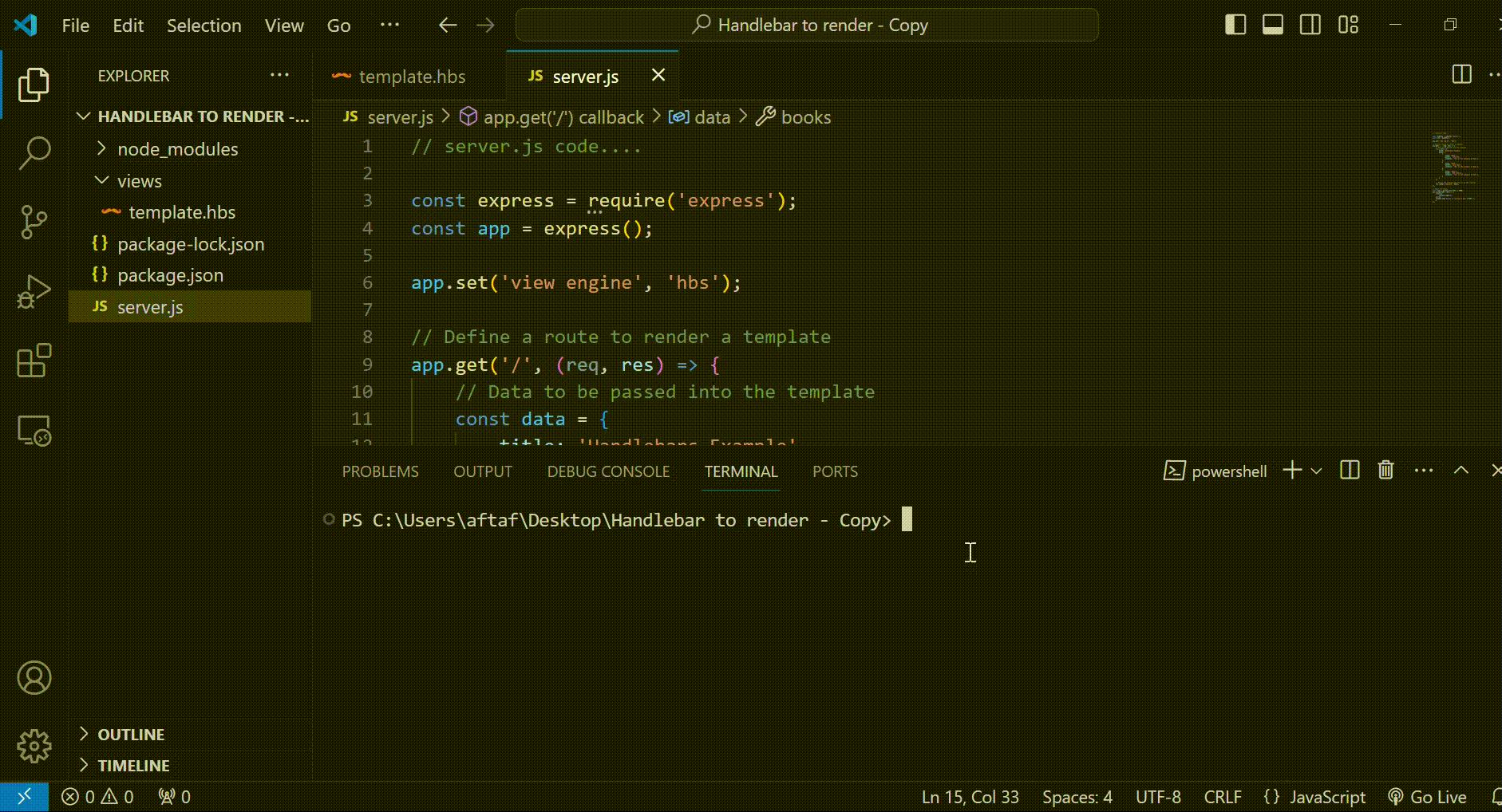
Output
Using CDN Link
- First create a Html template and include the Handlebars CDN link in the <head> section using <script> tag.
- Then create a JavaScript section at the bottom of the HTML file or in an external JavaScript file and in the javascript code define the data object containing information about the books. .
- For compile the Handlebars template and retrieving its content using document.getElementById(‘book-template’).innerHTML and then used Handlebars.compile() method.
