How to setup React Router v6 ?
Last Updated :
29 Feb, 2024
React Router is an amazing library that was created by Remix. The purpose of React Router is to enable Client-Side routing in the React application, that is, by using React Router we can create different routes in our React app and can easily navigate to different pages without even reloading the page.
Client-side routing, facilitated by React Router, enables changing routes without page reloads, creating single-page applications (SPAs). React Router efficiently manages what is displayed on each route, making it a powerful and user-friendly library for seamless navigation in React applications.
We will discuss the following setup approach and different methods of React Router v6 in this article:
Approach to setup React Router in Application:
- NavBar.js: Utilizes the
Link
tag instead of an anchor tag for navigation without page reloads. Ensures the website remains a single-page application.
- index.js: Uses
createBrowserRouter
to establish routes: “/” (Home), “/home” (About), “/contact” (Contact). Wraps components needing routing in RouterProvider
with the created router.
- App.js: Renders NavBar and the
Outlet
component provided by React Router. Outlet
dynamically displays content based on the current route.
Steps to set up React Router:
Step 1: Create a react project folder, open the terminal, and write the following command.
# Run this to use npm
npx create-react-app foldername
# Or run this to use yarn
yarn create react-app foldername
Step 2: Navigate to the root directory of your project using the following command.
cd foldername
Step 3: Now, install the React Router library
npm install react-router-dom
or
yarn add react-router-dom
Project Structure:
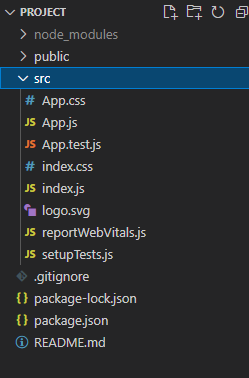
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
"react-router-dom": "^6.22.0"
}
Implementation of React Router v6:
Example: Below is the code to create the navbar and routes.
CSS
nav {
background-color : #333 ;
padding : 10px ;
}
ul {
list-style-type : none ;
margin : 0 ;
padding : 0 ;
}
li {
display : inline ;
margin-right : 20px ;
}
a {
text-decoration : none ;
color : white ;
}
a:hover {
color : lightgray;
}
|
Javascript
import { Link } from 'react-router-dom' ;
const Navbar = () => {
return (
<nav>
<ul>
<li><Link to= "/" >Home</Link></li>
<li><Link to= "/about" >About</Link></li>
<li><Link to= "/contact" >Contact</Link></li>
</ul>
</nav>
);
}
export default Navbar;
|
Javascript
import React from 'react'
import ReactDOM from 'react-dom/client'
import {
RouterProvider,
createBrowserRouter
} from 'react-router-dom'
import App from './App'
import Home from './Home'
import About from './About'
import Contact from './Contact'
const router = createBrowserRouter([
{
path: '/' ,
element: <App />,
children: [
{
path: '/' ,
element: <Home />,
},
{
path: '/about' ,
element: <About />,
},
{
path: '/contact' ,
element: <Contact />,
},
]
}
]);
ReactDOM.createRoot(document.getElementById( 'root' )).render(
<RouterProvider router={router}>
<App />
</RouterProvider>
)
|
Javascript
import { Outlet } from 'react-router-dom' ;
import './App.css'
import NavBar from "./NavBar" ;
const App = () => {
return (
<>
<NavBar />
<Outlet />
</>
);
}
export default App;
|
Javascript
import React from 'react'
const Home = () => {
return (
<div>Home</div>
)
}
export default Home
|
Javascript
import React from 'react'
const About = () => {
return (
<div>About</div>
)
}
export default About
|
Javascript
import React from 'react'
const Contact = () => {
return (
<div>Contact</div>
)
}
export default Contact
|
Start your application using the following command.
npm start
Output:
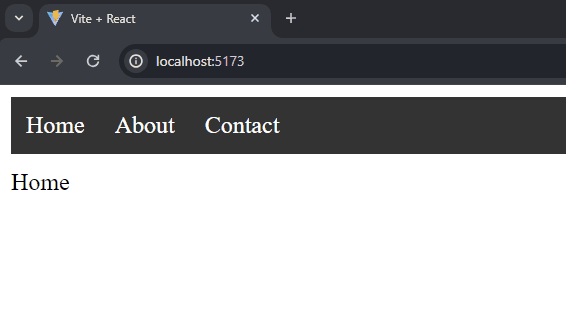
Output
Nested Routes:
Nested routes means routes within routes. For example, the About page can have two links, one link for About company and one link for About founder of the company.
We will have to create routes something like these.
"/about/about-founder"
"/about/about-company"
This is what nested routes are. So creating nested routes is very simple, as you have already created a route for about, you have to add children array to it.
Example 2: Below is an example of nested routes.
