How can you optimize the performance of React-Redux applications?
Last Updated :
17 Apr, 2024
Performance optimization in React-Redux involves improving the speed, efficiency, and responsiveness of applications by minimizing rendering times, reducing unnecessary re-renders, and optimizing data fetching and processing. By implementing optimization techniques, you can enhance the overall user experience and ensure that applications run smoothly even under heavy loads.
Below are the methods by which we can optimize the performance in a react-redux application.
Memoization
Memoization is a technique used to optimize expensive function calls by caching their results. In React-Redux applications, memoization can be implemented using libraries like reselect. Memoized selectors prevent unnecessary re-renders by memoizing the results of expensive computations.
Syntax:
import { createSelector } from 'reselect';
const firstSelector = state => state.first;
const secondSelector = state => state.second;
const myMemoizedSelector = createSelector(
firstSelector,
secondSelector,
(first, second) => {
// Compute derived data based on input selectors
return /* derived data */;
}
);
Selectors
Selectors are functions that compute derived data from the Redux store state. They efficiently extract specific pieces of state for components, reducing the amount of unnecessary rendering. Selectors are particularly useful in large-scale applications with complex state structures.
Syntax:
import { createSelector } from 'reselect';
const selectItems = state => state.items;
export const selectFilteredItems = createSelector(
[selectItems],
items => items.filter(item => item.completed)
);
Redux Toolkit is the official recommended way to write Redux logic. It simplifies the Redux workflow by providing utilities like createSlice for defining reducers, createAction for creating action creators, and createAsyncThunk for handling asynchronous actions. Using Redux Toolkit can streamline development and improve performance.
Syntax:
import { createSlice } from '@reduxjs/toolkit';
const itemsSlice = createSlice({
name: 'items',
initialState: {
items: [],
},
reducers: {
toggleCompleted: (state, action) => {
state.items = state.items.map(item =>
item.id === action.payload ? { ...item, completed: !item.completed } : item
);
},
},
});
export const { toggleCompleted } = itemsSlice.actions;
export default itemsSlice.reducer;
Code Splitting
Code splitting involves breaking down the application bundle into smaller chunks and loading them asynchronously. This technique reduces the initial load time of the application, as only the essential code is loaded initially. Lazy loading components and routes can significantly improve performance, especially in large applications.
Syntax:
const AsyncComponent = React.lazy(() => import('./AsyncComponent'));
Virtualized Lists
Virtualized lists optimize rendering performance by only rendering the items visible in the viewport. Libraries like react-virtualized or react-window efficiently handle large lists by rendering only the items currently in view, reducing the memory footprint and improving scrolling performance.
Syntax:
import { FixedSizeList as List } from 'react-window';
const Row = ({ index, style }) => (
<div style={style}>
Row {index}
</div>
);
<List
height={400}
itemCount={1000}
itemSize={35}
width={300}
>
{Row}
</List>
Server-side Rendering
Server-side rendering (SSR) pre-renders the React components on the server before sending them to the client. This approach improves perceived performance and SEO, as the initial HTML content is served immediately, while JavaScript bundles are loaded in the background. Libraries like Next.js make implementing SSR in React-Redux applications straightforward.
Syntax:
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/items');
const items = await res.json();
return {
props: { items },
};
}
export default IndexPage;
Steps to Create Application and Install Required Modules
Step 1: Create the react application using the following command.
npm create vite@latest my-app-redux
cd my-app-redux
Step 2: Install Redux, React-Redux and Redux Toolkit:
npm install redux react-redux @reduxjs/toolkit reselect react-virtualized
Updated Dependencies in package.json File:
"dependencies": {
"react": "^17.0.2",
"react-dom": "^17.0.2",
"redux": "^4.1.0",
"react-redux": "^7.2.5",
"@reduxjs/toolkit": "^1.6.2",
"reselect": "^4.1.3",
"react-virtualized": "^9.22.3",
}
Folder Structure:

Example: In this example, selectFilteredItems is a memoized selector that efficiently computes the filtered list of items based on the Redux store state. It only recomputes the filtered list when the items state changes, preventing unnecessary re-renders in connected components.
JavaScript
//main.jsx
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
JavaScript
// App.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { selectFilteredItems } from './selectors';
const App = () => {
const filteredItems = useSelector(selectFilteredItems);
const dispatch = useDispatch();
const handleToggle = id => {
dispatch({ type: 'TOGGLE_COMPLETED', payload: id });
};
return (
<div>
<h1>Todo List</h1>
<ul>
{filteredItems.map(item => (
<li
key={item.id}
style={{ textDecoration: item.completed ? 'line-through' : 'none' }}
onClick={() => handleToggle(item.id)}
>
{item.text}
</li>
))}
</ul>
</div>
);
};
export default App;
JavaScript
// store.js
import { createStore } from 'redux';
// Initial state
const initialState = {
items: [
{ id: 1, text: 'Buy groceries', completed: false },
{ id: 2, text: 'Do laundry', completed: true },
{ id: 3, text: 'Clean house', completed: false }
]
};
// Reducer
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'TOGGLE_COMPLETED':
return {
...state,
items: state.items.map(item =>
item.id === action.payload ?
{ ...item, completed: !item.completed } : item
)
};
default:
return state;
}
};
// Create store
const store = createStore(reducer);
export default store;
JavaScript
// selectors.js
import { createSelector } from 'reselect';
const selectItems = state => state.items;
export const selectFilteredItems = createSelector(
[selectItems],
items => items
);
To start the application run the following command.
npm run dev
Output:
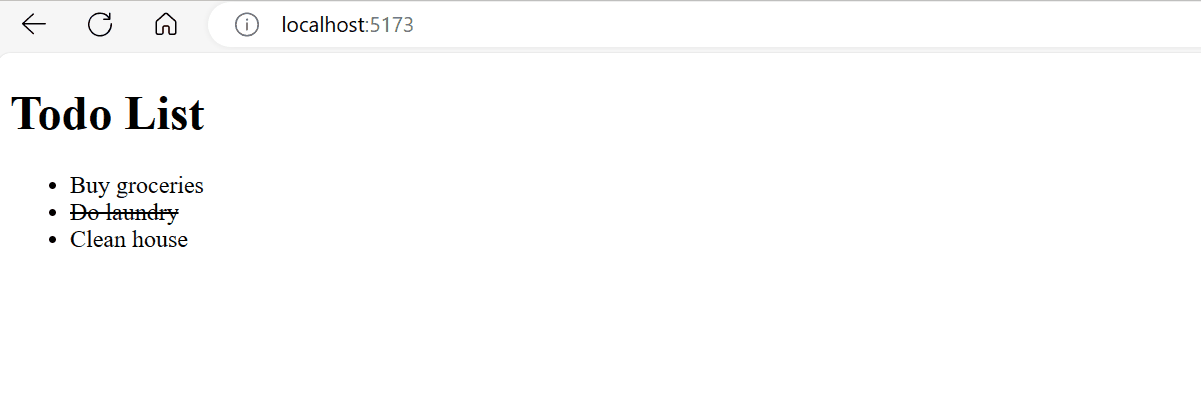
How can you optimize the performance of React-Redux applications
Share your thoughts in the comments
Please Login to comment...