How to Set Box Shadow in React JS ?
Last Updated :
04 Dec, 2023
The CSS property box-shadow is used to set box shadow in React. This can apply to any React.js component. The CSS box-shadow property creates shadow effects around an element’s frame possible to set several effects at once by separating them with commas. A box shadow is defined by the element’s X and Y offsets, blur and spread radius, and color. In this article, we’ll look at how to create a box shadow.
Prerequisites
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app boxshadow-project
Step 2: After creating your project folder, i.e. boxshadow-project, use the following command to navigate to it:
cd react-project
Project Structure:
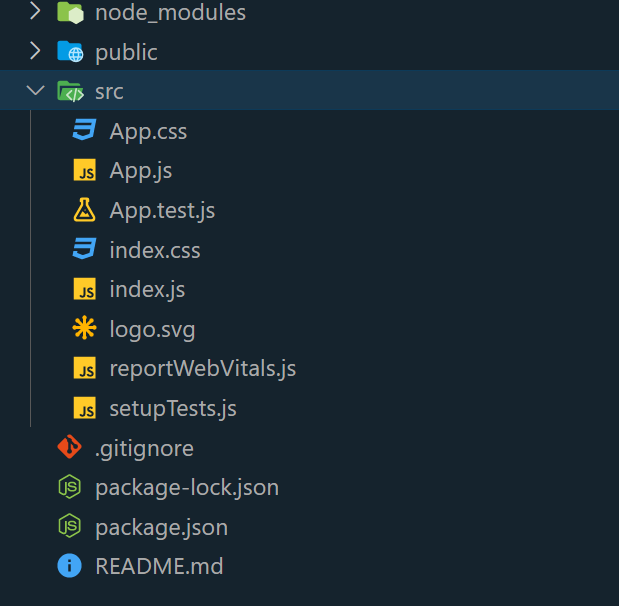
Example 1: Â In this example, the App component uses useState hook to create a showBox state variable, initially set to false. The handleClick function toggles showBox value on button click. Code returns a button invoking the handleClick function on click, and an inline conditional rendering shows a green text header and a paragraph in a div with class “box” if showBox is true.
Javascript
import React, { useState } from "react" ;
import "./App.css" ;
function App() {
const [showBox, setShowBox] = useState( false );
const handleClick = () => {
setShowBox(!showBox);
};
return (
<>
<button className= "button"
onClick={handleClick}>
Box Shadow
</button>
{showBox && (
<div className= "box" >
<h1 style={{ color: "green" }}>
Welcome To Geeksforgeeks!!
</h1>
<p>
<!-- Content goes here -->
</p>
</div>
)}
</>
);
}
export default App;
|
CSS
body {
margin : 1 rem;
}
.button {
padding : 12px 24px ;
background-color : green ;
border-radius: 10px ;
border : none ;
color : #fff ;
font-size : 16px ;
cursor : pointer ;
box-shadow: 1px 1px 10px 1px grey;
}
.box {
border-radius: 10px ;
margin-top : 1 rem;
width : 50% ;
height : 150px ;
padding : 10px 10px 10px 10px ;
background-color : #f5f5f5 ;
border : 1px solid #ccc ;
box-shadow: 1px 1px 10px 0px red ;
}
|
To run the application, open the Terminal and enter the command listed below. Go to http://localhost:3000/ to see the output.
npm start
Output:
Â
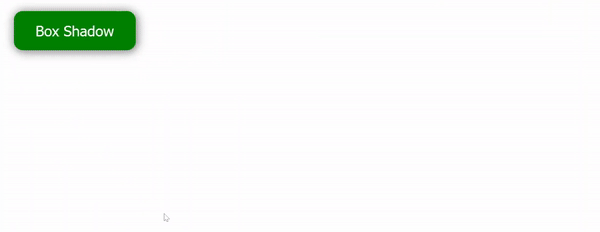
Example 2: This example is the same as the previous example but the difference is that in one previous example, we use onclick function to show the box shadow on a content box but in this example, we use :hover to show the box shadow. When the user hovers over box content it will show a box shadow. Â To run this code follow the same step which is previously mentioned.
Javascript
import React from "react" ;
import "./App.css" ;
function App() {
return (
<>
<div className= "box" >
<h1 style={{ color: "green" }}>
Welcome To Geeksforgeeks!!
</h1>
<p>
A Computer Science portal for
geeks. It contains well written,
well thought and well explained
computer science and programming
articles
</p>
</div>
</>
);
}
export default App;
|
CSS
body {
margin : 2 rem;
}
.box {
border-radius: 10px ;
margin-top : 1 rem;
width : 50% ;
height : 150px ;
padding : 10px 10px 10px 10px ;
background-color : #f5f5f5 ;
border : 1px solid #ccc ;
transition: linear all 0.4 s;
cursor : pointer ;
}
.box:hover {
box-shadow: 1px 1px 10px 0px red ;
}
|
Output:
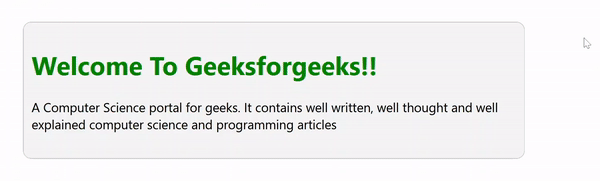
Share your thoughts in the comments
Please Login to comment...