How to optimize the performance of React app ?
Last Updated :
04 Dec, 2023
The operations involved in keeping the DOM updates are costly but react uses several techniques to minimize the no. of operations which leads to natively faster UI for many cases.
The following techniques can be used to speed up the application:
1. Use binding functions in constructors:
By adding an arrow function in a class, we add it as an object and not as the prototype property of the class. If we use the component multiple times, there will be various instances of these functions within each object of the component. The most reliable way to use functions is to bind them with the constructor.
2. Avoid inline style attributes:
The browser often invests a lot of time rendering, when styles are implied inline. Scripting and rendering take time because the browser has to plan all the React style rules to the CSS properties. Creating a separate style.js file and importing it into the component is a faster method.
Example: Creating a separate style.js file and importing in the component instead of using inline style attribute:
Javascript
import React from "react" ;
import styles from "./styles.css"
export default class StyleExample extends React.Component {
render() {
return (
<>
<h1>
GeeksforGeeks
</h1>
</>
);
}
}
|
CSS
h 1 {
color : green ;
margin : 50 ;
}
|
Output:
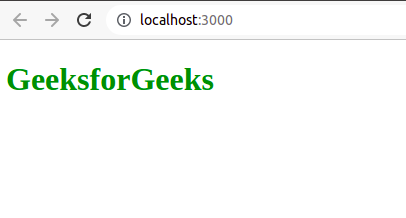
Using react fragments decreases the no. of additional tags and satisfies the necessity of having a single parent element in the component.
Example: Using react fragments
javascript
import React from "react" ;
const App = () => {
return (
<div>
<h1 style={{ color: "green" , margin: 50 }}>
GeeksforGeeks: react fragments as root element
</h1>
</div>
);
};
export default App;
|
Output:
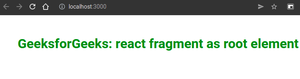
4. Avoid inline function in the render method:
If we use the inline function, the function will generate a new instance of the object in every render and there will be multiple instances of these functions which will lead to consuming more time in garbage collection. To optimize that we can define functions outside the render method and call them wherever required.
Example: Creating functions outside the render method
javascript
import React, { useState } from "react" ;
const App = () => {
const [isClicked, setIsclicked] = useState( false );
const handleClicked = () => {
setIsclicked( true );
console.log(`clicked ${isClicked}`);
};
return (
<div style={{ margin: 50 }}>
<h1 style={{ color: "green" }}>
GeeksforGeeks: function outside render example
</h1>
<button onClick={handleClicked}>Click me!</button>
</div>
);
};
export default App;
|
Output:
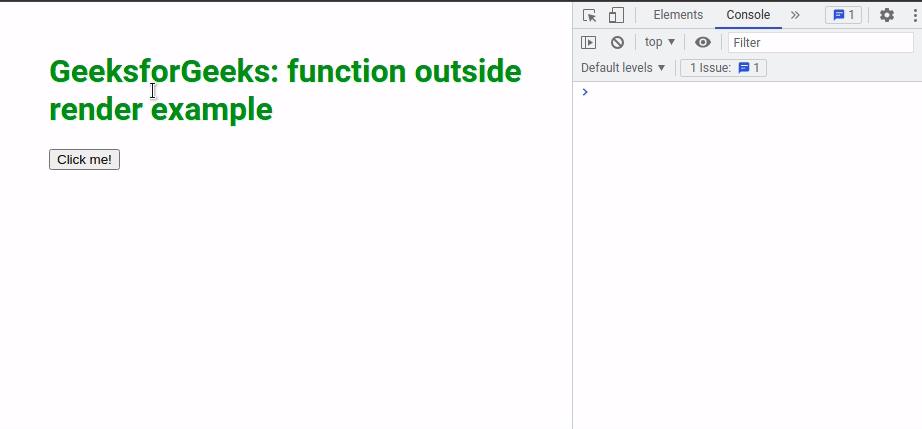
5. Avoid bundling all of the front end code in a single file:
By splitting the files into resource and on-demand code files we can reduce the time consumed in presenting bundled files to the browser transformers.
Share your thoughts in the comments
Please Login to comment...