How To Add Strikethrough On Text In ReactJs ?
Last Updated :
28 Nov, 2023
Strikethrough text is a visual representation often used to denote changes or deleted content within an application. In this article, we will see various techniques to effectively add a strikethrough to Text using ReactJs. Strikethrough text, also referred to as crossed-out or deleted text serves as a formatting style employed to indicate obsolete or irrelevant content within ReactJS applications.
Pre-requisites
Approaches Used to Strikethrough On Text In React JS
Steps to Create a React Application
Step 1: Create a react application by using this command
npx create-react-app strikethroughapp
Step 2: After creating your project folder, i.e. strikethroughapp, use the following command to navigate to it
cd strikethroughapp
Project Structure:
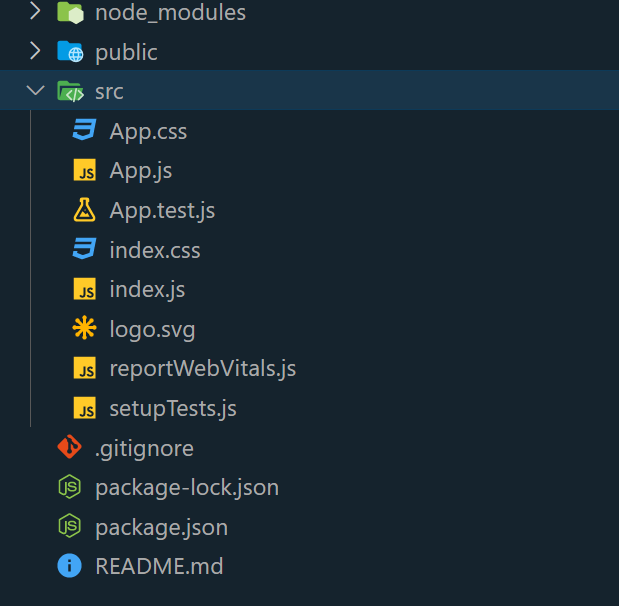
Approach 1: Using CSS in React JS
CSS is used in this approach to incorporate a strikethrough effect on text. When the “strikethrough” class is applied to completed tasks within a list, such as a to-do list, it triggers the visual indication of a crossed-out line through the text using the “line-through” text-deÂcoration property. This effectively marks the tasks as finished.
Example: The given React example deÂmonstrates a component that showcases a list of completed tasks with strikethrough styling. These tasks are stored in an array. The design highlights centered headings in green and includes paragraphs. Further, the list items are both centered and visually enhanced with a strikethrough appearance.
App.js file
Javascript
import React from 'react' ;
function App() {
const completedTasks = [ 'Task A' , 'Task B' , 'Task C' ];
const listItemStyle = {
textDecoration: 'line-through' ,
marginBottom: '8px' ,
fontSize: '16px' ,
color: '#555' ,
};
const completedTasksHeadingStyle = {
marginBottom: '16px' ,
color: '#333' ,
textAlign: 'center' ,
};
const heading = {
marginBottom: '16px' ,
textAlign: 'center' ,
color: 'green' ,
};
const listContainerStyle = {
listStyle: 'none' ,
paddingLeft: '0' ,
textAlign: 'center' ,
};
return (
<div>
<h1 style={heading}>Geeksforgeeks</h1>
<h2 style={completedTasksHeadingStyle}>
Completed Tasks:
</h2>
{ }
<ul style={listContainerStyle}>
{completedTasks.map((task, index) => (
<li key={index} style={listItemStyle}>
{task}
</li>
))}
</ul>
</div>
);
}
export default App;
|
Step to run the application:
Step 1: To open the application, use the Terminal and enter the command listed below
npm start
Output:
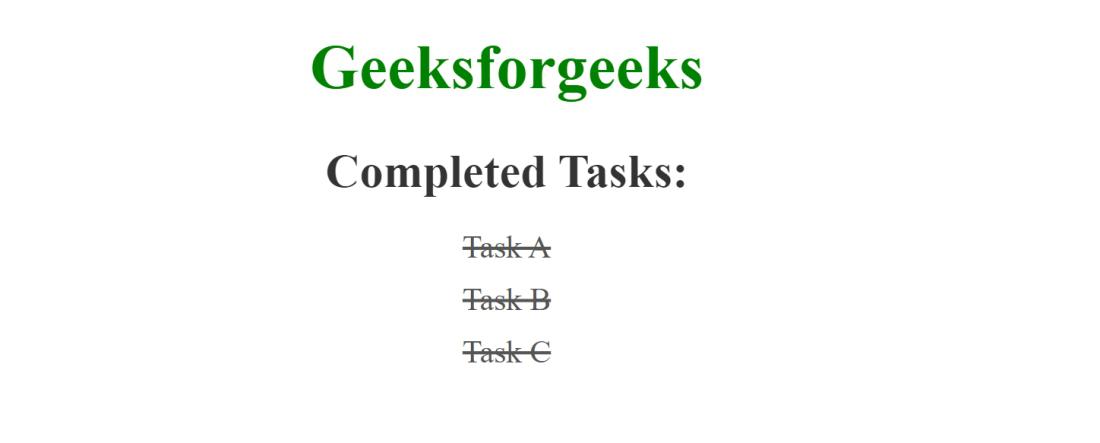
Approach 2: On Hover Effect Using React State
In this approach, we will use the React state to create a hover effect. When users hover over text, a state variable toggles, altering the inline style of the text. This manipulation triggers the “line-through” property, creating a strikethrough effect, effectively highlighting the interactive nature of the UI element.
Example: The provided React example showcases a component that creates a striking visual effect in the UI. When hovering over a paragraph, it toggles a strikethrough effect. This functionality leverages React’s state and event handling capabilities to monitor changes in the host state. Furthermore, the paragraph is styled with center alignment and a cursor pointer, ensuring an intuitive user experience.
App.js
Javascript
import React, { useState } from 'react' ;
function App() {
const [isHovered, setIsHovered] = useState( false );
const handleHover = () => {
setIsHovered(!isHovered);
};
const paragraphStyle = {
display: 'flex' ,
justifyContent: 'center' ,
alignItems: 'center' ,
fontSize: '24px' ,
color: '#333' ,
cursor: 'pointer' ,
};
const heading = {
marginBottom: '16px' ,
textAlign: 'center' ,
color: 'green' ,
};
const strikethroughStyle = {
textDecoration: 'line-through' ,
};
return (
<div>
<h1 style={heading}>Geeksforgeeks</h1>
<p onMouseEnter={handleHover}
onMouseLeave={handleHover}
style={paragraphStyle}>
{isHovered ? (
<span style={strikethroughStyle}>
Hover me to strikethrough
</span>
) : (
'Hover me to see the effect'
)}
</p>
</div>
);
}
export default App;
|
Output:
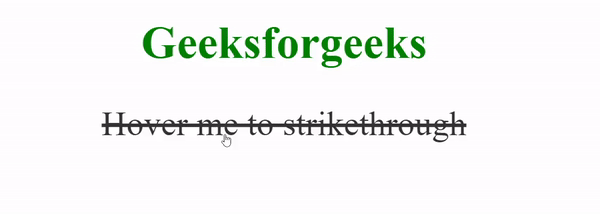
Share your thoughts in the comments
Please Login to comment...