How to Make Text Blink in ReactJS ?
Last Updated :
30 Oct, 2023
In this article, we are going to learn about making a text blink in React. Making text blink in React refers to the process of creating a visual effect where text repeatedly alternates between being visible and invisible, creating a flashing or blinking appearance, often achieved through CSS animations or state management within a React application
We will explore all the above methods along with their basic implementation with the help of examples.
Prerequisites:
- Introduction to React
- NPM or NPX
Steps to Create React Application:
Step 1: Create a React project:
npx create-react-app appname
Step 2: After creating your project folder i.e. appname, move to it using the following command:
cd appname
Step to run the application: Run the application using the following command from the root directory of the project.
npm start
Project Structure:
The project should look like this:
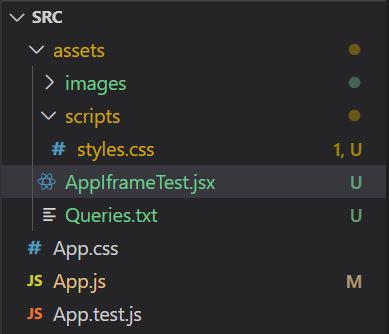
Approach 1: Using React dependency ‘react-blink-text’:
Using the ‘react-blink-text’ library in a React application to create text that blinks. You can simply import and use the ‘Blink’ component from the library, providing the text you want to blink as a prop.
Install Required Dependency
npm install react-blink-text --save
Syntax:
<Blink text='Geeks Premier League 2023' ></Blink>
Example : In this example, we are using the ‘react-blink-text’ library to create a blinking text effect with the text “Geeks Premier League 2023.” The text blinks inside a React component within a web page.
Javascript
import React from 'react' ;
import Blink from 'react-blink-text' ;
function App() {
return (
<div className= "App" >
<div>
<h2 style={{ color: 'green' }}>
GeeksforGeeks
</h2>
<h2>
How to make text blink in React
</h2>
<Blink text= "Geeks Premier League 2023" >
{ }
</Blink>
</div>
</div>
);
}
export default App;
|
Output:
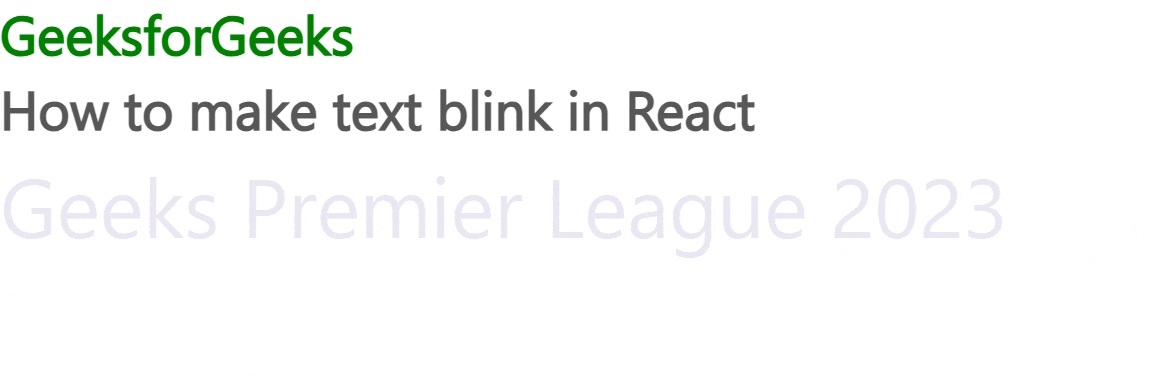
Approach 2: Using external CSS
In this approach, we are using external CSS to make text blink in a React app. It defines a CSS class named .blink with a keyframes animation controlling opacity, creating a blinking effect when applied to the text element.
Syntax:
.blink {
animation: blink-animation 1s infinite;
}
@keyframes blink-animation {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
Example : In this example, we will Blink our text using CSS animation.
Javascript
import React from "react" ;
import "./App.css" ;
function App() {
return (
<div className= "App" >
<div>
<h2 style={{ color: "green" }}>
GeeksforGeeks
</h2>
<h2>
How to make text blink in React
</h2>
<h3 className= "blink"
style={{ color: "green" }}>
Geeks Premier League 2023
</h3>
</div>
</div>
);
}
export default App;
|
CSS
. blink {
animation: blink-animation 1 s infinite;
}
@keyframes blink-animation {
0% {
opacity: 1 ;
}
50% {
opacity: 0 ;
}
100% {
opacity: 1 ;
}
}
|
Step: to run the application: Enter the following command to run the application.
npm start
Output:
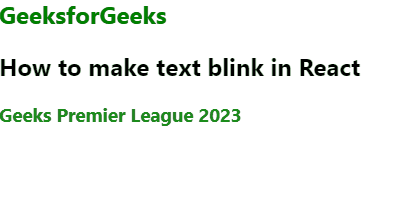
Share your thoughts in the comments
Please Login to comment...