Example: The below code uses the handlebars CDN Link to use use handlebars.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Handlebars Example</title>
<script src=
"https://cdn.jsdelivr.net/npm/handlebars@4.7.7/dist/handlebars.min.js">
</script>
<style>
body {
font-family:
'Segoe UI', Tahoma, Geneva,
Verdana, sans-serif;
background-color: #f8f9fa;
margin: 0;
padding: 0;
height: 100vh;
color: #444;
line-height: 1.6;
}
.container {
display: flex;
justify-content: space-between;
max-width: 900px;
padding: 20px;
width: 55vw;
}
.card {
flex: 0 0 calc(33.33% - 20px);
background-color: #58e724;
margin-bottom: 20px;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease;
margin-left: 9rem;
}
.card:hover {
transform: translateY(-5px);
}
h2 {
color: #fff;
margin-bottom: 10px;
text-align: center;
}
p {
color: #666;
text-align: center;
}
.author {
font-style: italic;
color: #888;
}
.button {
display: block;
width: 100%;
margin-top: 20px;
padding: 5px;
text-align: center;
color: #fff;
background-color: #007bff;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
text-decoration: none;
}
.button:hover {
background-color: #0056b3;
}
.main_heading {
text-align: center;
margin-top: 5rem;
margin-bottom: 20px;
color: rgb(27, 230, 91);
}
</style>
</head>
<body>
<h1 class="main_heading">
This is an Example to use Handlebars to
render HTML templates
</h1>
<div class="container"></div>
<script id="book-template"
type="text/x-handlebars-template">
{{#each books}}
<div class="card">
<h2>{{ this.title }}</h2>
<p class="author">Written by: {{ this.author }}</p>
<div class="body">{{{ this.synopsis }}}</div>
<a href="#" class="button">Read More...</a>
</div>
{{/each}}
</script>
<script>
const data = {
title: 'Handlebars Example',
books: [
{
title: 'Book 1',
author: 'John Doe',
synopsis:
'This is the synopsis of book 1.'
},
{
title: 'Book 2',
author: 'Jane Smith',
synopsis:
'This is the synopsis of book 2.'
},
{
title: 'Book 3',
author: 'Bob Johnson',
synopsis:
'This is the synopsis of book 3.'
}
]
};
const source = document.
getElementById('book-template').innerHTML;
const template = Handlebars.compile(source);
const html = template(data);
document.querySelector('.container').
innerHTML = html;
</script>
</body>
</html>
Output:
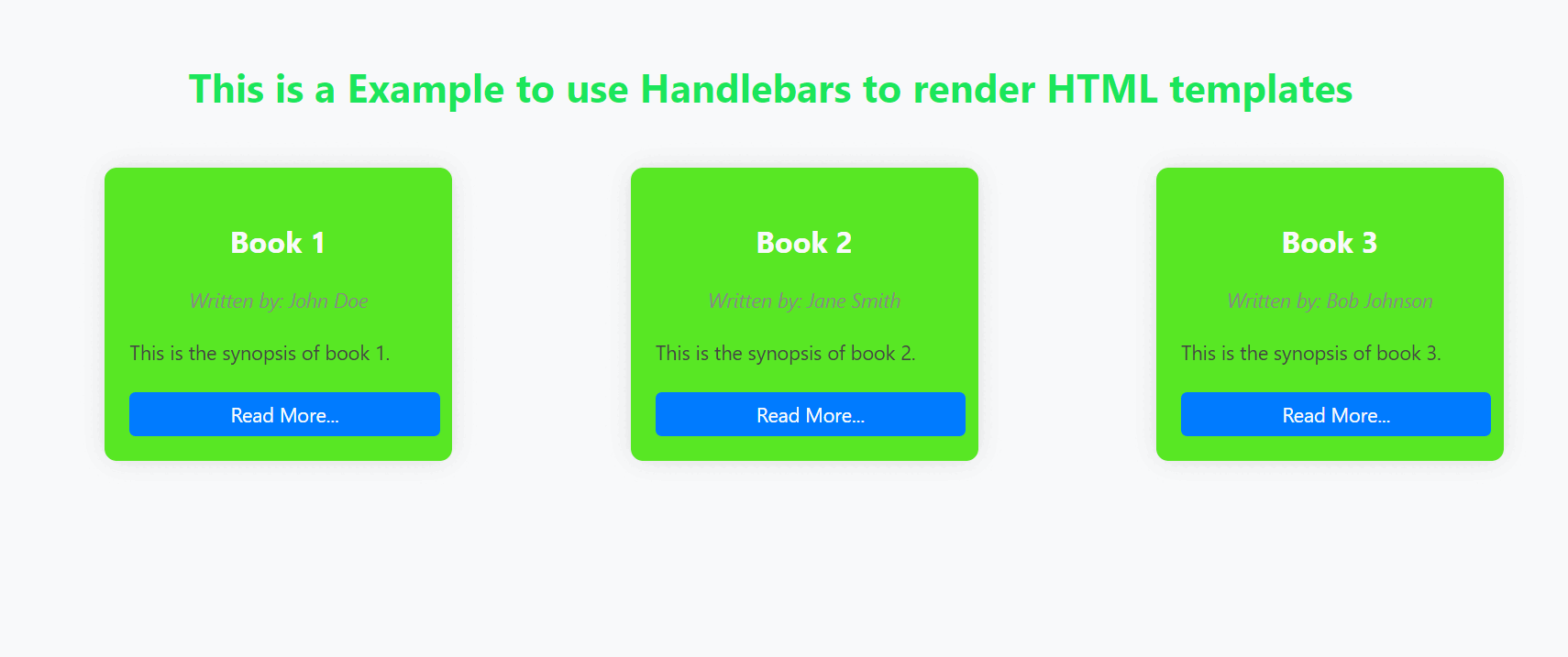
Output
Share your thoughts in the comments
Please Login to comment...