How to implement client-side routing in React?
Last Updated :
09 Feb, 2024
Client-side routing is a fundamental concept in modern single-page applications (SPAs) that allows for navigation within the application without a full page reload. In React, libraries like React Router provide the tools necessary to implement client-side routing seamlessly.
In this article, we will explore the process of implementing client-side routing in a React application, covering various aspects and best practices.
Prerequisites
Using React Router
React Router is a standard library for routing in React. It allows you to handle routes declaratively. It enables dynamic routing, which means that your application can modify its routes based on the application’s state or user interactions. It supports everything from basic routing to nested routes, route parameters, and authenticated routes.
Steps to Implement React Router
Step 1: Create a react app by using the following command.
npx create-react-app routing
cd routing
Step 2: Install the required dependencies.
npm install react-router-dom
Project Structure:
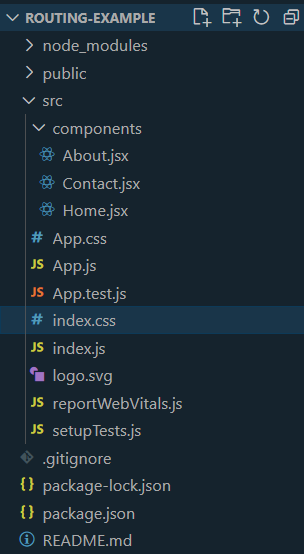
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Create the required files and add the following code.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { BrowserRouter } from 'react-router-dom' ;
import App from './App' ;
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById( 'root' )
);
|
Javascript
import React from 'react' ;
import { Routes, Route, Link } from 'react-router-dom' ;
import Home from './components/Home' ;
import About from './components/About' ;
import Contact from './components/Contact' ;
function App() {
return (
<div>
<nav>
<ul>
<li>
<Link to= "/" >Home</Link>
</li>
<li>
<Link to= "/about" >About Us</Link>
</li>
<li>
<Link to= "/contact" >Contact Us</Link>
</li>
</ul>
</nav>
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
<Route path= "/contact" element={<Contact />} />
</Routes>
</div>
);
}
export default App;
|
Javascript
import React from 'react' ;
function About() {
return (
<div>
<h1>About Us</h1>
<p>Learn more about our company and mission.</p>
</div>
);
}
export default About;
|
Javascript
import React from 'react' ;
function Home() {
return (
<div>
<h1>Welcome to my app!</h1>
<p>This is the home page.</p>
</div>
);
}
export default Home;
|
Javascript
import React from 'react' ;
function Contact() {
return (
<div>
<h1>Contact Us</h1>
<p>Get in touch with us.</p>
</div>
);
}
export default Contact;
|
Output:
.gif)
React Router Pros and Cons:
Pros:
- Organized Code: It keeps your app structure logical and maintainable.
- Declarative Routing: It has simple setup, intuitive path definitions.
- Dynamic URLs: It has powerful navigation based on user interactions or data.
- SEO-friendly: Supports SSR and SSG for better search visibility.
- Large Community & Ecosystem: Plenty of resources and solutions available.
Cons:
- Learning Curve: Requires some effort to understand the concepts and API.
- Boilerplate Code: Initial setup might involve repetitive code.
- Performance Overhead: Adds a slight overhead compared to manual routing.
- Complex for Large Apps: Managing extensive navigation can get intricate.
Share your thoughts in the comments
Please Login to comment...