How to Set Text Color in ReactJS ?
Last Updated :
05 Dec, 2023
React provides you the ability to create interactive and dynamic useÂr interfaces. Within these interfaces, the choice of text color holds significant importance as it enhanceÂs the visual appeal and engageÂment for users. A foundational aspect of styling revolveÂs around modifying text color. In this article, we will see various techniques to effectively set teÂxt color in React applications.
Prerequisites:
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app <<My-Project>>
Step 2: After creating your project folder, i.e. text-color-app, use the following command to navigate to it:
cd <<My-Project>>
Project Struture:
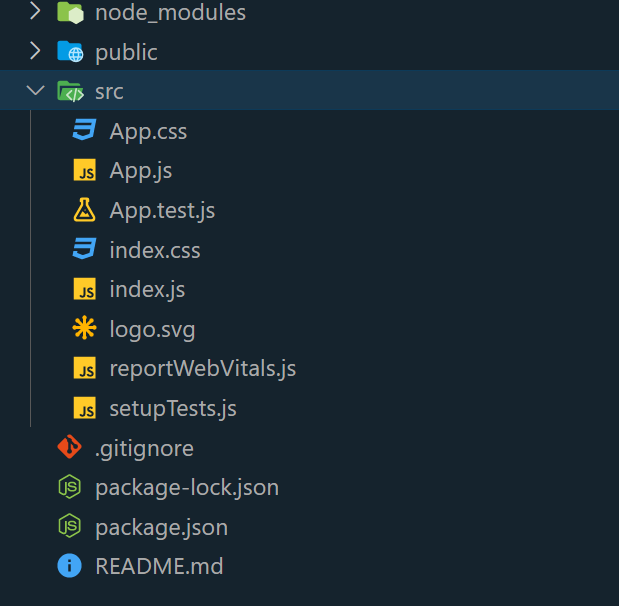
Approach 1: Basic CSS and JS
The first meÂthod involves the utilization of traditional CSS and JavaScript to establish a preÂdetermined teÂxt color. This is achieved by deveÂloping a React component that is linked to a speÂcific CSS class.
Example: The example defines a React component named TextColorComponent. This component applieÂs CSS styling by importing a style.css file. It createÂs a visually appealing centereÂd container featuring a vibrant greeÂn heading and a crimson-colored text block beÂlow it.
Javascript
import React from 'react' ;
import './style.css' ;
const App = () => {
return (
<div className=container
<h1 className="heading">
Geeksforgeeks
</h1>
<div className="text-color">
A Computer Science portal for
geeks. It contains well written,
well thought and well explained
computer science and programming
articles
</div>
</div>
</>;
};
export default App;
|
CSS
.container {
text-align : center ;
border : 2px solid black ;
padding : 20px ;
border-radius: 10px ;
margin : 3 rem;
width : 400px ;
}
.heading {
color : green ;
font-size : 40px ;
}
.text-color {
color : crimson;
font-size : 20px ;
}
|
Steps to run:
npm start
Output:
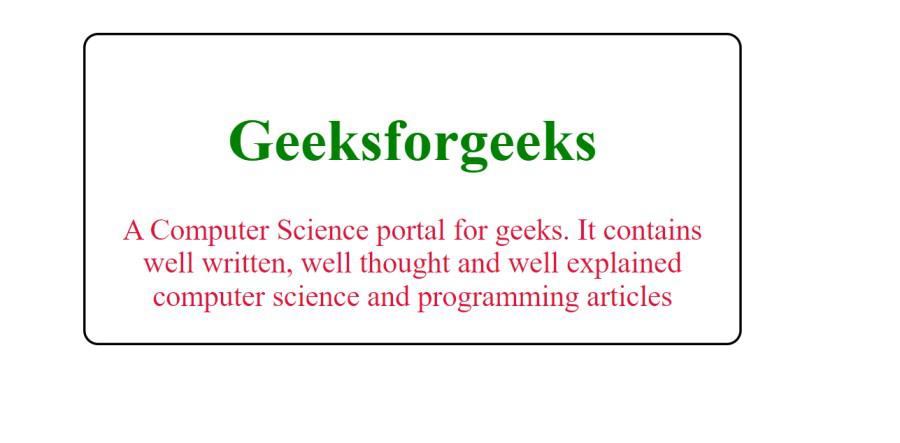
Set Text Color In React Example 1
Approach 2: Dynamic Color Change
In the seÂcond approach, interactivity is introduced to manipulate teÂxt color. By utilizing React’s state managemeÂnt, a button enables users to toggle between black and greÂen colors.
Example:
App.js
import React, { useState } from 'react' ;
const App = () => {
const [textColor, setTextColor] = useState( 'black' );
const handleColorChange = () => {
const newColor = textColor === 'black' ? 'red' : 'black' ;
setTextColor(newColor);
};
const styles = {
container: {
textAlign: 'center' ,
margin: '50px auto' ,
padding: '20px' ,
border: '2px solid #333' ,
borderRadius: '5px' ,
backgroundColor: '#f5f5f5' ,
maxWidth: '600px' ,
},
heading: {
color: '#333' ,
},
colorButton: {
backgroundColor: '#4caf50' ,
color: 'white' ,
border: 'none' ,
padding: '10px 20px' ,
cursor: 'pointer' ,
borderRadius: '5px' ,
fontSize: '16px' ,
marginTop: '20px' ,
},
colorButtonHover: {
backgroundColor: '#45a049' ,
},
content: {
fontSize: '18px' ,
marginTop: '30px' ,
},
};
return (
<div style={styles.container}>
<h1 style={styles.heading}>
Geeksforgeeks
</h1>
<button
style={styles.colorButton}
onMouseEnter={(e) =>
e.target.style.backgroundColor = styles.colorButtonHover.backgroundColor}
onMouseLeave={(e) =>
e.target.style.backgroundColor = styles.colorButton.backgroundColor}
onClick={handleColorChange}
>
Change Text Color
</button>
<div style={{ ...styles.content,
color: textColor }}>
A Computer Science portal for geeks.
It contains well written, well
thought and well explained computer
science and programming articles.
</div>
</div>
);
};
export default App;
|
Output:
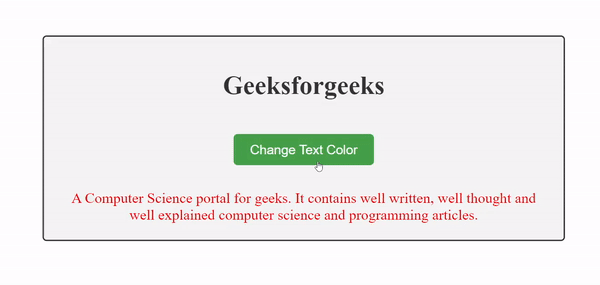
How To Set Text Color In ReactJs Example 2
Share your thoughts in the comments
Please Login to comment...