How to Create Card with Box Shadow in React Native ?
Last Updated :
06 Mar, 2024
Creating Card with box shadow in react native makes it stand out within the interface and display information in an appealing way. It will be done in React Native by using the expo cli.
Expo simplifies cross-platform app development by providing a unified codebase for iOS, Android, and the web. With its tools and services, developers can create high-quality apps effortlessly.
Pre-requisites
Approach to Create Card with Box Shadow in React Native
To Create Card with Box Shadow in React Native we will be using React Native View Component and add the shadow effect with the box-shadow property like shadowOffset and color in CSS object.
Syntax for Cards with Box Shadow in React Native
box-shadow: [h offset] [v offset] [blur radius]
[optional spread] [any color];
Steps to Create React Native Application
Step 1:Â Create a react native application by using this command
npx create-expo-app card-with-boxshadow
Step 2:Â After creating your project folder, i.e. card-with-boxshadow, use the following command to navigate to it:
cd card-with-boxshadow
Card with Box Shadow in React Native Examples
Example 1: Simple Card with Box Shadow in React Native
In this example, we will see create the box-shadow using react native.
Javascript
import React from 'react' ;
import { View, Text, StyleSheet, Dimensions }
from 'react-native' ;
const App = () => {
return (
<View style={styles.container}>
{ }
<View style={styles.card}>
{ }
<View style={styles.header}>
<Text style={styles.title}>
Welcome To Geeksforgeeks!!
</Text>
<Text style={styles.subtitle}>
React Native Card
</Text>
</View>
{ }
<View style={styles.content}>
<Text style={styles.text}>
A Computer Science portal for geeks.
It contains well written, well thought
and well explained computer science
and programming articles
</Text>
</View>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
backgroundColor: '#f5f5f5' ,
},
card: {
backgroundColor: 'white' ,
borderRadius: 15,
padding: 16,
shadowColor: 'black' ,
shadowOffset: {
width: 0,
height: 4,
},
shadowOpacity: 0.3,
shadowRadius: 6,
elevation: 14,
width: 350,
height: 350,
justifyContent: 'center' ,
alignItems: 'center' ,
},
header: {
marginBottom: 16,
alignItems: 'center' ,
},
title: {
fontSize: 30,
fontWeight: 'bold' ,
color: 'green' ,
},
subtitle: {