Javascript
import { Outlet, useNavigate } from "react-router-dom"
const About = () => {
const navigate = useNavigate();
return (
<div>
<button onClick={() => navigate( "about-founder" )}>About - Founder</button>
<button onClick={() => navigate( "about-company" )}>About - Company</button>
<Outlet />
</div>
)
}
export default About
|
Javascript
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App'
import { RouterProvider, createBrowserRouter } from 'react-router-dom'
import Home from './Home'
import About from './About'
import Contact from './Contact'
import Founder from './Founder'
import Company from './Company'
const router = createBrowserRouter([
{
path: '/' ,
element: <App />,
children: [
{
path: '/' ,
element: <Home />,
},
{
path: '/about' ,
element: <About />,
children: [
{
path: "about-founder" ,
element: <Founder />
},
{
path: "about-company" ,
element: <Company />
}
]
},
{
path: '/contact' ,
element: <Contact />,
},
]
}
]);
ReactDOM.createRoot(document.getElementById( 'root' )).render(
<React.StrictMode>
<RouterProvider router={router}>
<App />
</RouterProvider>
</React.StrictMode>
)
|
Javascript
import React from 'react'
const Founder = () => {
return (
<div>Founder</div>
)
}
export default Founder
|
Javascript
import React from 'react'
const Company = () => {
return (
<div>Comapny</div>
)
}
export default Company
|
Output:
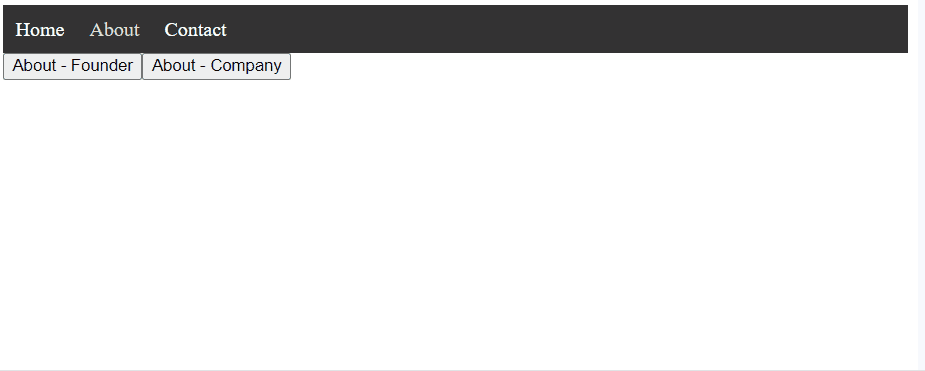
Output
useNavigate():
This is a hook given by React Router. useNavigate returns a function, and using this function we can navigate anywhere. You just need to enter the path where you want to navigate. That’s all there was to nested routes, now you can create routes at any depth. Now let’s see how to create dynamic routes.
DynamicRoutes:
Let us first understand what dynamic routes are. Dynamic route means the route that we did not know before. They are created dynamically based on any variable or parameter and are non-static and numbers can reach thousands and millions.
for example, this youtube video link:
https://youtube.com/watch/[some video id]
Now this video ID will be different for every video. So such routes are dynamic routes.
To set up dynamic routes, let’s take an example. Suppose there are many contacts in the contact page and each contact has a unique ID. We will just use this unique ID and create pages for all contacts with just one route.
We can write a path like this
path: "/contact/:id"
Example 3: Look at the below code for a better understanding
Javascript
import { useParams } from "react-router-dom"
const ContactCard = () => {
const { id } = useParams();
return (
<h1>Contact id: {id}</h1>
)
}
export default ContactCard
|
Javascript
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App'
import { RouterProvider, createBrowserRouter } from 'react-router-dom'
import Home from './Home'
import About from './About'
import Contact from './Contact'
import Founder from './Founder'
import Company from './Company'
import ContactCard from './ContactCard'
const router = createBrowserRouter([
{
path: '/' ,
element: <App />,
children: [
{
path: '/' ,
element: <Home />,
},
{
path: '/about' ,
element: <About />,
children: [
{
path: "about-founder" ,
element: <Founder />
},
{
path: "about-company" ,
element: <Company />
}
]
},
{
path: '/contact' ,
element: <Contact />,
},
{
path: "/contact/:id" ,
element: <ContactCard />
}
]
}
]);
ReactDOM.createRoot(document.getElementById( 'root' )).render(
<React.StrictMode>
<RouterProvider router={router}>
<App />
</RouterProvider>
</React.StrictMode>
)
|
Output:
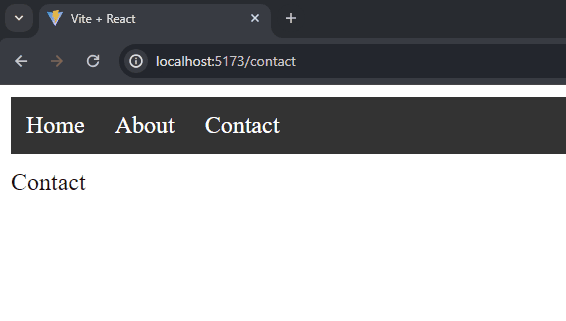
Dynamic Routes: Output
createRoutesFromElements:
If you do not like the syntax to create routes from these objects then we can also use a different syntax. To do this, we will call another function in createBrowserRouter which is createRoutesFromElements and in this function, we will set up the routes.
import {
RouterProvider,
createBrowserRouter,
createRoutesFromElements,
Route,
} from "react-router-dom";
const router = createBrowserRouter(
createRoutesFromElements(
<Route path="/" element={<App />}>
<Route path="/" element={<Home />} />
\\Other Route
</Route>
)
);
Share your thoughts in the comments
Please Login to comment...