fontSize: 24,
color: '#333' ,
marginTop: 10,
},
content: {
alignItems: 'center' ,
},
text: {
fontSize: 17,
color: '#444444' ,
textAlign: 'center' ,
},
});
export default App;
|
Step to run the application: open the Terminal and enter the command listed below.Â
npx expo start
npx react-native run-android
npx react-native run-ios
Output:
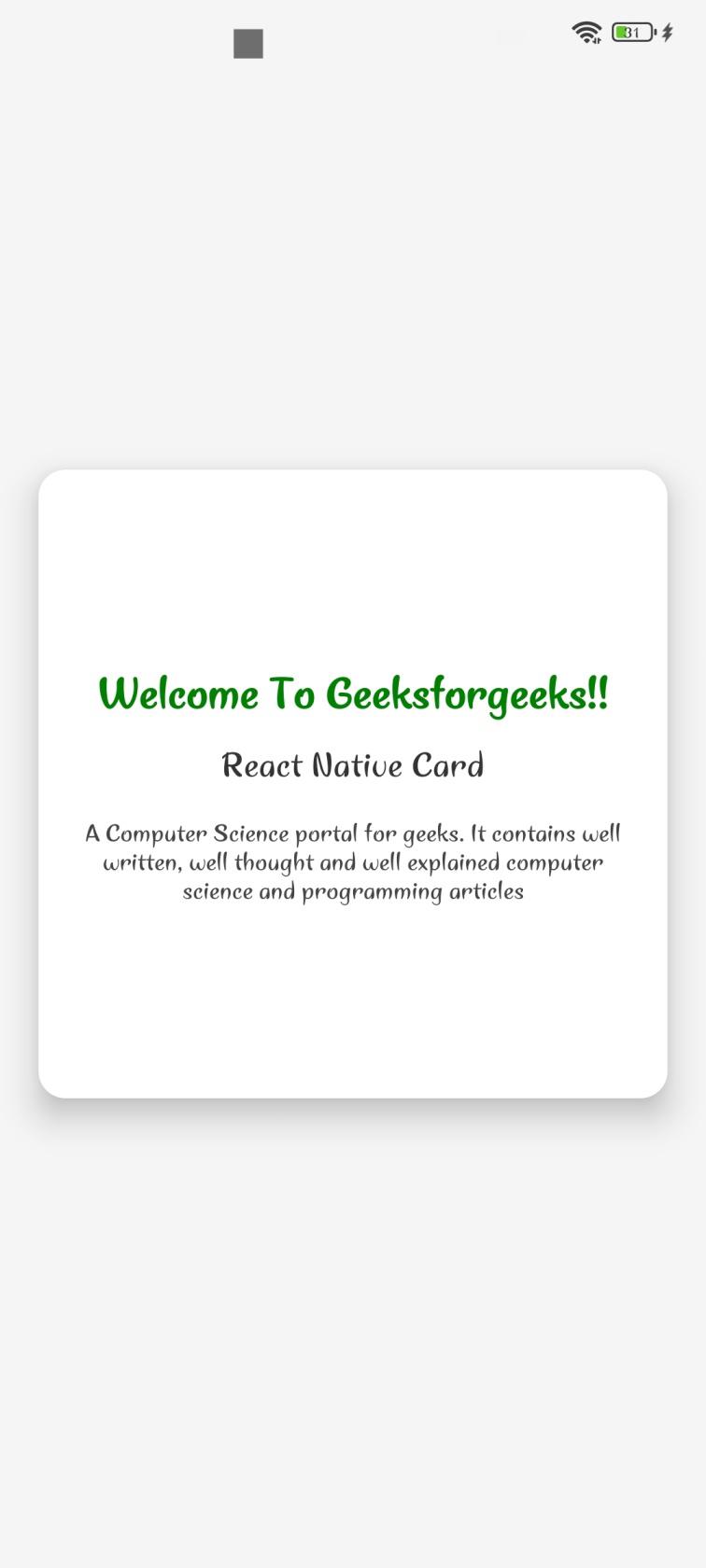
Example 2: Card with Box Shadow with Button in React Native
This example is similar to the previous example, only the difference is that we have added a button where we are using the onPress() function to show the card with a box shadow.
Javascript
import React, { useState } from 'react' ;
import { View, Text, StyleSheet, TouchableOpacity }
from 'react-native' ;
const App = () => {
const [showCard, setShowCard] = useState( false );
const handleButtonClick = () => {
setShowCard(!showCard);
};
return (
<View style={styles.container}>
<TouchableOpacity onPress={handleButtonClick}
style={styles.button}>
<Text style={styles.buttonText}>
Box Shadow
</Text>
</TouchableOpacity>
{showCard && (
<View style={styles.cardContainer}>
<Text style={styles.heading}>
Welcome To Geeksforgeeks!!
</Text>
<Text style={styles.paragraph}>
A Computer Science portal for geeks.
It contains well written, well thought
and well explained computer science and
programming articles
</Text>
</View>
)}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center' ,
justifyContent: 'center' ,
backgroundColor: '#F5F5F5' ,
},
button: {
backgroundColor: 'green' ,
paddingVertical: 12,
paddingHorizontal: 24,
borderRadius: 8,
marginBottom: 16,
},
buttonText: {
color: '#FFFFFF' ,
fontSize: 16,
fontWeight: 'bold' ,
},
cardContainer: {
backgroundColor: '#FFFFFF' ,
borderRadius: 8,
padding: 16,
shadowColor: '#000000' ,
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.3,
shadowRadius: 4,
elevation: 10,
width: 350,
height: 350,
},
heading: {
fontSize: 25,
fontWeight: 'bold' ,
marginBottom: 8,
color: 'green' ,
alignItems: 'center' ,
},
paragraph: {
fontSize: 17,
lineHeight: 24,
},
});
export default App;
|
Step to run:Â To run the react native application, open the Terminal and enter the command listed below.Â
npx expo start
npx react-native run-android
npx react-native run-ios
Output:
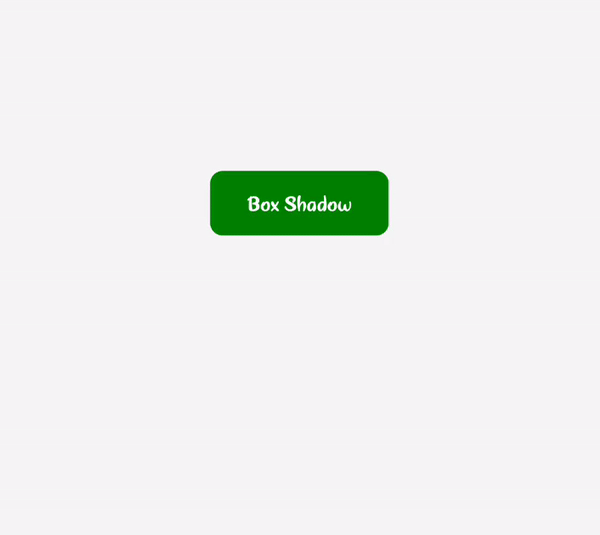
Share your thoughts in the comments
Please Login to comment...